3 回答
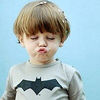
TA贡献1942条经验 获得超3个赞
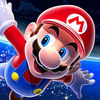
TA贡献1804条经验 获得超7个赞
如果你需要继承来重用,这个例子展示了我如何重用形状界面的宽度/高度,
package main
// compare to a c++ example: http://www.tutorialspoint.com/cplusplus/cpp_interfaces.htm
import (
"fmt"
)
// interface
type Shape interface {
Area() float64
GetWidth() float64
GetHeight() float64
SetWidth(float64)
SetHeight(float64)
}
// reusable part, only implement SetWidth and SetHeight method of the interface
// {
type WidthHeight struct {
width float64
height float64
}
func (this *WidthHeight) SetWidth(w float64) {
this.width = w
}
func (this *WidthHeight) SetHeight(h float64) {
this.height = h
}
func (this *WidthHeight) GetWidth() float64 {
return this.width
}
func (this *WidthHeight) GetHeight() float64 {
fmt.Println("in WidthHeight.GetHeight")
return this.height
}
// }
type Rectangle struct {
WidthHeight
}
func (this *Rectangle) Area() float64 {
return this.GetWidth() * this.GetHeight() / 2
}
// override
func (this *Rectangle) GetHeight() float64 {
fmt.Println("in Rectangle.GetHeight")
// in case you still needs the WidthHeight's GetHeight method
return this.WidthHeight.GetHeight()
}
func main() {
var r Rectangle
var i Shape = &r
i.SetWidth(4)
i.SetHeight(6)
fmt.Println(i)
fmt.Println("width: ",i.GetWidth())
fmt.Println("height: ",i.GetHeight())
fmt.Println("area: ",i.Area())
}
结果:
&{{4 6}}
width: 4
in Rectangle.GetHeight
in WidthHeight.GetHeight
height: 6
in Rectangle.GetHeight
in WidthHeight.GetHeight
area: 12
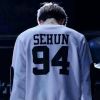
TA贡献1155条经验 获得超0个赞
我认为嵌入足够接近定义:
package main
import "net/url"
type address struct { *url.URL }
func newAddress(rawurl string) (address, error) {
p, e := url.Parse(rawurl)
if e != nil {
return address{}, e
}
return address{p}, nil
}
func main() {
a, e := newAddress("https://stackoverflow.com")
if e != nil {
panic(e)
}
{ // inherit
s := a.String()
println(s)
}
{ // fully qualified
s := a.URL.String()
println(s)
}
}
https://golang.org/ref/spec#Struct_types
- 3 回答
- 0 关注
- 144 浏览
添加回答
举报