3 回答
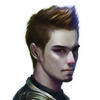
TA贡献1993条经验 获得超5个赞
让我们一行一行地做...
public class ArraysInJava
{
public static void main(String[] args)
{
int[] a = new int[3]; // a = {0, 0, 0} as default value for int elements is 0
a[1] = 50; // a = {0, 50, 0}
Object o = a; // o = a = {0, 50, 0} - casting affects the variable type, the referenced object remains the same (recall that objects are saved by reference)
int[] b = (int[])o; // b = o = a = {0, 50, 0}
b[1] = 100; // b = o = a = {0, 100, 0}
System.out.println(a[1]); // Prints 100
((int[])o)[1] = 500; // b = o = a = {0, 500, 0}
System.out.println(a[1]); // Prints 500
}
}
所有的转换都不做任何事情,因为它只影响你在编译时可以做的事情。例如,你不能写,o[1]因为它在编译时不是数组。
编辑
Carlos Heuberger确实在评论中提出了一个重要观点。我认为缺少的部分是关于数据类型的。
有两种主要的数据类型:原始数据类型和非原始数据类型。
原始数据类型包括byte、short、long、float、double、char、boolean。当您将这些数据类型的变量传递给方法或分配给另一个变量时,您正在传递值。
int a = 15;
int b = a; // b now holds 15
非原始数据类型(您可以称它们为对象类型)是除上述所有类型之外的所有其他类型。这包括数组(也是基本类型的数组)、枚举、类、接口和String.
当您将这些数据类型的变量传递给方法,或将它们分配给另一个变量时,您传递的是对象的引用。
int[] a = {1, 2};
Object b = a; // "b" now holds the reference (memory address) of the "a"
int[] c = b; // "c" now also points to the exact same array
b = null; // "b" holds no memory reference now, "a" and "c" continues to hold reference of the same array
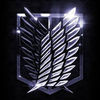
TA贡献1860条经验 获得超8个赞
绘制内存模型并跟踪每个引用,如果您理解这一点,请在代码中的注释中查看我的内容,这将消除您的困惑。 a, b, and o是参考
a[1]------> 50
a[1]------> 50 <------------o[1]
a[1], b[1]------->50 <---------------o[1]
a[1], b[1] -------->100 <-----------o[1] (but the value 50 is overwritten with 100)
a[1], b[1] -------->500<-----------o[1] (but the value 100 is overwritten with 500)
int[] a = new int[3];
a[1] = 50; // a------> 50,
Object o = a; // a------> 50 <------------o
int[] b = (int[])o; // a, b------->50 <---------------o
b[1] = 100; //a, b -------->100 <-----------o(but the value 50 is overwritten with 100)
System.out.println(a[1]); // so prints 100
((int[])o)[1] = 500; //a, b -------->500<-----------o(but the value 100 is overwritten with 500)
System.out.println(a[1]); // hence prints 500
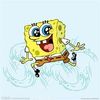
TA贡献1816条经验 获得超4个赞
如果您调试代码,您可以找到以下操作:
首先 a = [0,0,0]
a = [0,50,0]
o = [0,50,0]
b = [0,50,0]
b = [0,100,0] -> o = [0,100,0] -> a = [0,100,0]
打印 a[1] = 100
o[1] = 500 -> o = [0,500,0] -> b = [0,500,0] -> a = [0,500,0]
打印 a[1] = 500
这就是 a[1] 的值是如何改变的。
基本上有相同的数组 a 一直在改变。
添加回答
举报