3 回答
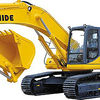
TA贡献1852条经验 获得超7个赞
如果您的printf支持该'标志(POSIX 2008要求printf()),则可以仅通过适当地设置区域设置来实现。例:
#include <stdio.h>
#include <locale.h>
int main(void)
{
setlocale(LC_NUMERIC, "");
printf("%'d\n", 1123456789);
return 0;
}
并运行:
$ ./example
1,123,456,789
在Mac OS X和Linux(Ubuntu 10.10)上进行了测试。
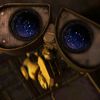
TA贡献2021条经验 获得超8个赞
您可以按以下方式递归执行此操作(请注意,INT_MIN如果使用二进制补码,则需要额外的代码来管理它):
void printfcomma2 (int n) {
if (n < 1000) {
printf ("%d", n);
return;
}
printfcomma2 (n/1000);
printf (",%03d", n%1000);
}
void printfcomma (int n) {
if (n < 0) {
printf ("-");
n = -n;
}
printfcomma2 (n);
}
总结:
用户printfcomma使用整数调用,负数的特殊情况是通过简单地打印“-”并使数字为正数来处理(这是不能使用的位INT_MIN)。
输入时printfcomma2,小于1,000的数字将被打印并返回。
否则,递归将在下一级上调用(因此将调用1,234,567,先以1,234,然后是1),直到找到小于1,000的数字。
然后将打印该数字,我们将返回递归树,在打印过程中打印逗号和下一个数字。
还有一个更简洁的版本,尽管它在检查每个级别的负数时进行了不必要的处理(这并不重要,因为递归级别的数量有限)。这是一个完整的测试程序:
#include <stdio.h>
void printfcomma (int n) {
if (n < 0) {
printf ("-");
printfcomma (-n);
return;
}
if (n < 1000) {
printf ("%d", n);
return;
}
printfcomma (n/1000);
printf (",%03d", n%1000);
}
int main (void) {
int x[] = {-1234567890, -123456, -12345, -1000, -999, -1,
0, 1, 999, 1000, 12345, 123456, 1234567890};
int *px = x;
while (px != &(x[sizeof(x)/sizeof(*x)])) {
printf ("%-15d: ", *px);
printfcomma (*px);
printf ("\n");
px++;
}
return 0;
}
输出为:
-1234567890 : -1,234,567,890
-123456 : -123,456
-12345 : -12,345
-1000 : -1,000
-999 : -999
-1 : -1
0 : 0
1 : 1
999 : 999
1000 : 1,000
12345 : 12,345
123456 : 123,456
1234567890 : 1,234,567,890
一个不信任递归的迭代解决方案(尽管递归的唯一问题往往是堆栈空间,这在这里不会成为问题,因为即使对于64位整数也只有几层深度):
void printfcomma (int n) {
int n2 = 0;
int scale = 1;
if (n < 0) {
printf ("-");
n = -n;
}
while (n >= 1000) {
n2 = n2 + scale * (n % 1000);
n /= 1000;
scale *= 1000;
}
printf ("%d", n);
while (scale != 1) {
scale /= 1000;
n = n2 / scale;
n2 = n2 % scale;
printf (",%03d", n);
}
}
这两个产生2,147,483,647的INT_MAX。
- 3 回答
- 0 关注
- 1012 浏览
添加回答
举报