3 回答
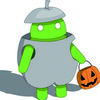
TA贡献1846条经验 获得超7个赞
您可以只从数组中切出第一个和最后一个项目,然后使用array_merge.
例子:
function swapFirstAndLast($array) {
return array_merge(
array_slice($array, -1 , 1), // Last item
array_slice($array, 1 , count($array) - 2), // Second - Second last items
array_slice($array, 0 , 1) // First item
);
}
var_dump(array_column(swapFirstAndLast($pet), 'name'));
//array(4) {
// [0] =>
// string(4) "Name"
// [1] =>
// string(9) "Spark Pug"
// [2] =>
// string(12) "Pico de Gato"
// [3] =>
// string(10) "Chew Barka"
//}
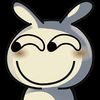
TA贡献2037条经验 获得超6个赞
有很多方法可以给这只猫剥皮。这里有两个函数,第一个总是交换第一个和最后一个元素,第二个交换任意元素。我已经展示了如何使用该函数轻松地交换第一个和最后一个。您可以在 arraySwapFirstLast 函数中使用来自 arraySwap 函数的逻辑,而不是array_shift、array_unshift和 array_pop,它可能会更有效,但这演示了一些重要的数组操作函数。arraySwap 中的简单逻辑是您将在教科书排序算法中使用的,并且非常高效。
<?php
$pet = array(
array(
'name' => 'Chew Barka',
'breed' => 'Bichon',
'age' => '2 years',
'weight' => 8,
'bio' => 'The park, The pool or the Playground - I love to go anywhere! I am really great at... SQUIRREL!',
'filename' => 'pet1.png'
),
array(
'name' => 'Spark Pug',
'breed' => 'Pug',
'age' => '1.5 years',
'weight' => 11,
'bio' => 'You want to go to the dog park in style? Then I am your pug!',
'filename' => 'pet2.png'
),
array(
'name' => 'Pico de Gato',
'breed' => 'Bengal',
'age' => '5 years',
'weight' => 9,
'bio' => 'Oh hai, if you do not have a can of salmon I am not interested.',
'filename' => 'pet3.png'
),
array(
'name' => 'Name',
'breed' => 'Breed',
'age' => 'Age',
'weight' => 'Weight',
'bio' => 'Biography',
'filename' => 'Filename'
)
);
$pet1 = $pet;
$pet2 = $pet;
/**
* Swap the first and last elements of an array
* Uses shift/unshift and standard array append
* @param $array
*/
function arraySwapFirstLast(&$array)
{
$first = array_shift($array);
$last = array_pop($array);
array_unshift($array, $last);
$array[] = $first;
}
/**
* Swap two arbitrary array elements
* @param $array
* @param $index1
* @param $index2
*/
function arraySwap(&$array, $index1, $index2)
{
$swapEl = $array[$index1];
$array[$index1] = $array[$index2];
$array[$index2] = $swapEl;
}
arraySwapFirstLast($pet);
echo 'arraySwapFirstLast:'.PHP_EOL;
print_r($pet).PHP_EOL;
arraySwap($pet1, 1, 2);
echo 'arraySwap second and third elements:'.PHP_EOL;
print_r($pet1).PHP_EOL;
arraySwap($pet2, 0, sizeof($pet2)-1);
echo 'arraySwap first and last elements:'.PHP_EOL;
print_r($pet2).PHP_EOL;
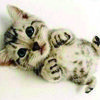
TA贡献1906条经验 获得超3个赞
我想你只是有兴趣在顶部移动标题阵列,你可以做一些圆形阵列移动,如下所示
$arr = array(
array(
'name' => 'Chew Barka',
'breed' => 'Bichon',
'age' => '2 years',
'weight' => 8,
'bio' => 'The park, The pool or the Playground - I love to go anywhere! I am really great at... SQUIRREL!',
'filename' => 'pet1.png'
),
array(
'name' => 'Spark Pug',
'breed' => 'Pug',
'age' => '1.5 years',
'weight' => 11,
'bio' => 'You want to go to the dog park in style? Then I am your pug!',
'filename' => 'pet2.png'
),
array(
'name' => 'Pico de Gato',
'breed' => 'Bengal',
'age' => '5 years',
'weight' => 9,
'bio' => 'Oh hai, if you do not have a can of salmon I am not interested.',
'filename' => 'pet3.png'
),
array(
'name' => 'Name',
'breed' => 'Breed',
'age' => 'Age',
'weight' => 'Weight',
'bio' => 'Biography',
'filename' => 'Filename'
)
);
echo "<pre>";
$key=count($arr)-1;
$output1 = array_slice($arr, $key);
$output2 = array_slice($arr, 0,$key);
$new=array_merge($output1,$output2);
print_r($new);
输出
Array
(
[0] => Array
(
[name] => Name
[breed] => Breed
[age] => Age
[weight] => Weight
[bio] => Biography
[filename] => Filename
)
[1] => Array
(
[name] => Chew Barka
[breed] => Bichon
[age] => 2 years
[weight] => 8
[bio] => The park, The pool or the Playground - I love to go anywhere! I am really great at... SQUIRREL!
[filename] => pet1.png
)
[2] => Array
(
[name] => Spark Pug
[breed] => Pug
[age] => 1.5 years
[weight] => 11
[bio] => You want to go to the dog park in style? Then I am your pug!
[filename] => pet2.png
)
[3] => Array
(
[name] => Pico de Gato
[breed] => Bengal
[age] => 5 years
[weight] => 9
[bio] => Oh hai, if you do not have a can of salmon I am not interested.
[filename] => pet3.png
)
)
- 3 回答
- 0 关注
- 231 浏览
添加回答
举报