2 回答
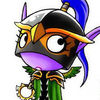
TA贡献1865条经验 获得超7个赞
此代码说明了您想要的一切。注意 hashCode、equals 和 compareTo 方法是如何被覆盖的。
package com.pkr.test;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Date;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class User implements Comparable<User> {
public static SimpleDateFormat sdf = new SimpleDateFormat("dd-MM-yyyy");
private long userId;
private String userName;
private Date createdDate;
public User(long userId, String userName, Date createdDate) {
this.userId = userId;
this.userName = userName;
this.createdDate = createdDate;
}
public long getUserId() {
return userId;
}
public void setUserId(long userId) {
this.userId = userId;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public Date getCreatedDate() {
return createdDate;
}
public void setCreatedDate(Date createdDate) {
this.createdDate = createdDate;
}
public String toString() {
return String.format("userId: %s, userName: %s, createdDate: %s",
new Object[] { userId, userName, sdf.format(createdDate) });
}
public boolean equals(Object o) {
if (o instanceof User) {
return ((User) o).getUserId() == this.getUserId();
}
return false;
}
public int hashCode() {
return new Long(userId).hashCode();
}
@Override
public int compareTo(User o) {
return this.getCreatedDate().compareTo(o.getCreatedDate());
}
public static void main(String args[]) throws Exception {
Set<User> userSet = new HashSet<User>();
userSet.add(new User(1, "Pushpesh", sdf.parse("16-02-2018")));
userSet.add(new User(2, "Vikrant", sdf.parse("12-02-2018")));
userSet.add(new User(3, "Abhay", sdf.parse("11-02-2018")));
userSet.add(new User(4, "Komal", sdf.parse("18-02-2018")));
userSet.stream().forEach(System.out::println);
// this will remove user with id 2 no matter what his name and
// createdDate are
userSet.remove(new User(2, "Vikrant", sdf.parse("12-02-2018")));
System.out.println("After removing userId 2");
userSet.stream().forEach(System.out::println);
System.out.println();
List<User> userList = new ArrayList<User>(userSet);
System.out.println("Before sorting");
userList.stream().forEach(System.out::println);
Collections.sort(userList); // This will sort based on date
System.out.println("After sorting");
userList.stream().forEach(System.out::println);
}
}
希望这能澄清你想要的一切。如果您有任何疑问,请告诉我。
执行后,此代码产生以下输出,
userId: 1, userName: Pushpesh, createdDate: 16-02-2018
userId: 2, userName: Vikrant, createdDate: 12-02-2018
userId: 3, userName: Abhay, createdDate: 11-02-2018
userId: 4, userName: Komal, createdDate: 18-02-2018
After removing userId 2
userId: 1, userName: Pushpesh, createdDate: 16-02-2018
userId: 3, userName: Abhay, createdDate: 11-02-2018
userId: 4, userName: Komal, createdDate: 18-02-2018
Before sorting
userId: 1, userName: Pushpesh, createdDate: 16-02-2018
userId: 3, userName: Abhay, createdDate: 11-02-2018
userId: 4, userName: Komal, createdDate: 18-02-2018
After sorting
userId: 3, userName: Abhay, createdDate: 11-02-2018
userId: 1, userName: Pushpesh, createdDate: 16-02-2018
userId: 4, userName: Komal, createdDate: 18-02-2018
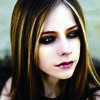
TA贡献2051条经验 获得超10个赞
这是javascript中的示例,但可以使用任何类型的字典/地图轻松将其重写为java。
people = new Map()
user = {id:"das", name: "Darek"}
people.set(user.id, user)
user = {id:"aaa", name: "Czarrek"}
people.set(user.id, user)
user = {id:"bbb", name: "Marek"}
people.set(user.id, user)
console.log(people.get("das"), people.get("aaa"), people.get("bbb"), people.size);
people.delete('aaa');
console.log(people.get("das"), people.get("aaa"), people.get("bbb"), people.size);
映射添加在 O(1) 中工作(对于哈希映射),删除也在 O(1) 中。如果您选择在您的语言中使用的数据结构是使用某种树之王实现的,那么这两个操作都在 O(log(n)) 中运行(可能)。
如果只有随机排序的列表并且没有额外的数据,那么唯一的选择就是查看所有列表的项目。它不能比 O(n) 快
如果您希望能够快速选择/删除项目,您应该从一开始就使用 Map/Hashmap/Dictionary(在您的语言中如何调用它们无关紧要)(即 O(log n) 或更好)) .
添加回答
举报