3 回答
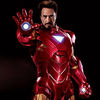
TA贡献2019条经验 获得超9个赞
这是一个解决方案,无需处理从一个textField到另一个textField的切换:
override func viewDidLoad() { super.viewDidLoad() NSNotificationCenter.defaultCenter().addObserver(self, selector: Selector("keyboardWillShow:"), name: UIKeyboardWillShowNotification, object: nil) NSNotificationCenter.defaultCenter().addObserver(self, selector: Selector("keyboardWillHide:"), name: UIKeyboardWillHideNotification, object: nil) } func keyboardWillShow(notification: NSNotification) { if let keyboardSize = (notification.userInfo?[UIKeyboardFrameBeginUserInfoKey] as? NSValue)?.CGRectValue() { self.view.frame.origin.y -= keyboardSize.height } }func keyboardWillHide(notification: NSNotification) { self.view.frame.origin.y = 0}
要解决此问题,请keyboardWillShow/Hide
使用以下代码替换这两个函数:
func keyboardWillShow(notification: NSNotification) { if let keyboardSize = (notification.userInfo?[UIKeyboardFrameBeginUserInfoKey] as? NSValue)?.CGRectValue() { if view.frame.origin.y == 0 { self.view.frame.origin.y -= keyboardSize.height } } }func keyboardWillHide(notification: NSNotification) { if view.frame.origin.y != 0 { self.view.frame.origin.y = 0 }}
编辑SWIFT 3.0:
override func viewDidLoad() { super.viewDidLoad() NotificationCenter.default.addObserver(self, selector: #selector(ViewController.keyboardWillShow), name: NSNotification.Name.UIKeyboardWillShow, object: nil) NotificationCenter.default.addObserver(self, selector: #selector(ViewController.keyboardWillHide), name: NSNotification.Name.UIKeyboardWillHide, object: nil) }@objc func keyboardWillShow(notification: NSNotification) { if let keyboardSize = (notification.userInfo?[UIKeyboardFrameBeginUserInfoKey] as? NSValue)?.cgRectValue { if self.view.frame.origin.y == 0 { self.view.frame.origin.y -= keyboardSize.height } } }@objc func keyboardWillHide(notification: NSNotification) { if self.view.frame.origin.y != 0 { self.view.frame.origin.y = 0 }}
编辑SWIFT 4.0:
override func viewDidLoad() { super.viewDidLoad() NotificationCenter.default.addObserver(self, selector: #selector(ViewController.keyboardWillShow), name: NSNotification.Name.UIKeyboardWillShow, object: nil) NotificationCenter.default.addObserver(self, selector: #selector(ViewController.keyboardWillHide), name: NSNotification.Name.UIKeyboardWillHide, object: nil) }@objc func keyboardWillShow(notification: NSNotification) { if let keyboardSize = (notification.userInfo?[UIKeyboardFrameBeginUserInfoKey] as? NSValue)?.cgRectValue { if self.view.frame.origin.y == 0 { self.view.frame.origin.y -= keyboardSize.height } } }@objc func keyboardWillHide(notification: NSNotification) { if self.view.frame.origin.y != 0 { self.view.frame.origin.y = 0 }}
编辑SWIFT 4.2:
override func viewDidLoad() { super.viewDidLoad() NotificationCenter.default.addObserver(self, selector: #selector(keyboardWillShow), name: UIResponder.keyboardWillShowNotification, object: nil) NotificationCenter.default.addObserver(self, selector: #selector(keyboardWillHide), name: UIResponder.keyboardWillHideNotification, object: nil)}@objc func keyboardWillShow(notification: NSNotification) { if let keyboardSize = (notification.userInfo?[UIResponder.keyboardFrameBeginUserInfoKey] as? NSValue)?.cgRectValue { if self.view.frame.origin.y == 0 { self.view.frame.origin.y -= keyboardSize.height } }}@objc func keyboardWillHide(notification: NSNotification) { if self.view.frame.origin.y != 0 { self.view.frame.origin.y = 0 }}
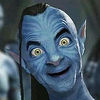
TA贡献1843条经验 获得超7个赞
最简单的方法,甚至不需要任何代码:
如果您还没有使用Spring动画框架,请下载KeyboardLayoutConstraint.swift并将文件添加(拖放)到您的项目中。
在故事板中,为视图或文本字段创建底部约束,选择约束(双击它),然后在Identity Inspector中,将其类从NSLayoutConstraint更改为KeyboardLayoutConstraint。
完成!
