2 回答
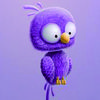
TA贡献1796条经验 获得超4个赞
Comparator<Coordinate> c = Comparator.comparingInt(Coordinate::getY)
.thenComparingInt(Coordinate::getX);
您可以通过thenComparing和构建复合比较器thenComparingX。
var list = List.of(
new Coordinate(6, 4),
new Coordinate(2, 5),
new Coordinate(5, 4)
);
list.sort(c);
System.out.println(list);
片段打印
[{y=4, x=5}, {y=4, x=6}, {y=5, x=2}]
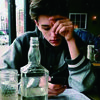
TA贡献1828条经验 获得超6个赞
使用比较器
import java.util.ArrayList;
import java.util.Comparator;
class Coordinate {
private int x, y;
public Coordinate(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public void setX(int x) {
this.x = x;
}
public int getY() {
return y;
}
public void setY(int y) {
this.y = y;
}
public String toString() {
return "x = " + x + " y = " + y;
}
}
public class Temp {
public static void main(String[] args) {
ArrayList<Coordinate> A = new ArrayList<>();
A.add(new Coordinate(1, 2));
A.add(new Coordinate(2, 1));
A.add(new Coordinate(3, 2));
A.sort(new Comparator<Coordinate>() {
@Override
public int compare(Coordinate o1, Coordinate o2) {
if (o1.getY() < o2.getY()) {
return -1;
} else if (o1.getY() > o2.getY()) {
return 1;
} else {
if (o1.getX() < o2.getX()) {
return -1;
} else if (o1.getX() > o2.getX()) {
return 1;
}
return 0;
}
}
});
System.out.println(A.toString());
}
}
使用可比较的接口
import java.util.ArrayList;
class Coordinate implements Comparable<Coordinate> { # Notice implementing Comparable interface
private int x, y;
public Coordinate(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public void setX(int x) {
this.x = x;
}
public int getY() {
return y;
}
public void setY(int y) {
this.y = y;
}
@Override
public int compareTo(Coordinate o) { # implementing the abstract method of Comparable interface
if (y < o.y) {
return -1;
} else if (y > o.y) {
return 1;
} else {
if (x < o.x) {
return -1;
} else if (x > o.x) {
return 1;
}
return 0;
}
}
public String toString() {
return "x = " + x + " y = " + y;
}
}
public class Temp {
public static void main(String[] args) {
ArrayList<Coordinate> A = new ArrayList<>();
A.add(new Coordinate(1, 2));
A.add(new Coordinate(2, 1));
A.add(new Coordinate(3, 2));
A.sort(null);
System.out.println(A.toString());
}
}
输出
[x = 2 y = 1, x = 1 y = 2, x = 3 y = 2]
添加回答
举报