3 回答
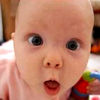
TA贡献1808条经验 获得超4个赞
首先,您可以找到行星和恒星元素,将它们添加到列表中,然后从中选择第一个值。
import xml.etree.ElementTree as ET
dump = ET.parse('planet_info.xml')
root = dump.getroot()
planet_name = star_name = planet_list = []
for content in root:
planet_name = content.findall("planet")
star_name = content.findall("star")
if planet_name:
for planet in planet_name:
planet_list.append(Planet(planet[0].text, planet[1].text, etc)
elif star_name:
for star in star_name:
planet_list.append(Planet(star[0].text, star[1].text, etc)
else:
print("Nothing")
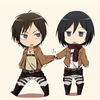
TA贡献1821条经验 获得超4个赞
此代码使用etree起作用。您缺少一行仅返回item已name设置为某个值的元素的行。
import xml.etree.ElementTree as ET
root = ET.parse(PATH_TO_YOUR_FILE)
planets = root.findall('./planets/planet')
planets_list = []
for planet in planets:
name = planet.find("./item[@name='Name']").text
d = planet.find("./item[@name='Distance from the Earth']").text
//...
planets_list.append(Planet(name, d, ...)
从这里您应该可以自己解决其余的问题。希望能帮助到你。
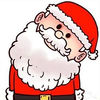
TA贡献1824条经验 获得超6个赞
这是一种遍历该数据结构的简单方法。为了使代码保持独立,我直接从字符串而不是从文件读取XML。
import xml.etree.ElementTree as ET
data = '''\
<?xml version="1.0"?>
<celestialBodies>
<stars>
<star>
<item name="Name">Sun</item>
<item name="Distance from the Earth">100000</item>
<item name="Size">9</item>
<item name="Moons">A,B,C</item>
<item name="Gravity">0.4</item>
<item name="Elements Found">H, HE</item>
<item name="Orbiting Time">15</item>
<item name="Day Time">12</item>
</star>
</stars>
<planets>
<planet>
<item name="Name">Mercury</item>
<item name="Distance from the Earth">100000</item>
<item name="Size">9</item>
<item name="Moons">A,B,C</item>
<item name="Gravity">0.4</item>
<item name="Elements Found">H, HE, C</item>
<item name="Orbiting Time">15</item>
<item name="Day Time">12</item>
</planet>
<planet>
<item name="Name">Venus</item>
<item name="Distance from the Earth">100000</item>
<item name="Size">9</item>
<item name="Moons">A,B,C</item>
<item name="Gravity">0.4</item>
<item name="Elements Found">H, HE, C</item>
<item name="Orbiting Time">15</item>
<item name="Day Time">12</item>
</planet>
<planet>
<item name="Name">Earth</item>
<item name="Distance from the Earth">0</item>
<item name="Size">9</item>
<item name="Moons">A,B,C</item>
<item name="Gravity">0.4</item>
<item name="Elements Found">H, HE, C</item>
<item name="Orbiting Time">15</item>
<item name="Day Time">12</item>
</planet>
<planet>
<item name="Name">Mars</item>
<item name="Distance from the Earth">100000</item>
<item name="Size">9</item>
<item name="Moons">A,B,C</item>
<item name="Gravity">0.4</item>
<item name="Elements Found">H, HE, C</item>
<item name="Orbiting Time">15</item>
<item name="Day Time">12</item>
</planet>
<planet>
<item name="Name">Jupiter</item>
<item name="Distance from the Earth">100000</item>
<item name="Size">9</item>
<item name="Moons">A,B,C</item>
<item name="Gravity">0.4</item>
<item name="Elements Found">H, HE, C</item>
<item name="Orbiting Time">15</item>
<item name="Day Time">12</item>
</planet>
<planet>
<item name="Name">Saturn</item>
<item name="Distance from the Earth">100000</item>
<item name="Size">9</item>
<item name="Moons">A,B,C</item>
<item name="Gravity">0.4</item>
<item name="Elements Found">H, HE, C</item>
<item name="Orbiting Time">15</item>
<item name="Day Time">12</item>
</planet>
<planet>
<item name="Name">Uranus</item>
<item name="Distance from the Earth">100000</item>
<item name="Size">9</item>
<item name="Moons">A,B,C</item>
<item name="Gravity">0.4</item>
<item name="Elements Found">H, HE, C</item>
<item name="Orbiting Time">15</item>
<item name="Day Time">12</item>
</planet>
<planet>
<item name="Name">Neptune</item>
<item name="Distance from the Earth">100000</item>
<item name="Size">9</item>
<item name="Moons">A,B,C</item>
<item name="Gravity">0.4</item>
<item name="Elements Found">H, HE, C</item>
<item name="Orbiting Time">15</item>
<item name="Day Time">12</item>
</planet>
<planet>
<item name="Name">Pluto</item>
<item name="Distance from the Earth">100000</item>
<item name="Size">9</item>
<item name="Moons">A,B,C</item>
<item name="Gravity">0.4</item>
<item name="Elements Found">H, HE, C</item>
