3 回答
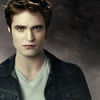
TA贡献1848条经验 获得超6个赞
只是为了详细说明我后面的评论,这里有两个示例如何上传到 Device Farm 的 SDK 在 java 中返回的预签名 URL。
下面是一个将文件上传到 Device Farm s3 预签名 URL 的示例:
package com.jmp.stackoveflow;
import java.io.File;
import java.io.IOException;
import java.io.OutputStreamWriter;
import java.net.HttpURLConnection;
import com.amazonaws.ClientConfiguration;
import com.amazonaws.auth.AWSSessionCredentials;
import com.amazonaws.auth.STSAssumeRoleSessionCredentialsProvider;
import com.amazonaws.services.devicefarm.*;
import com.amazonaws.services.devicefarm.model.CreateUploadRequest;
import com.amazonaws.services.devicefarm.model.Upload;
import org.apache.commons.lang3.RandomStringUtils;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpPut;
import org.apache.http.entity.FileEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
public class App {
public static void main(String[] args) {
String PROJECT_ARN = "arn:aws:devicefarm:us-west-2:111122223333:project:ffb3d9f2-3dd6-4ab8-93fd-bbb6be67b29b";
String ROLE_ARN = "arn:aws:iam::111122223333:role/DeviceFarm_FULL_ACCESS";
System.out.println("Creating credentials object");
// gettting credentials
STSAssumeRoleSessionCredentialsProvider sts = new STSAssumeRoleSessionCredentialsProvider.Builder(ROLE_ARN,
RandomStringUtils.randomAlphanumeric(8)).build();
AWSSessionCredentials creds = sts.getCredentials();
ClientConfiguration clientConfiguration = new ClientConfiguration()
.withUserAgent("AWS Device Farm - stackoverflow example");
AWSDeviceFarmClient api = new AWSDeviceFarmClient(creds, clientConfiguration);
api.setServiceNameIntern("devicefarm");
System.out.println("Creating upload object");
File app_debug_apk = new File(
"PATH_TO_YOUR_FILE_HERE");
FileEntity fileEntity = new FileEntity(app_debug_apk);
CreateUploadRequest appUploadRequest = new CreateUploadRequest().withName(app_debug_apk.getName())
.withProjectArn(PROJECT_ARN).withContentType("application/octet-stream").withType("ANDROID_APP");
Upload upload = api.createUpload(appUploadRequest).getUpload();
// Create the connection and use it to upload the new object using the
// pre-signed URL.
CloseableHttpClient httpClient = HttpClients.createSystem();
HttpPut httpPut = new HttpPut(upload.getUrl());
httpPut.setHeader("Content-Type", upload.getContentType());
httpPut.setEntity(fileEntity);
try {
HttpResponse response = httpClient.execute(httpPut);
System.out.println("Response: "+ response.getStatusLine().getStatusCode());
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
输出
Creating credentials object
Creating upload object
Response: 200
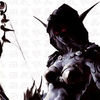
TA贡献2041条经验 获得超4个赞
这是一个有点老的问题。万一其他人发现了这个。这是我如何解决小于 5mb 的文件的问题。对于超过 5mb 的文件,建议使用分段上传。注意:使用 Java 的“尝试资源”很方便。Try Catch 使这成为一个笨拙的操作,但它确保在方法中以最少的代码量关闭资源。
/**
* Serial upload of an array of media files to S3 using a presignedUrl.
*/
public void serialPutMedia(ArrayList<String> signedUrls) {
long getTime = System.currentTimeMillis();
LOGGER.debug("serialPutMedia called");
String toDiskDir = DirectoryMgr.getMediaPath('M');
try {
HttpURLConnection connection;
for (int i = 0; i < signedUrls.size(); i++) {
URL url = new URL(signedUrls.get(i));
connection = (HttpURLConnection) url.openConnection();
connection.setDoOutput(true);
connection.setRequestMethod("PUT");
localURL = toDiskDir + "/" + fileNames.get(i);
try (BufferedInputStream bin = new BufferedInputStream(new FileInputStream(new File(localURL)));
ObjectOutputStream out = new ObjectOutputStream(new BufferedOutputStream(connection.getOutputStream())))
{
LOGGER.debug("S3put request built ... sending to s3...");
byte[] readBuffArr = new byte[4096];
int readBytes = 0;
while ((readBytes = bin.read(readBuffArr)) >= 0) {
out.write(readBuffArr, 0, readBytes);
}
connection.getResponseCode();
LOGGER.debug("response code: {}", connection.getResponseCode());
} catch (FileNotFoundException e) {
LOGGER.warn("\tFile Not Found exception");
LOGGER.warn(e.getMessage());
e.printStackTrace();
}
}
} catch (MalformedURLException e) {
LOGGER.warn(e.getMessage());
e.printStackTrace();
} catch (IOException e) {
LOGGER.warn(e.getMessage());
e.printStackTrace();
}
getTime = (System.currentTimeMillis() - getTime);
System.out.print("Total get time in syncCloudMediaAction: {" + getTime + "} milliseconds, numElement: {" + signedUrls.size() + "}");
}
添加回答
举报