Python:如何并行运行python函数?我先研究过,找不到我的问题的答案。我试图在Python中并行运行多个函数。我有这样的事情:files.pyimport common #common is a util class that handles all the IO stuffdir1 = 'C:\folder1'dir2 = 'C:\folder2'filename = 'test.txt'addFiles = [25, 5, 15, 35, 45, 25, 5, 15, 35, 45]def func1():
c = common.Common()
for i in range(len(addFiles)):
c.createFiles(addFiles[i], filename, dir1)
c.getFiles(dir1)
time.sleep(10)
c.removeFiles(addFiles[i], dir1)
c.getFiles(dir1)def func2():
c = common.Common()
for i in range(len(addFiles)):
c.createFiles(addFiles[i], filename, dir2)
c.getFiles(dir2)
time.sleep(10)
c.removeFiles(addFiles[i], dir2)
c.getFiles(dir2)我想调用func1和func2并让它们同时运行。这些函数不会相互交互或在同一个对象上交互。现在我必须等待func1在func2启动之前完成。我如何做以下事情:process.pyfrom files import func1, func2
runBothFunc(func1(), func2())我希望能够创建非常接近同一时间的两个目录,因为每分钟我都在计算正在创建的文件数量。如果目录不在那里,它会甩掉我的时间。
3 回答
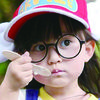
萧十郎
TA贡献1815条经验 获得超13个赞
你可以使用threading
或multiprocessing
。
由于CPython的特殊性,threading
不太可能实现真正的并行性。出于这个原因,multiprocessing
通常是一个更好的选择。
这是一个完整的例子:
from multiprocessing import Processdef func1(): print 'func1: starting' for i in xrange(10000000): pass print 'func1: finishing'def func2(): print 'func2: starting' for i in xrange(10000000): pass print 'func2: finishing'if __name__ == '__main__': p1 = Process(target=func1) p1.start() p2 = Process(target=func2) p2.start() p1.join() p2.join()
启动/加入子进程的机制可以很容易地封装到一个函数中runBothFunc
:
def runInParallel(*fns): proc = [] for fn in fns: p = Process(target=fn) p.start() proc.append(p) for p in proc: p.join()runInParallel(func1, func2)
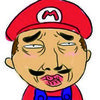
江户川乱折腾
TA贡献1851条经验 获得超5个赞
这可以通过Ray优雅地完成,这个系统允许您轻松地并行化和分发Python代码。
要并行化您的示例,您需要使用@ray.remote
装饰器定义您的函数,然后使用它来调用它们.remote
。
import ray ray.init()dir1 = 'C:\\folder1'dir2 = 'C:\\folder2'filename = 'test.txt'addFiles = [25, 5, 15, 35, 45, 25, 5, 15, 35, 45]# Define the functions. # You need to pass every global variable used by the function as an argument.# This is needed because each remote function runs in a different process,# and thus it does not have access to the global variables defined in # the current process.@ray.remotedef func1(filename, addFiles, dir): # func1() code here...@ray.remotedef func2(filename, addFiles, dir): # func2() code here...# Start two tasks in the background and wait for them to finish.ray.get([func1.remote(filename, addFiles, dir1), func2.remote(filename, addFiles, dir2)])
如果将相同的参数传递给两个函数并且参数很大,则更有效的方法是使用ray.put()
。这避免了大型参数被序列化两次并创建它的两个内存副本:
largeData_id = ray.put(largeData)ray.get([func1(largeData_id), func2(largeData_id)])
如果func1()
并func2()
返回结果,则需要重写代码,如下所示:
ret_id1 = func1.remote(filename, addFiles, dir1)ret_id2 = func1.remote(filename, addFiles, dir2)ret1, ret2 = ray.get([ret_id1, ret_id2])
在多处理模块上使用Ray有许多优点。特别是,相同的代码将在一台机器上运行,也可以在一组机器上运行。有关Ray的更多优点,请参阅此相关帖子。
添加回答
举报
0/150
提交
取消