<item name="Orbiting Time">15</item>
<item name="Day Time">12</item>
</planet>
</planets>
</celestialBodies>
'''
root = ET.fromstring(data)
for kind in root:
print(kind.tag)
for child in kind:
print(' '*2, child.tag)
for item in child:
print(' '*4, '{!r}: {!r}'.format(item.attrib['name'], item.text))
print()
输出
stars
star
'Name': 'Sun'
'Distance from the Earth': '100000'
'Size': '9'
'Moons': 'A,B,C'
'Gravity': '0.4'
'Elements Found': 'H, HE'
'Orbiting Time': '15'
'Day Time': '12'
planets
planet
'Name': 'Mercury'
'Distance from the Earth': '100000'
'Size': '9'
'Moons': 'A,B,C'
'Gravity': '0.4'
'Elements Found': 'H, HE, C'
'Orbiting Time': '15'
'Day Time': '12'
planet
'Name': 'Venus'
'Distance from the Earth': '100000'
'Size': '9'
'Moons': 'A,B,C'
'Gravity': '0.4'
'Elements Found': 'H, HE, C'
'Orbiting Time': '15'
'Day Time': '12'
planet
'Name': 'Earth'
'Distance from the Earth': '0'
'Size': '9'
'Moons': 'A,B,C'
'Gravity': '0.4'
'Elements Found': 'H, HE, C'
'Orbiting Time': '15'
'Day Time': '12'
planet
'Name': 'Mars'
'Distance from the Earth': '100000'
'Size': '9'
'Moons': 'A,B,C'
'Gravity': '0.4'
'Elements Found': 'H, HE, C'
'Orbiting Time': '15'
'Day Time': '12'
planet
'Name': 'Jupiter'
'Distance from the Earth': '100000'
'Size': '9'
'Moons': 'A,B,C'
'Gravity': '0.4'
'Elements Found': 'H, HE, C'
'Orbiting Time': '15'
'Day Time': '12'
planet
'Name': 'Saturn'
'Distance from the Earth': '100000'
'Size': '9'
'Moons': 'A,B,C'
'Gravity': '0.4'
'Elements Found': 'H, HE, C'
'Orbiting Time': '15'
'Day Time': '12'
planet
'Name': 'Uranus'
'Distance from the Earth': '100000'
'Size': '9'
'Moons': 'A,B,C'
'Gravity': '0.4'
'Elements Found': 'H, HE, C'
'Orbiting Time': '15'
'Day Time': '12'
planet
'Name': 'Neptune'
'Distance from the Earth': '100000'
'Size': '9'
'Moons': 'A,B,C'
'Gravity': '0.4'
'Elements Found': 'H, HE, C'
'Orbiting Time': '15'
'Day Time': '12'
planet
'Name': 'Pluto'
'Distance from the Earth': '100000'
'Size': '9'
'Moons': 'A,B,C'
'Gravity': '0.4'
'Elements Found': 'H, HE, C'
'Orbiting Time': '15'
'Day Time': '12'
这种变化将数据加载到包含两个列表的字典中,一个列表用于恒星,一个列表用于行星。这些列表中的每个列表都包含该类别中每个正文的字典。我们可以使用该json模块很好地打印数据。
import xml.etree.ElementTree as ET
import json
root = ET.fromstring(data)
bodies = {}
for kind in root:
bodies[kind.tag] = bodylist = []
for child in kind:
bodylist.append({item.attrib['name']: item.text for item in child})
print(json.dumps(bodies, indent=4))
输出
{
"stars": [
{
"Name": "Sun",
"Distance from the Earth": "100000",
"Size": "9",
"Moons": "A,B,C",
"Gravity": "0.4",
"Elements Found": "H, HE",
"Orbiting Time": "15",
"Day Time": "12"
}
],
"planets": [
{
"Name": "Mercury",
"Distance from the Earth": "100000",
"Size": "9",
"Moons": "A,B,C",
"Gravity": "0.4",
"Elements Found": "H, HE, C",
"Orbiting Time": "15",
"Day Time": "12"
},
{
"Name": "Venus",
"Distance from the Earth": "100000",
"Size": "9",
"Moons": "A,B,C",
"Gravity": "0.4",
"Elements Found": "H, HE, C",
"Orbiting Time": "15",
"Day Time": "12"
},
{
"Name": "Earth",
"Distance from the Earth": "0",
"Size": "9",
"Moons": "A,B,C",
"Gravity": "0.4",
"Elements Found": "H, HE, C",
"Orbiting Time": "15",
"Day Time": "12"
},
{
"Name": "Mars",
"Distance from the Earth": "100000",
"Size": "9",
"Moons": "A,B,C",
"Gravity": "0.4",
"Elements Found": "H, HE, C",
"Orbiting Time": "15",
"Day Time": "12"
},
{
"Name": "Jupiter",
"Distance from the Earth": "100000",
"Size": "9",
"Moons": "A,B,C",
"Gravity": "0.4",
"Elements Found": "H, HE, C",
"Orbiting Time": "15",
"Day Time": "12"
},
{
"Name": "Saturn",
"Distance from the Earth": "100000",
"Size": "9",
"Moons": "A,B,C",
"Gravity": "0.4",
"Elements Found": "H, HE, C",
"Orbiting Time": "15",
"Day Time": "12"
},
{
"Name": "Uranus",
"Distance from the Earth": "100000",
"Size": "9",
"Moons": "A,B,C",
"Gravity": "0.4",
"Elements Found": "H, HE, C",
"Orbiting Time": "15",
"Day Time": "12"
},
{
"Name": "Neptune",
"Distance from the Earth": "100000",
"Size": "9",
"Moons": "A,B,C",
"Gravity": "0.4",
"Elements Found": "H, HE, C",
"Orbiting Time": "15",
"Day Time": "12"
},
{
"Name": "Pluto",
"Distance from the Earth": "100000",
"Size": "9",
"Moons": "A,B,C",
"Gravity": "0.4",
"Elements Found": "H, HE, C",
"Orbiting Time": "15",
"Day Time": "12"
}
]
}
添加回答
举报