3 回答
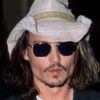
TA贡献1765条经验 获得超5个赞
您可以简单地解析如下
创建一个模型类作为
public class TimeSlot {
public String TimeSlotId;
public String TimeOfSlot;
public String TimeofSlotDateTime;
public TimeSlot(String timeSlotId, String timeOfSlot, String timeofSlotDateTime) {
TimeSlotId = timeSlotId;
TimeOfSlot = timeOfSlot;
TimeofSlotDateTime = timeofSlotDateTime;
}
}
然后将这个类与ArrayList.
ArrayList<TimeSlot> ovbjTimeSlots = new ArrayList<>();
try {
JSONObject jo = new JSONObject(response);
JSONArray jaDetail = jo.getJSONArray("Detail");
for (int i = 0; i < jaDetail.length(); i++) {
JSONArray jsonArray = jaDetail.getJSONObject(i).getJSONArray("AvailableTimeSlots");
for (int j = 0; j < jsonArray.length(); j++) {
String TimeSlotId = jsonArray.getJSONObject(i).getString("TimeSlotId");
String TimeOfSlot = jsonArray.getJSONObject(i).getString("TimeOfSlot");
String TimeofSlotDateTime = jsonArray.getJSONObject(i).getString("TimeofSlotDateTime");
ovbjTimeSlots.add(new TimeSlot(TimeSlotId, TimeOfSlot, TimeofSlotDateTime));
}
}
} catch (JSONException e) {
e.printStackTrace();
}
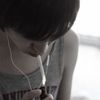
TA贡献1844条经验 获得超8个赞
我用jackjson来解析;
private List<String> parseString(String str) throws IOException {
if(str == null){
return null;
}
List<String> list =new ArrayList<>();
ObjectMapper mapper = new ObjectMapper();
JsonNode rootNode = mapper.readTree(str).get("Detail");
for(int i=0 ; i< rootNode.size() ;i++){
JsonNode rootnode1 =rootNode.get(i).get("AvailableTimeSlots");
for(int j =0 ; j<rootnode1.size() ;j++){
JsonNode rootnode2 =rootnode1.get(j);
list.add((rootnode2.get("TimeOfSlot")).toString());
}
}
return list;
}
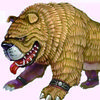
TA贡献1810条经验 获得超5个赞
package com.Model;
import com.google.gson.annotations.Expose;
import com.google.gson.annotations.SerializedName;
import java.util.List;
public class Model_Json {
@SerializedName("Response")
@Expose
private Response response;
@SerializedName("Detail")
@Expose
private List<Detail> detail = null;
public Response getResponse() {
return response;
}
public void setResponse(Response response) {
this.response = response;
}
public List<Detail> getDetail() {
return detail;
}
public void setDetail(List<Detail> detail) {
this.detail = detail;
}
public class Response {
@SerializedName("ResponseCode")
@Expose
private Integer responseCode;
@SerializedName("ResponseText")
@Expose
private String responseText;
public Integer getResponseCode() {
return responseCode;
}
public void setResponseCode(Integer responseCode) {
this.responseCode = responseCode;
}
public String getResponseText() {
return responseText;
}
public void setResponseText(String responseText) {
this.responseText = responseText;
}
}
public class GetBlockedTiming {
@SerializedName("DateOfSlot")
@Expose
private String dateOfSlot;
@SerializedName("AvailableTimeSlots")
@Expose
private Object availableTimeSlots;
@SerializedName("ScheduleId")
@Expose
private Integer scheduleId;
@SerializedName("GetBlockedTimings")
@Expose
private Object getBlockedTimings;
@SerializedName("BlockId")
@Expose
private Integer blockId;
@SerializedName("fkTimeId")
@Expose
private Integer fkTimeId;
@SerializedName("IsDeleted")
@Expose
private Boolean isDeleted;
@SerializedName("fkScheduledId")
@Expose
private Integer fkScheduledId;
@SerializedName("utcDateOfSlot")
@Expose
private String utcDateOfSlot;
public String getDateOfSlot() {
return dateOfSlot;
}
public void setDateOfSlot(String dateOfSlot) {
this.dateOfSlot = dateOfSlot;
}
public Object getAvailableTimeSlots() {
return availableTimeSlots;
}
public void setAvailableTimeSlots(Object availableTimeSlots) {
this.availableTimeSlots = availableTimeSlots;
}
public Integer getScheduleId() {
return scheduleId;
}
public void setScheduleId(Integer scheduleId) {
this.scheduleId = scheduleId;
}
public Object getGetBlockedTimings() {
return getBlockedTimings;
}
public void setGetBlockedTimings(Object getBlockedTimings) {
this.getBlockedTimings = getBlockedTimings;
}
public Integer getBlockId() {
return blockId;
}
public void setBlockId(Integer blockId) {
this.blockId = blockId;
}
public Integer getFkTimeId() {
return fkTimeId;
}
public void setFkTimeId(Integer fkTimeId) {
this.fkTimeId = fkTimeId;
}
public Boolean getIsDeleted() {
return isDeleted;
}
public void setIsDeleted(Boolean isDeleted) {
this.isDeleted = isDeleted;
}
public Integer getFkScheduledId() {
return fkScheduledId;
}
public void setFkScheduledId(Integer fkScheduledId) {
this.fkScheduledId = fkScheduledId;
}
public String getUtcDateOfSlot() {
return utcDateOfSlot;
}
