3 回答
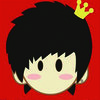
TA贡献1898条经验 获得超8个赞
将您的所有文件读入List某种形式的文件:
List<File> filesInFolder = Files.walk(Paths.get("\\path\\to\\folder"))
.filter(Files::isRegularFile)
.map(Path::toFile)
.collect(Collectors.toList());
然后循环遍历它们,如果文件未以所需的扩展名结尾,则将其删除:
filesInFolder.stream().filter((file) -> (!file.toString().endsWith(".jpg"))).forEach((file) -> {
file.delete();
});
您可以根据自己的特定需求进行调整。
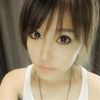
TA贡献1813条经验 获得超2个赞
这是MCVE:
本示例实现了Set通过仅提供包含图像的文件夹/目录的路径来自动删除重复图像的方法(只是一个不同的想法,以显示其他可用选项以及如何利用Java中的OO功能)
import java.io.File;
import java.util.HashSet;
import java.util.Set;
public class DuplicateRemover {
// inner class to represent an image
class Image{
String path; // the absolute path of image file as a String
// constructor
public Image(String path) {
this.path = path;
}
@Override
public boolean equals(Object o) {
if(o instanceof Image){
// if both base names are equal -> delete the old one
if(getBaseName(this.path).equals(getBaseName(((Image)o).path))){
File file = new File(this.path);
return file.delete();
}
}
return false;
}
@Override
public int hashCode() {
return 0; // in this case, only "equals()" method is considered for duplicate check
}
/**
* This method to get the Base name of the image from the path
* @param fileName
* @return
*/
private String getBaseName(String fileName) {
int index = fileName.lastIndexOf('.');
if (index == -1) { return fileName; }
else { return fileName.substring(0, index); }
}
}
Set<Image> images; // a set of image files
//constructor
public DuplicateRemover(){
images = new HashSet<>();
}
/**
* Get the all the images from the given folder
* and loop through all files to add them to the images set
* @param dirPath
*/
public void run(String dirPath){
File dir = new File(dirPath);
File[] listOfImages = dir.listFiles();
for (File f : listOfImages){
if (f.isFile()) {
images.add(new Image(f.getAbsolutePath()));
}
}
}
//TEST
public static void main(String[] args) {
String dirPath = "C:\\Users\\Yahya Almardeny\\Desktop\\folder";
/* dir contains: {image1.png, image1.jpeg, image1.jpg, image2.png} */
DuplicateRemover dr = new DuplicateRemover();
// the images set will delete any duplicate image from the folder
// according to the logic we provided in the "equals()" method
dr.run(dirPath);
// print what images left in the folder
for(Image image : dr.images) {
System.out.println(image.path);
}
//Note that you can use the set for further manipulation if you have in later
}
}
结果
C:\Users\Yahya Almardeny\Desktop\folder\image1.jpeg
C:\Users\Yahya Almardeny\Desktop\folder\image2.png
添加回答
举报