3 回答
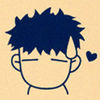
TA贡献1796条经验 获得超4个赞
你可以创建一个interface DeviceValueExtractor看起来像这样的:
@FunctionalInterface
public interface DeviceValueExtractor {
Object extractValue(Device device);
}
现在将您的方法重写为:
public void filterByType(Device[] devices, DeviceValueExtractor extractor, Object expect) {
if (devices == null) {
return;
}
for (int i = 0; i < devices.length; i++) {
if (devices[i] == null) {
continue;
}
Object actual = extractor.extractValue(devices[i]);
if (actual == null && expect== null) {
continue;
} else if (actual == null) {
devices[i] = null;
continue;
}
if (!Objects.equals(actual, expect)) {
devices[i] = null;
}
}
}
用法:
filterByType(devices, Device::getType, "Hello");
注意:我使用是Object因为要求没有泛型 - 因为调用的唯一方法是equals这实际上没什么大不了的。
但是,对于更多类型安全性,您可以引入泛型(并取消DeviceValueExtractor:
public static <T> void filterByType(Device[] devices, Function<Device, T> extractor, T expect) {
if (devices == null) {
return;
}
for (int i = 0; i < devices.length; i++) {
if (devices[i] == null) {
continue;
}
Object actual = extractor.apply(devices[i]);
if (actual == null && expect== null) {
continue;
} else if (actual == null) {
devices[i] = null;
continue;
}
if (!Objects.equals(actual, expect)) {
devices[i] = null;
}
}
}
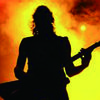
TA贡献1111条经验 获得超0个赞
也许一些 Java 8 魔法会在这里有所帮助:
public void filtrateByType(Device[] devices, String type) {
filtrateBy(devices, Device::getType, type);
}
public void filtrateBy(Device[] devices, Function<? super Device, String> attributeGetter, String attribute) {
if (devices == null) {
return;
}
for (int i = 0; i < devices.length; i++) {
if (devices[i] == null) {
continue;
}
if (attributeGetter.apply(devices[i]) == null && attribute == null) {
continue;
} else if (attributeGetter.apply(devices[i]) == null) {
devices[i] = null;
continue;
}
if (!attributeGetter.apply(devices[i]).equals(attribute)) {
devices[i] = null;
}
}
}
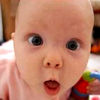
TA贡献1808条经验 获得超4个赞
这是更简单的版本。您可以使用原始类型,但这会更容易出错。
public static <T> void filtrateByType(T[] objects, Function<T, String> function, String type) {
if (objects == null || type == null)
return;
for (int i = 0; i < objects.length; i++) {
if (objects[i] == null) continue;
String match = function.apply(objects[i]);
if (match == null || !match.equals(type))
objects[i] = null;
}
}
但是,我怀疑您真正想要的是使用 Stream API
Device[] filtered = Stream.of(devices)
.filter(d -> Objects.equals(d.getType(), type))
.toArray(Device[]::new);
添加回答
举报