public void setUtcDateOfSlot(String utcDateOfSlot) {
this.utcDateOfSlot = utcDateOfSlot;
}
}
public class Detail {
@SerializedName("DateOfSlot")
@Expose
private String dateOfSlot;
@SerializedName("AvailableTimeSlots")
@Expose
private List<AvailableTimeSlot> availableTimeSlots = null;
@SerializedName("ScheduleId")
@Expose
private Integer scheduleId;
@SerializedName("GetBlockedTimings")
@Expose
private List<GetBlockedTiming> getBlockedTimings = null;
@SerializedName("BlockId")
@Expose
private Integer blockId;
@SerializedName("fkTimeId")
@Expose
private Integer fkTimeId;
@SerializedName("IsDeleted")
@Expose
private Boolean isDeleted;
@SerializedName("fkScheduledId")
@Expose
private Integer fkScheduledId;
@SerializedName("utcDateOfSlot")
@Expose
private String utcDateOfSlot;
public String getDateOfSlot() {
return dateOfSlot;
}
public void setDateOfSlot(String dateOfSlot) {
this.dateOfSlot = dateOfSlot;
}
public List<AvailableTimeSlot> getAvailableTimeSlots() {
return availableTimeSlots;
}
public void setAvailableTimeSlots(List<AvailableTimeSlot> availableTimeSlots) {
this.availableTimeSlots = availableTimeSlots;
}
public Integer getScheduleId() {
return scheduleId;
}
public void setScheduleId(Integer scheduleId) {
this.scheduleId = scheduleId;
}
public List<GetBlockedTiming> getGetBlockedTimings() {
return getBlockedTimings;
}
public void setGetBlockedTimings(List<GetBlockedTiming> getBlockedTimings) {
this.getBlockedTimings = getBlockedTimings;
}
public Integer getBlockId() {
return blockId;
}
public void setBlockId(Integer blockId) {
this.blockId = blockId;
}
public Integer getFkTimeId() {
return fkTimeId;
}
public void setFkTimeId(Integer fkTimeId) {
this.fkTimeId = fkTimeId;
}
public Boolean getIsDeleted() {
return isDeleted;
}
public void setIsDeleted(Boolean isDeleted) {
this.isDeleted = isDeleted;
}
public Integer getFkScheduledId() {
return fkScheduledId;
}
public void setFkScheduledId(Integer fkScheduledId) {
this.fkScheduledId = fkScheduledId;
}
public String getUtcDateOfSlot() {
return utcDateOfSlot;
}
public void setUtcDateOfSlot(String utcDateOfSlot) {
this.utcDateOfSlot = utcDateOfSlot;
}
}
public class AvailableTimeSlot {
@SerializedName("TimeSlotId")
@Expose
private Integer timeSlotId;
@SerializedName("TimeOfSlot")
@Expose
private String timeOfSlot;
@SerializedName("TimeofSlotDateTime")
@Expose
private String timeofSlotDateTime;
public Integer getTimeSlotId() {
return timeSlotId;
}
public void setTimeSlotId(Integer timeSlotId) {
this.timeSlotId = timeSlotId;
}
public String getTimeOfSlot() {
return timeOfSlot;
}
public void setTimeOfSlot(String timeOfSlot) {
this.timeOfSlot = timeOfSlot;
}
public String getTimeofSlotDateTime() {
return timeofSlotDateTime;
}
public void setTimeofSlotDateTime(String timeofSlotDateTime) {
this.timeofSlotDateTime = timeofSlotDateTime;
}
}
}
实现 'com.google.code.gson:gson:2.2.4'
List<Model_Json> jsonList= new Gson().fromJson("your_json",new TypeToken<List<Model_Json>>(){}.getType());
List<Model_Json.AvailableTimeSlot> timeSlots =jsonList.get(0).getDetail().get(0).getAvailableTimeSlots();
这里 timeSlots 是一个列表,您可以使用它来设置适配器。
ArrayAdapterItem adapter = new ArrayAdapterItem(this, R.layout.list_view_row_item, timeSlots);
ListView listViewItems = findViewById(R.id.yourId);
listViewItems.setAdapter(adapter);
// here's our beautiful adapter
public class ArrayAdapterItem extends ArrayAdapter<Model_Json.AvailableTimeSlot> {
Context mContext;
int layoutResourceId;
List<Model_Json.AvailableTimeSlot> data;
public ArrayAdapterItem(Context mContext, int layoutResourceId, List<Model_Json.AvailableTimeSlot> data) {
super(mContext, layoutResourceId, data);
this.layoutResourceId = layoutResourceId;
this.mContext = mContext;
this.data = data;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
if(convertView==null){
// inflate the layout
LayoutInflater inflater = ((Activity) mContext).getLayoutInflater();
convertView = inflater.inflate(layoutResourceId, parent, false);
}
// object item based on the position
Model_Json.AvailableTimeSlot objectItem = data.get(position);
// get the TextView and then set the text (item name) and tag (item ID) values
TextView textViewItem = (TextView) convertView.findViewById(R.id.textViewItem);
textViewItem.setText(objectItem.getTimeOfSlot());
return convertView;
}
}
list_view_row_item.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:padding="10dp" >
<TextView
android:id="@+id/textViewItem"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:text="Item name here..."
android:textSize="15dp" />
</RelativeLayout>
添加回答
举报