3 回答
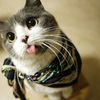
TA贡献1811条经验 获得超6个赞
这是另一种更简单的方法:
public class ReadTimes {
private Map<String, List<String>> timeLists = new HashMap();
public void read_file() {
try {
Scanner times = new Scanner(new File("times.csv"));
read_file(times);
} catch (Exception e) {
System.out.printf("Could not find file\n");
}
}
public void read_file(Scanner times) {
String label=null;
while (times.hasNextLine()) {
String time[] = times.nextLine().trim().split("[, ]+");
for (String timeString : time) {
if (timeString.startsWith("time")) {
label = timeString;
timeLists.put(label, new ArrayList());
} else if (label != null) {
timeLists.get(label).add(timeString);
}
}
}
// dump the map of arraylists for demonstration purposes...
for (Entry<String,List<String>> timeEntry : timeLists.entrySet()) {
System.out.println(timeEntry);
}
}
public static void main(String[] args) {
ReadTimes rt = new ReadTimes();
rt.read_file();
}
}
鉴于您的问题中显示的输入数据,将产生以下输出:
time1=[5:01, 3:21, 4:05, 1:52]
time2=[2:11, 6:35, 2:00, 5:00]
time3=[12:09, 11:35, 9:02]
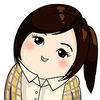
TA贡献1836条经验 获得超3个赞
由于您确定您只需要 3 个字符串数组,因此下面的代码使用“时间”作为分隔符拆分初始字符串,在开头删除"1, ", "2, ","3, "在,结尾处进行修剪和删除,最后在删除所有空格后将每个项目拆分为, 作为产生 3 个字符串数组的分隔符。
String times = "time1, 5:01,3:21,4:05,1:52, time2, 2:11,6:35,2:00,5:00, time3, 12:09,11:35, 9:02";
String[] splitted = times.split("time");
// exclude 0th item which is ""
for (int i = 1; i < splitted.length; i++) {
splitted[i] = splitted[i].trim();
int index = splitted[i].indexOf(" ");
if (splitted[i].endsWith(","))
splitted[i] = splitted[i].substring(index + 1, splitted[i].length() - 1);
else
splitted[i] = splitted[i].substring(index + 1);
splitted[i] = splitted[i].replaceAll(" ", "");
}
try { // just in case
String time1[] = splitted[1].split(",");
System.out.println(Arrays.toString(time1));
String time2[] = splitted[2].split(",");
System.out.println(Arrays.toString(time2));
String time3[] = splitted[3].split(",");
System.out.println(Arrays.toString(time3));
} catch (Exception e) {
e.printStackTrace();
}
打印 3 个字符串数组:
[5:01, 3:21, 4:05, 1:52]
[2:11, 6:35, 2:00, 5:00]
[12:09, 11:35, 9:02]
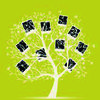
TA贡献1873条经验 获得超9个赞
这是一个单元测试,它可以满足您的需求。我不得不稍微格式化您的输入字符串。您应该能够以最少的更改为您完成这项工作。
import org.junit.Test;
import java.time.LocalDate;
import java.time.LocalTime;
import java.time.format.DateTimeFormatter;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* @author Dr. Parameter
*/
public class TimeParser {
private final DateTimeFormatter formatter = DateTimeFormatter.ofPattern("k:mm");
@Test
public void test(){
// Test input
String times = "time1, 5:01,3:21,4:05,1:52, time2, 2:11,6:35,2:00,5:00, time3, 12:09,11:35, 9:02";
// Set up Objects used to collect
String currentKey = "";
HashMap<String, List<LocalTime>> timeMap = new HashMap();
// Iterate though list
String[] lineBreaks = times.split("\n");
for(String line : lineBreaks){
String[] csvBreaks = line.split(",");
for(String csv : csvBreaks){
//try to parse a time and add it to the key set, add a new key if there is a failure
try {
timeMap.get(currentKey).add(LocalTime.parse(csv.trim(), formatter));
} catch (Exception ex){
currentKey = csv;
timeMap.put(currentKey, new ArrayList<>());
}
}
}
//Print result:
for(Map.Entry<String, List<LocalTime>> entry : timeMap.entrySet()){
System.out.println("times for key: " + entry.getKey());
for(LocalTime time : entry.getValue()){
System.out.println("\t" + time);
}
}
}
}
添加回答
举报