编写一个方法来查找给定元素在堆栈中的位置,从堆栈顶部开始计数。更准确地说,如果元素出现在顶部,该方法应该返回 0,如果它上面有另一个元素,则返回 1,依此类推。如果元素出现多次,则应返回最上面的位置。如果元素根本没有出现,则必须返回 -1。要求您以两种不同的方式编写此方法;一种方法是在 ArrayStack 类内部实现它,另一种方法是在单独的类中外部实现它。重要提示:最后堆栈应返回到原始状态(即不应删除任何元素且不应更改元素的顺序)。这是外部类public class Stack{public static int searchstack(ArrayStack z, int n) { ArrayStack temp = new ArrayStack(z.size()); int c = 0; boolean flag = false; while (!z.isEmpty()) { if (z.top() == n) { flag = true; return c; } if (z.top() != n) { temp.push(z.pop()); c++; flag = false; } } if (flag == false) { c = -1; } while (!temp.isEmpty() && !z.isFull()) { z.push(temp.pop()); } return c; } public static void main(String[] args) { ArrayStack z = new ArrayStack(4); z.push(3); // first element z.push(7);// 2nd z.push(8);// 3rd z.push(1);// 4th z.printStack(); int n = 3; System.out.println("Searching externally for" + " " + n + " " + searchstack(z, n)); System.out.println("Searching internally for" +" "+n+" "+ z.searchfor(n)+" "); //THE ERROR IS HERE} }这是 ArrayClasspublic class ArrayStack { private int[] theStack; private int maxSize; private int top;public ArrayStack(int s) { maxSize = s; theStack = new int[maxSize]; top = -1; }public void push(int elem) { top++; theStack[top] = elem; }public int pop() { int result = theStack[top]; top--; return result; }public int top() { return theStack[top]; }public boolean isFull() { return (top == (maxSize - 1)); }public boolean isEmpty() { return (top == -1); }Stack类出现的错误是在调用Arraystack类中实现的searchfor方法的最后一行,error表示Arraystack中没有实现名为searchfor()的方法;虽然我确实实施了它。似乎是问题所在?
1 回答
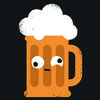
ITMISS
TA贡献1871条经验 获得超8个赞
您的 searchStack() 实现中有一个错误。如果您找到了您正在寻找的元素,并且它不是最顶层的元素,那么您就会丢失元素。
如何修复您的 searchStack() 方法:
继续弹出 z 直到你有一个空的 ArrayStack。这样做时,将该值添加到队列中。
创建 valIndex 并将其分配给 -1。
然后遍历队列并从中删除项目并将它们添加到 z。这样做时,检查所需值的最后一次出现并将其保存在 valIndex 中。
如果 valIndex 等于 -1,则返回它。否则,使用以下等式将其转换为正确的索引并返回:
valIndex = (z.size - 1) - valIndex
添加回答
举报
0/150
提交
取消