public Class Point{ private double x; private double y; public Point() { super(); } public Point(double x, double y) { super(); this.x = x; this.y = y; } public static Point deepCopy(Point p2) { Point point2 = new Point(p2.x+2, p2.y+2); return point2; } public static Point shallowCopy(Point p4){ return p4; } public void setPoint3X(double x3) { this.x = x+1; } public void setPoint3Y(double y3) { this.y = y+1; } public void setPoint2(double x2, double y2) { this.x = x2+2; this.y = y2+2; } public double getX() { return x; } public void setX(double x) { this.x = x; } public double getY() { return y; } public void setY(double y) { this.y = y; } @Override public String toString() { return "Point [x=" + x + ", y=" + y + "]"; }public class PointDemo { public static void main(String[] args) { double x = 0; double y = 0; Point point1 = new Point(5, 10); Point point2 = Point.deepCopy(point1); Point point3 = Point.deepCopy(point2); point3.setPoint3X(x); point3.setPoint3Y(y); Point point4 = new Point(); point4 = Point.shallowCopy(point3);问题 4 - 编写一个名为 Point 的类。该类有两个实例字段:x 和 y,都是 double 类型。编写两个构造函数:一个使用 x 和 y 值作为一个点,另一个使用第一个点值创建具有完全相同 x 和 y 值的第二个 Point 对象。编写一个 Demo 类来构建以下四个 Point 对象。第 1 点:(x=5,y=10)第 2 点:(x=7,x=12)。该点需要使用复制点 1 的深拷贝构造函数来构建,然后仅使用一个 setter 方法。第 3 点:(x=10,y=15)。该点需要使用深度复制方法构建,该方法使用点 2 作为原始值,然后使用两个 setter 方法更改所需的 x 和 y 值。第4点:这个点需要使用浅拷贝的方式构建,并且必须使用第3点作为浅拷贝模板。最后使用一个语句打印所有四个点。好的。因此,我的代码为我提供了 point1-point4 中的所有值,但是,我无法想出一种方法将它们全部打印在一个语句中。显然,演示类中的循环可以打印每个 Point 对象,但这将是多个打印语句,这违反了一个打印语句的要求。另外,我不能在 Point 类中使用数组,因为它违反了 2 个字段的要求。关于如何获取所有 Point 对象并将其打印在一个语句中,任何人都可以帮助或给我建议吗?或者这是可能的,也许我读错了问题?
3 回答
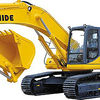
千巷猫影
TA贡献1829条经验 获得超7个赞
您可以使用PrintStream.format(format(String format, Object... args):
System.out.format("(%f, %f), (%f, %f), (%f, %f), (%f, %f)\n", point1.x, point1.y, point2.x, point2.y, ...and so on);
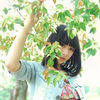
RISEBY
TA贡献1856条经验 获得超5个赞
我也会将此作为答案发布,因为我认为这可能是您的讲师真正想要的。
这里的关键点是要记住,toString()您的类中的方法可以像常规字符串一样使用并连接其他字符串,这就是您+在调用println(). 因此,只需使用println()您可能已经在做的常规方法。
System.out.println( "Point 1 - " + point1.toString() + ";\n"
+ "Point 2 - " + point2.toString() + ";\n"
+ "Point 3 - " + point3.toString() + ";\n"
+ "Point 4 - " + point4.toString() + ";" );
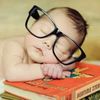
长风秋雁
TA贡献1757条经验 获得超7个赞
您可以使用流:
Arrays.stream(new Point[] {point1, point2, point3, point4}).forEach(System.out::println);
或者 String.format()
System.out::println(String.format("%s %s %s %s", point1, point2, point3, point4));
添加回答
举报
0/150
提交
取消