3 回答
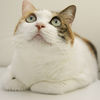
TA贡献1798条经验 获得超3个赞
首先,您需要继承UIImageView并重写drawRect方法。您的类需要一个UIColor属性(我们将其称为overlayColor)来保存混合颜色,以及一个自定义设置器,当颜色更改时,该设置器将强制重绘。像这样:
- (void) setOverlayColor:(UIColor *)newColor {
if (overlayColor)
[overlayColor release];
overlayColor = [newColor retain];
[self setNeedsDisplay]; // fires off drawRect each time color changes
}
在drawRect方法中,您将要先绘制图像,然后在其上覆盖一个填充有所需颜色的矩形以及适当的混合模式,如下所示:
- (void) drawRect:(CGRect)area
{
CGContextRef context = UIGraphicsGetCurrentContext();
CGContextSaveGState(context);
// Draw picture first
//
CGContextDrawImage(context, self.frame, self.image.CGImage);
// Blend mode could be any of CGBlendMode values. Now draw filled rectangle
// over top of image.
//
CGContextSetBlendMode (context, kCGBlendModeMultiply);
CGContextSetFillColor(context, CGColorGetComponents(self.overlayColor.CGColor));
CGContextFillRect (context, self.bounds);
CGContextRestoreGState(context);
}
通常,为了优化图形,您可以将实际图形限制为仅传递给drawRect的区域,但是由于每次更改颜色后都必须重新绘制背景图像,因此很有可能需要刷新整个图形。
要使用它,请创建对象的实例,然后将image属性(从UIImageView继承)设置为图片和overlayColorUIColor值(可以通过更改向下传递的颜色的alpha值来调整混合级别)。
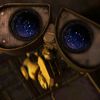
TA贡献2021条经验 获得超8个赞
在iOS7中,他们在UIImageView上引入了tintColor属性,在UIImage上引入了renderingMode。要在iOS7上着色UIImage,您要做的就是:
UIImageView* imageView = …
UIImage* originalImage = …
UIImage* imageForRendering = [originalImage imageWithRenderingMode:UIImageRenderingModeAlwaysTemplate];
imageView.image = imageForRendering;
imageView.tintColor = [UIColor redColor]; // or any color you want to tint it with
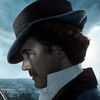
TA贡献1772条经验 获得超6个赞
我想用alpha着色图像,并创建了以下课程。如果您发现任何问题,请告诉我。
我已经命名了我的类,CSTintedImageView并且它继承UIView自类,因为UIImageView它没有调用该drawRect:方法,就像前面的答复中提到的那样。我已经设置了一个指定的初始化程序,该初始化程序类似于在UIImageView该类中找到的初始化程序。
用法:
CSTintedImageView * imageView = [[CSTintedImageView alloc] initWithImage:[UIImage imageNamed:@"image"]];
imageView.tintColor = [UIColor redColor];
CSTintedImageView.h
@interface CSTintedImageView : UIView
@property (strong, nonatomic) UIImage * image;
@property (strong, nonatomic) UIColor * tintColor;
- (id)initWithImage:(UIImage *)image;
@end
CSTintedImageView.m
#import "CSTintedImageView.h"
@implementation CSTintedImageView
@synthesize image=_image;
@synthesize tintColor=_tintColor;
- (id)initWithImage:(UIImage *)image
{
self = [super initWithFrame:CGRectMake(0, 0, image.size.width, image.size.height)];
if(self)
{
self.image = image;
//set the view to opaque
self.opaque = NO;
}
return self;
}
- (void)setTintColor:(UIColor *)color
{
_tintColor = color;
//update every time the tint color is set
[self setNeedsDisplay];
}
- (void)drawRect:(CGRect)rect
{
CGContextRef context = UIGraphicsGetCurrentContext();
//resolve CG/iOS coordinate mismatch
CGContextScaleCTM(context, 1, -1);
CGContextTranslateCTM(context, 0, -rect.size.height);
//set the clipping area to the image
CGContextClipToMask(context, rect, _image.CGImage);
//set the fill color
CGContextSetFillColor(context, CGColorGetComponents(_tintColor.CGColor));
CGContextFillRect(context, rect);
//blend mode overlay
CGContextSetBlendMode(context, kCGBlendModeOverlay);
//draw the image
CGContextDrawImage(context, rect, _image.CGImage);
}
@end
- 3 回答
- 0 关注
- 509 浏览
添加回答
举报