2 回答
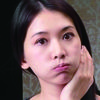
TA贡献1784条经验 获得超8个赞
//Begin by sorting your list by y values using List.sort()
polyMatList.sort( (pnt_a, pnt_b) => pnt_b.y - pnt_a.y ); // points 0 & 1 will by definition be your top points and points 2, 3 will be definition be your bottom points.
// now your top 2 points may be out of order since we only sorted by y in the previous step
Point tempPoint;
if(polyMatList[0].x > polyMatList[1].x)
{
tempPoint = polyMatList[0];
polyMatList[0] = polyMatList[1];
polyMatList[1] = tempPoint ;
}
// same goes for your bottom two points
if(polyMatList[2].x > polyMatList[3].x)
{
tempPoint = polyMatList[2];
polyMatList[2] = polyMatList[3];
polyMatList[3] = tempPoint ;
}
//now your list will be ordered tl, tr, bl, br
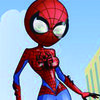
TA贡献1807条经验 获得超9个赞
这是我根据本教程实现的(尽管我确信我可以做一些更优雅的事情)。
polyMat.convertTo(orientationMat, CvType.CV_32S); //conver MatOfPoint2f back to MatofPoint so it can be drawn
//sort by y val
polyMatList = polyMatList.OrderBy(p => p.x).ToList();
List<Point> leftmostPts = new List<Point>();
leftmostPts.Add(polyMatList[0]);
leftmostPts.Add(polyMatList[1]);
List<Point> rightmostPts = new List<Point>();
rightmostPts.Add(polyMatList[2]);
rightmostPts.Add(polyMatList[3]);
//we now have a top left point
leftmostPts = leftmostPts.OrderBy(p => p.y).ToList();
//calculate distance from top left to rightmost 2 points:
double dX0 = rightmostPts[0].x - leftmostPts[0].x;
double dY0 = rightmostPts[0].y - leftmostPts[0].y;
double d0 = Math.Sqrt(dX0 * dX0 + dY0 * dY0);
double dX1 = rightmostPts[1].x - leftmostPts[0].x;
double dY1 = rightmostPts[1].y - leftmostPts[0].y;
double d1 = Math.Sqrt(dX1 * dX1 + dY1 * dY1);
List<Point> orderedPolyMat = new List<Point>();
orderedPolyMat.Add(leftmostPts[0]);
if (d0 > d1){ //greatest distance between right two points will be bottom right
orderedPolyMat.Add(rightmostPts[1]);
orderedPolyMat.Add(rightmostPts[0]);
} else {
orderedPolyMat.Add(rightmostPts[0]);
orderedPolyMat.Add(rightmostPts[1]);
}
orderedPolyMat.Add(leftmostPts[1]);
添加回答
举报