3 回答
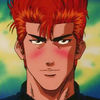
TA贡献1839条经验 获得超15个赞
以下是强类型列表的两个通用解决方案,它们将返回列表成员的所有唯一组合(如果您可以使用更简单的代码解决此问题,我向您致敬):
// Recursive
public static List<List<T>> GetAllCombos<T>(List<T> list)
{
List<List<T>> result = new List<List<T>>();
// head
result.Add(new List<T>());
result.Last().Add(list[0]);
if (list.Count == 1)
return result;
// tail
List<List<T>> tailCombos = GetAllCombos(list.Skip(1).ToList());
tailCombos.ForEach(combo =>
{
result.Add(new List<T>(combo));
combo.Add(list[0]);
result.Add(new List<T>(combo));
});
return result;
}
// Iterative, using 'i' as bitmask to choose each combo members
public static List<List<T>> GetAllCombos<T>(List<T> list)
{
int comboCount = (int) Math.Pow(2, list.Count) - 1;
List<List<T>> result = new List<List<T>>();
for (int i = 1; i < comboCount + 1; i++)
{
// make each combo here
result.Add(new List<T>());
for (int j = 0; j < list.Count; j++)
{
if ((i >> j) % 2 != 0)
result.Last().Add(list[j]);
}
}
return result;
}
// Example usage
List<List<int>> combos = GetAllCombos(new int[] { 1, 2, 3 }.ToList());
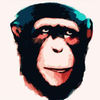
TA贡献1790条经验 获得超9个赞
这是使用递归的通用解决方案
public static ICollection<ICollection<T>> Permutations<T>(ICollection<T> list) {
var result = new List<ICollection<T>>();
if (list.Count == 1) { // If only one possible permutation
result.Add(list); // Add it and return it
return result;
}
foreach (var element in list) { // For each element in that list
var remainingList = new List<T>(list);
remainingList.Remove(element); // Get a list containing everything except of chosen element
foreach (var permutation in Permutations<T>(remainingList)) { // Get all possible sub-permutations
permutation.Add(element); // Add that element
result.Add(permutation);
}
}
return result;
}
我知道这是一篇旧帖子,但有人可能会觉得这很有帮助。
- 3 回答
- 0 关注
- 379 浏览
添加回答
举报