3 回答
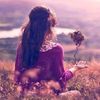
TA贡献1842条经验 获得超21个赞
使用 Gofmt包。例如,
package main
import "fmt"
func main() {
variable := "var"
fmt.Println(variable)
fmt.Printf("%#v\n", variable)
header := map[string]string{"content-type": "text/plain"}
fmt.Println(header)
fmt.Printf("%#v\n", header)
}
输出:
var
"var"
map[content-type:text/plain]
map[string]string{"content-type":"text/plain"}
包文件
import "fmt"
概述
包 fmt 使用类似于 C 的 printf 和 scanf 的函数实现格式化的 I/O。“动词”格式源自 C,但更简单。
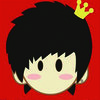
TA贡献1898条经验 获得超8个赞
我认为在很多情况下,使用 "%v" 已经足够简洁了:
fmt.Printf("%v", myVar)
从 fmt 包的文档页面:
%v 默认格式的值。打印结构时,加号 ( %+v ) 添加字段名称
%#v 值 的 Go 语法表示
下面是一个例子:
package main
import "fmt"
func main() {
// Define a struct, slice and map
type Employee struct {
id int
name string
age int
}
var eSlice []Employee
var eMap map[int]Employee
e1 := Employee{1, "Alex", 20}
e2 := Employee{2, "Jack", 30}
fmt.Printf("%v\n", e1)
// output: {1 Alex 20}
fmt.Printf("%+v\n", e1)
// output: {id:1 name:Alex age:20}
fmt.Printf("%#v\n", e1)
// output: main.Employee{id:1, name:"Alex", age:20}
eSlice = append(eSlice, e1, e2)
fmt.Printf("%v\n", eSlice)
// output: [{1 Alex 20} {2 Jack 30}]
fmt.Printf("%#v\n", eSlice)
// output: []main.Employee{main.Employee{id:1, name:"Alex", age:20}, main.Employee{id:2, name:"Jack", age:30}}
eMap = make(map[int]Employee)
eMap[1] = e1
eMap[2] = e2
fmt.Printf("%v\n", eMap)
// output: map[1:{1 Alex 20} 2:{2 Jack 30}]
fmt.Printf("%#v\n", eMap)
// output: map[int]main.Employee{1:main.Employee{id:1, name:"Alex", age:20}, 2:main.Employee{id:2, name:"Jack", age:30}}
}
- 3 回答
- 0 关注
- 204 浏览
添加回答
举报