该对象将与键盘同步自动向上移动。
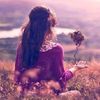
TA贡献1815条经验 获得超10个赞
此线程上的一个流行答案使用以下代码:
func keyboardWillShow(sender: NSNotification) { self.view.frame.origin.y -= 150}func keyboardWillHide(sender: NSNotification) { self.view.frame.origin.y += 150}
将静态量偏移视图存在明显问题。它在一台设备上看起来不错,但在任何其他尺寸配置上看起来都很糟糕。您需要获得键盘高度并将其用作偏移值。
这是一个适用于所有设备的解决方案,可以处理用户在键入时隐藏预测文本字段的边缘情况。
解
需要注意的是,我们将self.view.window作为对象参数传递。这将为我们提供键盘数据,例如它的高度!
@IBOutlet weak var messageField: UITextField!override func viewDidLoad() { super.viewDidLoad() NSNotificationCenter.defaultCenter().addObserver(self, selector: Selector("keyboardWillShow:"), name:UIKeyboardWillShowNotification, object: self.view.window) NSNotificationCenter.defaultCenter().addObserver(self, selector: Selector("keyboardWillHide:"), name:UIKeyboardWillHideNotification, object: self.view.window)}func keyboardWillHide(sender: NSNotification) { let userInfo: [NSObject : AnyObject] = sender.userInfo! let keyboardSize: CGSize = userInfo[UIKeyboardFrameBeginUserInfoKey]!.CGRectValue.size self.view.frame.origin.y += keyboardSize.height}
我们将使它在所有设备上看起来都很漂亮,并处理用户添加或删除预测文本字段的情况。
func keyboardWillShow(sender: NSNotification) { let userInfo: [NSObject : AnyObject] = sender.userInfo! let keyboardSize: CGSize = userInfo[UIKeyboardFrameBeginUserInfoKey]!.CGRectValue.size let offset: CGSize = userInfo[UIKeyboardFrameEndUserInfoKey]!.CGRectValue.size if keyboardSize.height == offset.height { UIView.animateWithDuration(0.1, animations: { () -> Void in self.view.frame.origin.y -= keyboardSize.height }) } else { UIView.animateWithDuration(0.1, animations: { () -> Void in self.view.frame.origin.y += keyboardSize.height - offset.height }) }}
删除观察员
在离开视图之前不要忘记删除观察者,以防止传输不必要的消息。
override func viewWillDisappear(animated: Bool) { NSNotificationCenter.defaultCenter().removeObserver(self, name: UIKeyboardWillShowNotification, object: self.view.window) NSNotificationCenter.defaultCenter().removeObserver(self, name: UIKeyboardWillHideNotification, object: self.view.window)}
根据评论中的问题进行更新:
如果您有两个或更多文本字段,则可以检查view.frame.origin.y是否为零。
func keyboardWillShow(sender: NSNotification) { let userInfo: [NSObject : AnyObject] = sender.userInfo! let keyboardSize: CGSize = userInfo[UIKeyboardFrameBeginUserInfoKey]!.CGRectValue.size let offset: CGSize = userInfo[UIKeyboardFrameEndUserInfoKey]!.CGRectValue.size if keyboardSize.height == offset.height { if self.view.frame.origin.y == 0 { UIView.animateWithDuration(0.1, animations: { () -> Void in self.view.frame.origin.y -= keyboardSize.height }) } } else { UIView.animateWithDuration(0.1, animations: { () -> Void in self.view.frame.origin.y += keyboardSize.height - offset.height }) } print(self.view.frame.origin.y)}
- 3 回答
- 0 关注
- 802 浏览
添加回答
举报