如何在Java中按两个字段排序?我有一系列的对象person (int age; String name;).我如何按名称和年龄按字母顺序对这个数组进行排序?你会用哪种算法来解决这个问题?
3 回答
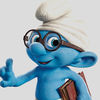
智慧大石
TA贡献1946条经验 获得超3个赞
.ThenBy(...)
.thenComparing(...)
Comparator<Person> comparator = Comparator.comparing(person -> person.name); comparator = comparator.thenComparing(Comparator.comparing(person -> person.age));
@Testpublic void testChainedSorting(){ // Create the collection of people: ArrayList<Person> people = new ArrayList<>(); people.add(new Person("Dan", 4)); people.add(new Person("Andi", 2)); people.add(new Person("Bob", 42)); people.add(new Person("Debby", 3)); people.add(new Person("Bob", 72)); people.add(new Person("Barry", 20)); people.add(new Person("Cathy", 40)); people.add(new Person("Bob", 40)); people.add(new Person("Barry", 50)); // Define chained comparators: // Great article explaining this and how to make it even neater: // http://blog.jooq.org/2014/01/31/java-8-friday-goodies-lambdas-and-sorting/ Comparator<Person> comparator = Comparator.comparing(person -> person.name); comparator = comparator.thenComparing(Comparator.comparing(person -> person.age)); // Sort the stream: Stream<Person> personStream = people.stream().sorted(comparator); // Make sure that the output is as expected: List<Person> sortedPeople = personStream.collect(Collectors.toList()); Assert.assertEquals("Andi", sortedPeople.get(0).name); Assert.assertEquals(2, sortedPeople.get(0).age); Assert.assertEquals("Barry", sortedPeople.get(1).name); Assert.assertEquals(20, sortedPeople.get(1).age); Assert.assertEquals("Barry", sortedPeople.get(2).name); Assert.assertEquals(50, sortedPeople.get(2).age); Assert.assertEquals("Bob", sortedPeople.get(3).name); Assert.assertEquals(40, sortedPeople.get(3).age); Assert.assertEquals("Bob", sortedPeople.get(4).name); Assert.assertEquals(42, sortedPeople.get(4).age); Assert.assertEquals("Bob", sortedPeople.get(5).name); Assert.assertEquals(72, sortedPeople.get(5).age); Assert.assertEquals("Cathy", sortedPeople.get(6).name); Assert.assertEquals(40, sortedPeople.get(6).age); Assert.assertEquals("Dan", sortedPeople.get(7).name); Assert.assertEquals(4, sortedPeople.get(7).age); Assert.assertEquals("Debby", sortedPeople.get(8).name); Assert.assertEquals(3, sortedPeople.get(8).age); // Andi : 2 // Barry : 20 // Barry : 50 // Bob : 40 // Bob : 42 // Bob : 72 // Cathy : 40 // Dan : 4 // Debby : 3}/** * A person in our system. */public static class Person{ /** * Creates a new person. * @param name The name of the person. * @param age The age of the person. */ public Person(String name, int age) { this.age = age; this.name = name; } /** * The name of the person. */ public String name; /** * The age of the person. */ public int age; @Override public String toString() { if (name == null) return super.toString(); else return String.format("%s : %d", this.name, this.age); }}
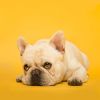
繁星coding
TA贡献1797条经验 获得超4个赞
//Creates and sorts a stream (does not sort the original list) persons.stream().sorted(Comparator.comparing(Person::getName).thenComparing(Person::getAge));
//Sorts the original list Lambda stylepersons.sort((p1, p2) -> { if (p1.getName().compareTo(p2.getName()) == 0) { return p1.getAge().compareTo(p2.getAge()); } else { return p1.getName().compareTo(p2.getName()); } });
//This is similar SYNTAX to the Streams above, but it sorts the original list!! persons.sort(Comparator.comparing(Person::getName).thenComparing(Person::getAge));
添加回答
举报
0/150
提交
取消