3 回答
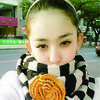
TA贡献1871条经验 获得超13个赞
在 Java 8+ 中,您可以使用Month枚举。
不幸的是,Month它parse不像大多数其他java.time类那样提供方法,但是,如果您查看该getDisplayName(TextStyle style, Locale locale)方法的实现,您会发现:
public String getDisplayName(TextStyle style, Locale locale) {
return new DateTimeFormatterBuilder()
.appendText(MONTH_OF_YEAR, style)
.toFormatter(locale)
.format(this);
}
所以为了解析我们可以创建我们自己的方法,使用相同类型的格式化程序,如下所示:
public static Month parseMonth(CharSequence text, TextStyle style, Locale locale) {
DateTimeFormatter fmt = new DateTimeFormatterBuilder()
.appendText(ChronoField.MONTH_OF_YEAR, style)
.toFormatter(locale);
return Month.from(fmt.parse(text));
}
例子
String s = Month.OCTOBER.getDisplayName(TextStyle.FULL, Locale.US);
System.out.println(s); // prints: October
Month month = parseMonth("October", TextStyle.FULL, Locale.US);
System.out.println(month); // prints: OCTOBER
System.out.println(month.getValue()); // prints: 10
在 Java <8 中,您可以使用SimpleDateFormat解析文本:
public static int parseMonth(String text) {
SimpleDateFormat fmt = new SimpleDateFormat("MMMM", Locale.US);
try {
Calendar cal = Calendar.getInstance();
cal.setTime(fmt.parse(text));
return cal.get(Calendar.MONTH) + 1;
} catch (ParseException e) {
throw new IllegalArgumentException("Invalid month: " + text);
}
}
例子
int month = parseMonth("October");
System.out.println(month); // prints: 10
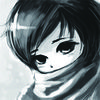
TA贡献1868条经验 获得超4个赞
顺便说一句,但如果您使用枚举,您还可以根据枚举的名称进行查找。
例如,一个枚举像
public enum MyMonth {January, February, March, April, May }
你可以这样做
MyMonth month = MyMonth.valueOf("February");
获取名为“二月”的月份的枚举。
要获取“月数”,您可以使用以下ordinal()方法:
MyMonth month = MyMonth.valueOf("February");
System.out.println( month.ordinal() );
由于 Java 枚举是从零开始的,因此二月打印“1”。如果您希望一月从一个开始,您必须记住添加一个。
使用整数可以方便地迭代您的所有月份(或任何其他枚举),但使用values()静态方法您不需要:
for( MyMonth m : MyMonth.values() )
System.out.println( m );
这些代码行将打印MyMonth枚举中定义的所有月份名称。
这是完整文档的链接。很多人不知道这一点,因为它隐藏在 JLS 的一个有点晦涩的部分中,并且没有在 API 文档中直接引用。
https://docs.oracle.com/javase/specs/jls/se7/html/jls-8.html#jls-8.9
In addition, if E is the name of an enum type,
then that type has the following implicitly declared
static methods:
/**
* Returns an array containing the constants of this enum
* type, in the order they're declared. This method may be
* used to iterate over the constants as follows:
*
* for(E c : E.values())
* System.out.println(c);
*
* @return an array containing the constants of this enum
* type, in the order they're declared
*/
public static E[] values();
/**
* Returns the enum constant of this type with the specified
* name.
* The string must match exactly an identifier used to declare
* an enum constant in this type. (Extraneous whitespace
* characters are not permitted.)
*
* @return the enum constant with the specified name
* @throws IllegalArgumentException if this enum type has no
* constant with the specified name
*/
public static E valueOf(String name);
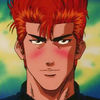
TA贡献1839条经验 获得超15个赞
Java 有一个内置程序enum可以在java.time包中为您处理这个问题。
你可以像这样使用它:
import java.time.Month;
public class Main {
public static void main(String[] args) {
// Use sample month of April
Month month = Month.APRIL;
// Get the month number
System.out.println("Month #: " + month.getValue());
}
}
添加回答
举报