3 回答
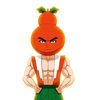
TA贡献1799条经验 获得超8个赞
你不必改变太多。如果对象不存在,则创建它,否则只需设置其属性。您还可以使用对象解构,并且不需要 jQuery:
let validationErrors = []
for(const [key, value] of Object.entries(this.errors)) {
let [ , index, name] = key.split('.');
if(!validationErrors[index]) valudationErrors[index] = {};
validationErrors[index][name] = value;
}
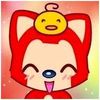
TA贡献1858条经验 获得超8个赞
Object.entries和Array.reduce非常适合做这种事情。
这里建议的解决方案,下面过于冗长的解释。
const input = {
"education_histories.0.school": [
"The education_histories.0.school field is required."
],
"education_histories.0.degree": [
"The education_histories.0.degree field is required."
],
"education_histories.1.school": [
"The education_histories.1.school field is required."
],
"education_histories.1.degree": [
"The education_histories.1.degree field is required."
],
}
// iterate over each key/value in the input object
// to build up the output.
const result = Object.entries(input).reduce((acc, [key, value]) => {
// split the key into parts
// e.g. ['educaton_histories', '0', 'school']
const [section, index, field] = key.split('.');
// use the index to bin the message in the right slot
// e.g. result.0 = { ... }
acc[index] = {
// keep anything that already exists in this bin
...(acc[index] || {}),
// add the message under the field's name
// e.g. school: [ ... ]
[field]: value
}
// return the updated object
return acc;
}, {})
// the object's values are in the format you're after.
// we don't need the keys (the indices)
console.log(Object.values(result));
Object.entries(input)返回一个字段/值对数组。所以:
const input = {
'education_histories.0.school': 'school 0',
'education_histories.0.degree': 'degree 0',
'education_histories.1.school': 'school 1',
'education_histories.1.degree': 'degree 1',
};
Object.entries(input);
返回此数组数组:
[
["education_histories.0.school", "school 0"],
["education_histories.0.degree", "degree 0"],
["education_histories.1.school", "school 1"],
["education_histories.1.degree", "degree 1"],
]
调用reduce此数组会为数组中的每个项目调用回调函数,传入上一次迭代结果和当前数组条目:
// reduce takes two args: a callback function and an initial value
Object.entries(input).reduce(callback, {})
// the callback function takes:
// 1) the result from the previous iteration, and
// 2) and the current array item:
function callback(result, currentItem) {
// on the first iteration:
// previousResult = {}
// currentItem[0] === "education_histories.0.school"
// currentItem[1] === "school 0"
// incorporate the item into the result:
// break the key on '.', and capture the three entries:
// section === 'eduction_histories'
// index === 0
// field === 'school 0'
const [section, index, field] = currentItem[0].split('.');
// result.0
result[index] = {
// if result.0 already exists, keep what's there
...(result[index] || {}),
// add the current item
// school: 'school 0'
[field]: currentItem[1]
}
// return the updated result.
// this will be passed in for the next iteration,
// and will be the final return value when reduce exits
return result;
}
回调可以内联,给你上面的例子。
Object.entries(input).reduce((acc, [key, value]) => {
// ...
}
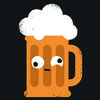
TA贡献2016条经验 获得超9个赞
让我们试试我的方式,我认为这不是最好的解决方案:
const input = {
"education_histories.0.school": [
"The education_histories.0.school field is required."
],
"education_histories.0.degree": [
"The education_histories.0.degree field is required."
],
"education_histories.1.school": [
"The education_histories.1.school field is required."
],
"education_histories.1.degree": [
"The education_histories.1.degree field is required."
],
};
const raw = Object
.keys(input) // ["education_histories.0.school", "education_histories.0.degree"]
.reduce((pre, curr) => {
const splittedStr = curr.split('.');
const dataKey = splittedStr.pop(); // school
const key = splittedStr.join('.'); // education_histories.0
if (!pre[key]) {
pre[key] = {};
}
pre[key][dataKey] = input[curr];
return pre;
}, {});
console.log(raw);
// {
// "education_histories.0": {
// "school": [
// "The education_histories.0.school field is required."
// ],
// "degree": [
// "The education_histories.0.degree field is required."
// ]
// },
// "education_histories.1": {
// "school": [
// "The education_histories.1.school field is required."
// ],
// "degree": [
// "The education_histories.1.degree field is required."
// ]
// }
// }
const output = Object.keys(raw).map((key) => raw[key]);
console.log(output);
添加回答
举报