3 回答
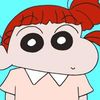
TA贡献1862条经验 获得超6个赞
Kris的解决方案确实很棒,但我发现工厂和流利的风格更好地融合在一起:
<?php
class Student
{
protected $firstName;
protected $lastName;
// etc.
/**
* Constructor
*/
public function __construct() {
// allocate your stuff
}
/**
* Static constructor / factory
*/
public static function create() {
$instance = new self();
return $instance;
}
/**
* FirstName setter - fluent style
*/
public function setFirstName( $firstName) {
$this->firstName = $firstName;
return $this;
}
/**
* LastName setter - fluent style
*/
public function setLastName( $lastName) {
$this->lastName = $lastName;
return $this;
}
}
// create instance
$student= Student::create()->setFirstName("John")->setLastName("Doe");
// see result
var_dump($student);
?>
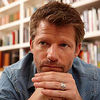
TA贡献1784条经验 获得超7个赞
我可能会做这样的事情:
<?php
class Student
{
public function __construct() {
// allocate your stuff
}
public static function withID( $id ) {
$instance = new self();
$instance->loadByID( $id );
return $instance;
}
public static function withRow( array $row ) {
$instance = new self();
$instance->fill( $row );
return $instance;
}
protected function loadByID( $id ) {
// do query
$row = my_awesome_db_access_stuff( $id );
$this->fill( $row );
}
protected function fill( array $row ) {
// fill all properties from array
}
}
?>
然后,如果我想要一个我知道ID的学生:
$student = Student::withID( $id );
或者,如果我有数据库行的数组:
$student = Student::withRow( $row );
从技术上讲,您不是在构建多个构造函数,而只是在构建静态辅助方法,而是通过这种方式避免在构造函数中使用大量意大利面条式代码。
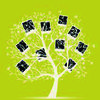
TA贡献1811条经验 获得超5个赞
PHP是一种动态语言,因此您不能重载方法。您必须像这样检查参数的类型:
class Student
{
protected $id;
protected $name;
// etc.
public function __construct($idOrRow){
if(is_int($idOrRow))
{
$this->id = $idOrRow;
// other members are still uninitialized
}
else if(is_array($idOrRow))
{
$this->id = $idOrRow->id;
$this->name = $idOrRow->name;
// etc.
}
}
- 3 回答
- 0 关注
- 788 浏览
添加回答
举报