3 回答
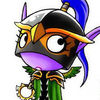
TA贡献1865条经验 获得超7个赞
您可以使用带有抽象基类的泛型来获得您需要的位置:
public abstract class Base<TModel, TService> where TService: IService
{
public TModel Model { get; set; }
private TService Service { get; set; }
protected bool Create()
{
try
{
Model = Service.Create(Model);
return true;
}
catch(Exception ex)
{
Console.WriteLine(ex.Message);
return false;
}
}
}
您在此处唯一需要更改的是向您的“服务”类型添加一个支持抽象,该类型具有对Create(). 然后,您必须将泛型限制为该抽象(在我上面的示例中,抽象是IService)。你的抽象看起来像这样:
public interface IService
{
T Create(T input);
}
实现这个抽象基类后,你的实现就像这样:
public class Example1 : Base<Object1, Service1>
{
//Code specific to this implementation
}
public class Example2 : Base<Object2, Service2>
{
//Code specific to this implementation
}
您永远不会解决的一件事是您的基地将如何实际获取其内容。您可能必须通过构造函数传递它们。我没有在这里提供,因为您也没有,但请记住,您至少需要将服务传递到基础中,以便它可以Create()从传递的服务中调用。
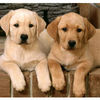
TA贡献1847条经验 获得超7个赞
如果模型和对象是同一个类,您的示例将起作用。如果 Object1 和 Object2 是不同的类,则可能需要进行一些调整,否则您可能无法实现。
如果它们是同一个类,您可以将 Object 变量设置为 protected 并在每个派生类的构造函数中以不同的方式声明它。
如果它们不是同一个类而是相似的,则 Object1、Object2 等类都可以从一个 Object 类继承,并且上面可以做同样的事情,但是如果 Object1 和 Object2 非常不同,这将没有用。
如果我明白你想要做什么,也许这会有所帮助:
public class Base
{
public Object model;
protected Service service;
protected bool Create()
{
try
{
Model = Service.Create(Model);
return true;
}
catch(Exception ex)
{
Console.WriteLine(ex.Message);
return false;
}
}
}
public class Example1 : Base
{
public Example1() //Assign specifics in constructor
{
model = model1;
service = service1;
}
}
public class Example2 : Base
{
public Example2() //Assign specifics in constructor
{
model = model2;
service = service2;
}
}
如果这没有帮助,那么也许您正在寻找的是抽象类/方法,但我不确定。
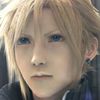
TA贡献1866条经验 获得超5个赞
interface IModel { }
interface IService<out TModel>
{
TModel Create(IModel model);
}
interface IModelService<TModel, TService> where TModel : IModel where TService : IService<TModel>
{
TModel Model { get; set; }
TService Service { get; set; }
}
class ExampleModel : IModel { }
class ExampleService : IService<ExampleModel>
{
public ExampleModel Create(TModel model)
{
return new ExampleModel();
}
}
class Example : ExampleBase<ExampleModel, ExampleService>
{
}
abstract class ExampleBase<TModel, TService> : IModelService<TModel, TService> where TModel : IModel where TService : IService<TModel>
{
public TModel Model { get; set; }
public TService Service { get; set; }
protected bool Create()
{
//do what you must
}
}
具有泛型类型参数和用于定义和约束的少数接口的抽象类
- 3 回答
- 0 关注
- 165 浏览
添加回答
举报