使用volley发送带有JSON数据的POST请求我想寄一个新的JsonObjectRequest请求:我想接收JSON数据(来自服务器的响应):好的我想将带有此请求的JSON格式化数据发送到服务器JsonObjectRequest request = new JsonObjectRequest(
Request.Method.POST, "myurl.com", null,
new Response.Listener<JSONObject>() {
@Override
public void onResponse(JSONObject response) {
//...
}
},
new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
//...
}
})
{
@Override
protected Map<String,String> getParams() {
// something to do here ??
return params;
}
@Override
public Map<String, String> getHeaders() throws AuthFailureError {
// something to do here ??
return params;
}
};我在我的项目中也使用了gson库。
3 回答
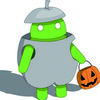
临摹微笑
TA贡献1982条经验 获得超2个赞
JsonObjectRequest
JSONObject
final String url = "some/url";final JSONObject jsonBody = new JSONObject("{\"type\":\"example\"}");new JsonObjectRequest(url, jsonBody, new Response.Listener<JSONObject>() { ... });
@param jsonRequest
):
/** * Creates a new request. * @param method the HTTP method to use * @param url URL to fetch the JSON from * @param jsonRequest A {@link JSONObject} to post with the request. Null is allowed and * indicates no parameters will be posted along with request. * @param listener Listener to receive the JSON response * @param errorListener Error listener, or null to ignore errors. */public JsonObjectRequest(int method, String url, JSONObject jsonRequest, Listener<JSONObject> listener, ErrorListener errorListener) { super(method, url, (jsonRequest == null) ? null : jsonRequest.toString(), listener, errorListener);}
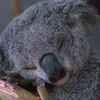
www说
TA贡献1775条经验 获得超8个赞
这,这个 JSONObjectRequest
并不完美:你必须期待 JSON
最后(见 Response.Listener<JSONObject>
).空响应(仅为200状态)怎么办? 如果我想直接从 ResponseListener
?
/** * Created by laurentmeyer on 25/07/15. */ public class GenericRequest<T> extends JsonRequest<T> { private final Gson gson = new Gson(); private final Class<T> clazz; private final Map<String, String> headers; // Used for request which do not return anything from the server private boolean muteRequest = false; /** * Basically, this is the constructor which is called by the others. * It allows you to send an object of type A to the server and expect a JSON representing a object of type B. * The problem with the #JsonObjectRequest is that you expect a JSON at the end. * We can do better than that, we can directly receive our POJO. * That's what this class does. * * @param method: HTTP Method * @param classtype: Classtype to parse the JSON coming from the server * @param url: url to be called * @param requestBody: The body being sent * @param listener: Listener of the request * @param errorListener: Error handler of the request * @param headers: Added headers */ private GenericRequest(int method, Class<T> classtype, String url, String requestBody, Response.Listener<T> listener, Response.ErrorListener errorListener, Map<String, String> headers) { super(method, url, requestBody, listener, errorListener); clazz = classtype; this.headers = headers; configureRequest(); } /** * Method to be called if you want to send some objects to your server via body in JSON of the request (with headers and not muted) * * @param method: HTTP Method * @param url: URL to be called * @param classtype: Classtype to parse the JSON returned from the server * @param toBeSent: Object which will be transformed in JSON via Gson and sent to the server * @param listener: Listener of the request * @param errorListener: Error handler of the request * @param headers: Added headers */ public GenericRequest(int method, String url, Class<T> classtype, Object toBeSent, Response.Listener<T> listener, Response.ErrorListener errorListener, Map<String, String> headers) { this(method, classtype, url, new Gson().toJson(toBeSent), listener, errorListener, headers); } /** * Method to be called if you want to send some objects to your server via body in JSON of the request (without header and not muted) * * @param method: HTTP Method * @param url: URL to be called * @param classtype: Classtype to parse the JSON returned from the server * @param toBeSent: Object which will be transformed in JSON via Gson and sent to the server * @param listener: Listener of the request * @param errorListener: Error handler of the request */ public GenericRequest(int method, String url, Class<T> classtype, Object toBeSent, Response.Listener<T> listener, Response.ErrorListener errorListener) { this(method, classtype, url, new Gson().toJson(toBeSent), listener, errorListener, new HashMap<String, String>()); } /** * Method to be called if you want to send something to the server but not with a JSON, just with a defined String (without header and not muted) * * @param method: HTTP Method * @param url: URL to be called * @param classtype: Classtype to parse the JSON returned from the server * @param requestBody: String to be sent to the server * @param listener: Listener of the request * @param errorListener: Error handler of the request */ public GenericRequest(int method, String url, Class<T> classtype, String requestBody, Response.Listener<T> listener, Response.ErrorListener errorListener) { this(method, classtype, url, requestBody, listener, errorListener, new