3 回答
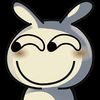
TA贡献1817条经验 获得超14个赞
您可以使用与其构造函数1的比较:
const int8 = new Uint8Array([0,1,2,3]);
console.log(int8.constructor === Uint8Array);
// so you can do
function checkTypedArrayType(someTypedArray) {
const typedArrayTypes = [
Int8Array,
Uint8Array,
Uint8ClampedArray,
Int16Array,
Uint16Array,
Int32Array,
Uint32Array,
Float32Array,
Float64Array,
BigInt64Array,
BigUint64Array
];
const checked = typedArrayTypes.filter(ta => someTypedArray.constructor === ta);
return checked.length && checked[0].name || null;
}
console.log(checkTypedArrayType(int8));
// but this can be hugely simplified
function checkTypedArrayType2(someTypedArray) {
return someTypedArray &&
someTypedArray.constructor &&
someTypedArray.constructor.name ||
null;
}
console.log(checkTypedArrayType2(int8));
// which can actually be generalized to
const whatsMyType = someObject =>
someObject &&
someObject.constructor &&
someObject.constructor.name &&
someObject.constructor.name ||
null;
console.log(whatsMyType(int8));
console.log(whatsMyType([1,2,3,4]));
console.log(whatsMyType("hello!"))
console.log(whatsMyType(/[a-z]/i))
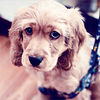
TA贡献1883条经验 获得超3个赞
对于 TypedArrays,实际上有一个等效于Array.isArray的,可以在ArrayBuffer构造函数上访问:
ArrayBuffer.isView.
但是请注意,它也会为DataView对象返回 true ,因此如果您真的想知道一个对象是否为 TypedArray,您可以简单地执行以下操作:
function isTypedArray( arr ) {
return ArrayBuffer.isView( arr ) && !(arr instanceof DataView);
}
function test( val ) {
console.log( isTypedArray( val ) );
}
test( false ); // false
test( null ); // false
test( [] ); // false
test( new ArrayBuffer( 12 ) ); // false
test( new DataView( new ArrayBuffer( 12 ) ) ); // false
test( new Uint8Array( 12 ) ); // true;
test( new BigInt64Array( 12 ) ); // true;
但这不会为您提供该 TypedArray 的特定类型。
TypedArray 的原型公开了一个BYTES_PER_ELEMENT属性,但仅此而已......
构造函数上有一个可以访问的.name属性,它本身可以通过.constructor每个实例的属性访问,但可以设置,IE 不支持。但是,如果这对您来说不是问题,那么只需:
function getType( arr ) {
return isTypedArray( arr ) && arr.constructor.name;
}
function isTypedArray( arr ) {
return ArrayBuffer.isView( arr ) && !(arr instanceof DataView);
}
function test( val ) {
console.log( getType( val ) );
}
test( [] ); // false
test( new ArrayBuffer( 12 ) ); // false
test( new DataView( new ArrayBuffer( 12 ) ) ); // false
test( new Uint8Array( 12 ) ); // "Uint8Array";
test( new BigInt64Array( 12 ) ); // "BigInt64Array";
如果您需要支持 IE,instanceof也可以选择独立测试所有可能的 TypedArrays 构造函数:
const typedArrays = [
'Int8',
'Uint8',
'Uint8Clamped',
'Int16',
'Uint16',
'Int32',
'Uint32',
'Float32',
'Float64',
'BigInt64',
'BigUint64'
].map( (pre) => pre + 'Array' );
function getType( arr ) {
return isTypedArray( arr ) &&
typedArrays.find( (type) => arr instanceof window[ type ] );
}
function isTypedArray( arr ) {
return ArrayBuffer.isView( arr ) && !(arr instanceof DataView);
}
function test( val ) {
console.log( getType( val ) );
}
test( [] ); // false
test( new Uint8Array( 12 ) ); // Uint8Array
test( new Int16Array( 12 ) ); // Int16Array
test( new Float32Array( 12 ) ); // Float32Array
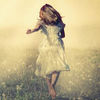
TA贡献1712条经验 获得超3个赞
另一种方法可能是,您可以使用BYTES_PER_ELEMENT类型化数组的属性
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/TypedArray/BYTES_PER_ELEMENT
Array.isTypedArray = function(inArray) {
if (inArray) {
const prototype = Object.getPrototypeOf(inArray);
return prototype ? prototype.hasOwnProperty("BYTES_PER_ELEMENT") : false;
}
return false;
};
正如其他答案中提到的,要获得实际类型,您可以使用constructor.name. 重用上面的isTypedArray函数,你可以这样写。
function getType(obj){
return Array.isTypedArray(obj) ? obj.constructor.name: (typeof obj)
}
示例代码和 console.logs
Array.isTypedArray = function(inArray){
if(inArray){
const prototype = Object.getPrototypeOf(inArray);
return prototype ? prototype.hasOwnProperty("BYTES_PER_ELEMENT") : false;
}
return false;
}
console.log(" 'Iam' a typed array 👉 ", Array.isTypedArray([1,2,3]));
console.log("[1,2,3] is typed array 👉 ", Array.isTypedArray([1,2,3]));
console.log("new Int8Array([1,2,3]) is typed array 👉 ", Array.isTypedArray(new Int8Array([1,2,3])));
console.log("new BigUint64Array() is typed array 👉 ", Array.isTypedArray(new BigUint64Array()));
console.log("new Uint8ClampedArray([1,2,3]) is typed array 👉 ", Array.isTypedArray(new Uint8ClampedArray([1,2,3])));
console.log("new Float32Array([1,2,3]) is typed array 👉 ", Array.isTypedArray(new Float32Array([1,2,3])));
console.log("new BigUint64Array() is typed array 👉 ", Array.isTypedArray(new BigUint64Array()));
console.log("new Set() is typed array 👉 ", Array.isTypedArray(new Set()));
console.log("null is typed array 👉 ", Array.isTypedArray(null));
console.log("undedined is typed array 👉 ", Array.isTypedArray(undefined));
console.log("{} is typed array 👉 ",Array.isTypedArray({}));
function getType(obj){
return Array.isTypedArray(obj) ? obj.constructor.name: (typeof obj)
}
console.log("Type of Array 👉 ", getType(new Array()));
console.log("Type of Int8Array 👉 ", getType(new Int8Array()));
console.log("Type of Uint8Array 👉 ",getType( new Uint8Array()));
console.log("Type of Uint8ClampedArray 👉 ",getType( new Uint8ClampedArray()));
console.log("Type of Int16Array 👉 ", getType(new Int16Array()));
console.log("Type of Float32Array 👉 ", getType(new Float32Array()));
console.log("Type of BigInt64Array 👉 ", getType(new BigInt64Array()));
console.log("Type of BigUint64Array 👉 ", getType(new BigUint64Array()));
添加回答
举报