3 回答
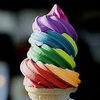
TA贡献1780条经验 获得超1个赞
你应该previous在循环中更新。
class Node {
constructor(data, next) {
this.data = data;
this.next = next;
}
}
class LinkedList {
constructor() {
this.head = null;
}
insertFirst(data) {
this.head = new Node(data, this.head);
}
size() {
let counter = 0,
node = this.head;
while (node) {
counter++;
node = node.next;
}
return counter;
}
toArray() {
let node = this.head;
const result = [];
while (node) {
result.push(node.data);
node = node.next;
}
return result;
}
removeEven() {
let previous = this.head;
let node = this.head.next;
if (this.isEven(previous.data)) {
console.log('outside loop, found one: ' + previous.data)
this.head = this.head.next;
}
while (node) {
if (this.isEven(node.data)) {
console.log('found ' + node.data);
previous.next = node.next;
} else {
previous = node;
}
node = node.next;
}
}
removeOdd() {
let previous = this.head;
let node = this.head.next;
if (!this.isEven(previous.data)) {
console.log('outside loop, found one: ' + previous.data)
this.head = this.head.next;
}
while (node) {
if (!this.isEven(node.data)) {
console.log('found ' + node.data);
previous.next = node.next;
} else {
previous = node;
}
node = node.next;
}
}
isEven(num) {
return num % 2 === 0 ? true : false;
}
}
const q = new LinkedList();
q.insertFirst(16)
q.insertFirst(3)
q.insertFirst(4)
q.insertFirst(7)
q.insertFirst(5)
q.insertFirst(2)
q.insertFirst(1)
q.insertFirst(15)
q.insertFirst(18)
q.removeOdd();
console.log(q.toArray());
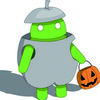
TA贡献1846条经验 获得超7个赞
您非常接近,您需要保留对上次更新值的引用
class Node {
constructor(data, next) {
this.data = data;
this.next = next;
}
}
class LinkedList {
constructor() {
this.head = null;
}
insertFirst(data) {
this.head = new Node(data, this.head);
}
size() {
let counter = 0,
node = this.head;
while (node) {
counter++;
node = node.next;
}
return counter;
}
toArray() {
let node = this.head;
const result = [];
while (node) {
result.push(node.data);
node = node.next;
}
return result;
}
removeEven() {
let current = this.head;
let final;
while (current.next) {
if (this.isEven(current.data)) {
current = current.next;
} else {
if (!final) {
final = current
this.head = final
} else {
final.next = current
final = current
}
current = current.next
}
}
if (this.isEven(current.data)) {
final.next = null
}
}
isEven(num) {
return num % 2 === 0 ? true : false;
}
}
const q = new LinkedList();
q.insertFirst(16)
q.insertFirst(3)
q.insertFirst(4)
q.insertFirst(7)
q.insertFirst(5)
q.insertFirst(2)
q.insertFirst(1)
q.insertFirst(15)
q.insertFirst(18)
q.removeEven();
console.log(q.toArray());
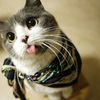
TA贡献1827条经验 获得超7个赞
class Node {
constructor(data, next) {
this.data = data;
this.next = next;
}
}
class LinkedList {
constructor() {
this.head = null;
}
removeNodesWithNumberType(type = "isEven") {
if (!this.head) return;
let previousNode = this.head;
let traversingNode = this.head;
while (traversingNode) {
if (this[type](traversingNode.data)) {
this.removeNode(previousNode, traversingNode);
} else {
previousNode = traversingNode;
}
traversingNode = traversingNode.next;
}
}
removeNode(previousNode, node) {
if (this.isFirstNode(node)) this.head = node.next;
previousNode.next = node.next;
}
isFirstNode(node) {
return this.head === node;
}
isEven(num) {
return num % 2 === 0;
}
isOdd(num) {
return !this.isEven(num);
}
insertFirst(data) {
this.head = new Node(data, this.head);
}
size() {
let counter = 0,
node = this.head;
while (node) {
counter++;
node = node.next;
}
return counter;
}
toArray() {
let node = this.head;
const result = [];
while (node) {
result.push(node.data);
node = node.next;
}
return result;
}
clear() {
this.head = null;
}
}
const q = new LinkedList();
// Test Case:1 Empty List
removeAndFormateOutput("isEven");
// Test Case:2 Single Node
q.insertFirst(16);
removeAndFormateOutput("isEven");
q.insertFirst(13);
removeAndFormateOutput("isOdd");
// Test Case:3 Two Consecutive Even
q.insertFirst(16);
q.insertFirst(18);
removeAndFormateOutput("isEven");
// Test Case:3 Two Consecutive odd
q.insertFirst(11);
q.insertFirst(13);
removeAndFormateOutput("isOdd");
// Test Case:4 Random List
q.insertFirst(3);
q.insertFirst(4);
q.insertFirst(7);
q.insertFirst(5);
q.insertFirst(2);
q.insertFirst(1);
q.insertFirst(15);
q.insertFirst(18);
q.insertFirst(20);
removeAndFormateOutput("isEven");
function removeAndFormateOutput(type) {
console.log(`Remove if ${type} \n Before : ${q.toArray()}`);
q.removeNodesWithNumberType(type);
console.log(` After : ${q.toArray()}\n`);
q.clear();
}
添加回答
举报