3 回答
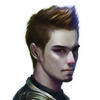
TA贡献1777条经验 获得超3个赞
这是我的CORS中间件:
<?php namespace App\Http\Middleware;
use Closure;
class CORS {
/**
* Handle an incoming request.
*
* @param \Illuminate\Http\Request $request
* @param \Closure $next
* @return mixed
*/
public function handle($request, Closure $next)
{
header("Access-Control-Allow-Origin: *");
// ALLOW OPTIONS METHOD
$headers = [
'Access-Control-Allow-Methods'=> 'POST, GET, OPTIONS, PUT, DELETE',
'Access-Control-Allow-Headers'=> 'Content-Type, X-Auth-Token, Origin'
];
if($request->getMethod() == "OPTIONS") {
// The client-side application can set only headers allowed in Access-Control-Allow-Headers
return Response::make('OK', 200, $headers);
}
$response = $next($request);
foreach($headers as $key => $value)
$response->header($key, $value);
return $response;
}
}
要使用CORS中间件,您必须先在app \ Http \ Kernel.php文件中注册它,如下所示:
protected $routeMiddleware = [
//other middlewares
'cors' => 'App\Http\Middleware\CORS',
];
然后您可以在路线中使用它
Route::get('example', array('middleware' => 'cors', 'uses' => 'ExampleController@dummy'));
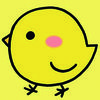
TA贡献1911条经验 获得超7个赞
我总是使用一种简单的方法。只需将以下行添加到\public\index.php文件即可。我认为您不必使用中间件。
header('Access-Control-Allow-Origin: *');
header('Access-Control-Allow-Methods: GET, PUT, POST, DELETE, OPTIONS');
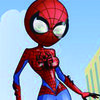
TA贡献1851条经验 获得超4个赞
我使用的是Laravel 5.4,不幸的是,尽管接受的答案似乎很好,但对于预检请求(如PUT和DELETE),该OPTIONS请求将在请求之前,在$routeMiddleware数组中指定中间件(并在路由定义文件中使用),除非您也定义一个路由处理程序OPTIONS。这是因为如果没有OPTIONS路由,Laravel将在内部响应该方法而没有CORS标头。
简而言之,要么在$middleware数组中定义针对所有请求全局运行的中间件,要么在其中进行操作,$middlewareGroups或者$routeMiddleware为定义路由处理程序OPTIONS。可以这样完成:
Route::match(['options', 'put'], '/route', function () {
// This will work with the middleware shown in the accepted answer
})->middleware('cors');
我还出于相同的目的编写了一个中间件,该中间件看起来很相似,但是由于试图更好地配置和处理一系列条件,因此其尺寸更大:
<?php
namespace App\Http\Middleware;
use Closure;
class Cors
{
private static $allowedOriginsWhitelist = [
'http://localhost:8000'
];
// All the headers must be a string
private static $allowedOrigin = '*';
private static $allowedMethods = 'OPTIONS, GET, POST, PUT, PATCH, DELETE';
private static $allowCredentials = 'true';
private static $allowedHeaders = '';
/**
* Handle an incoming request.
*
* @param \Illuminate\Http\Request $request
* @param \Closure $next
* @return mixed
*/
public function handle($request, Closure $next)
{
if (! $this->isCorsRequest($request))
{
return $next($request);
}
static::$allowedOrigin = $this->resolveAllowedOrigin($request);
static::$allowedHeaders = $this->resolveAllowedHeaders($request);
$headers = [
'Access-Control-Allow-Origin' => static::$allowedOrigin,
'Access-Control-Allow-Methods' => static::$allowedMethods,
'Access-Control-Allow-Headers' => static::$allowedHeaders,
'Access-Control-Allow-Credentials' => static::$allowCredentials,
];
// For preflighted requests
if ($request->getMethod() === 'OPTIONS')
{
return response('', 200)->withHeaders($headers);
}
$response = $next($request)->withHeaders($headers);
return $response;
}
/**
* Incoming request is a CORS request if the Origin
* header is set and Origin !== Host
*
* @param \Illuminate\Http\Request $request
*/
private function isCorsRequest($request)
{
$requestHasOrigin = $request->headers->has('Origin');
if ($requestHasOrigin)
{
$origin = $request->headers->get('Origin');
$host = $request->getSchemeAndHttpHost();
if ($origin !== $host)
{
return true;
}
}
return false;
}
/**
* Dynamic resolution of allowed origin since we can't
* pass multiple domains to the header. The appropriate
* domain is set in the Access-Control-Allow-Origin header
* only if it is present in the whitelist.
*
* @param \Illuminate\Http\Request $request
*/
private function resolveAllowedOrigin($request)
{
$allowedOrigin = static::$allowedOrigin;
// If origin is in our $allowedOriginsWhitelist
// then we send that in Access-Control-Allow-Origin
$origin = $request->headers->get('Origin');
if (in_array($origin, static::$allowedOriginsWhitelist))
{
$allowedOrigin = $origin;
}
return $allowedOrigin;
}
/**
* Take the incoming client request headers
* and return. Will be used to pass in Access-Control-Allow-Headers
*
* @param \Illuminate\Http\Request $request
*/
private function resolveAllowedHeaders($request)
{
$allowedHeaders = $request->headers->get('Access-Control-Request-Headers');
return $allowedHeaders;
}
}
- 3 回答
- 0 关注
- 794 浏览
添加回答
举报