HashMap<String, String>()); } /** * Method to be called if you want to GET something from the server and receive the POJO directly after the call (no JSON). (Without header) * * @param url: URL to be called * @param classtype: Classtype to parse the JSON returned from the server * @param listener: Listener of the request * @param errorListener: Error handler of the request */ public GenericRequest(String url, Class<T> classtype, Response.Listener<T> listener, Response.ErrorListener errorListener) { this(Request.Method.GET, url, classtype, "", listener, errorListener); } /** * Method to be called if you want to GET something from the server and receive the POJO directly after the call (no JSON). (With headers) * * @param url: URL to be called * @param classtype: Classtype to parse the JSON returned from the server * @param listener: Listener of the request * @param errorListener: Error handler of the request * @param headers: Added headers */ public GenericRequest(String url, Class<T> classtype, Response.Listener<T> listener, Response.ErrorListener errorListener, Map<String, String> headers) { this(Request.Method.GET, classtype, url, "", listener, errorListener, headers); } /** * Method to be called if you want to send some objects to your server via body in JSON of the request (with headers and muted) * * @param method: HTTP Method * @param url: URL to be called * @param classtype: Classtype to parse the JSON returned from the server * @param toBeSent: Object which will be transformed in JSON via Gson and sent to the server * @param listener: Listener of the request * @param errorListener: Error handler of the request * @param headers: Added headers * @param mute: Muted (put it to true, to make sense) */ public GenericRequest(int method, String url, Class<T> classtype, Object toBeSent, Response.Listener<T> listener, Response.ErrorListener errorListener, Map<String, String> headers, boolean mute) { this(method, classtype, url, new Gson().toJson(toBeSent), listener, errorListener, headers); this.muteRequest = mute; } /** * Method to be called if you want to send some objects to your server via body in JSON of the request (without header and muted) * * @param method: HTTP Method * @param url: URL to be called * @param classtype: Classtype to parse the JSON returned from the server * @param toBeSent: Object which will be transformed in JSON via Gson and sent to the server * @param listener: Listener of the request * @param errorListener: Error handler of the request * @param mute: Muted (put it to true, to make sense) */ public GenericRequest(int method, String url, Class<T> classtype, Object toBeSent, Response.Listener<T> listener, Response.ErrorListener errorListener, boolean mute) { this(method, classtype, url, new Gson().toJson(toBeSent), listener, errorListener, new HashMap<String, String>()); this.muteRequest = mute; } /** * Method to be called if you want to send something to the server but not with a JSON, just with a defined String (without header and not muted) * * @param method: HTTP Method * @param url: URL to be called * @param classtype: Classtype to parse the JSON returned from the server * @param requestBody: String to be sent to the server * @param listener: Listener of the request * @param errorListener: Error handler of the request * @param mute: Muted (put it to true, to make sense) */ public GenericRequest(int method, String url, Class<T> classtype, String requestBody, Response.Listener<T> listener, Response.ErrorListener errorListener, boolean mute) { this(method, classtype, url, requestBody, listener, errorListener, new HashMap<String, String>()); this.muteRequest = mute; } @Override protected Response<T> parseNetworkResponse(NetworkResponse response) { // The magic of the mute request happens here if (muteRequest) { if (response.statusCode >= 200 && response.statusCode <= 299) { // If the status is correct, we return a success but with a null object, because the server didn't return anything return Response.success(null, HttpHeaderParser.parseCacheHeaders(response)); } } else { try { // If it's not muted; we just need to create our POJO from the returned JSON and handle correctly the errors String json = new String(response.data, HttpHeaderParser.parseCharset(response.headers)); T parsedObject = gson.fromJson(json, clazz); return Response.success(parsedObject, HttpHeaderParser.parseCacheHeaders(response)); } catch (UnsupportedEncodingException e) { return Response.error(new ParseError(e)); } catch (JsonSyntaxException e) { return Response.error(new ParseError(e)); } } return null; } @Override public Map<String, String> getHeaders() throws AuthFailureError { return headers != null ? headers : super.getHeaders(); } private void configureRequest() { // Set retry policy // Add headers, for auth for example // ... } }
解析为POJO/Header的请求手动设置/POJO发送 解析为POJO/POJO以发送响应的请求 解析为POJO/String以发送响应的请求 解析为POJO(GET)的响应的请求 将响应解析为POJO(GET)/Header手动设置的请求 没有响应的请求(200-空体)/头手动设置/POJO发送 没有响应的请求(200-空体)/POJO发送 没有响应的请求(200-空体)/字符串发送
compile 'com.google.code.gson:gson:x.y.z'
2.3.1
).
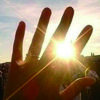
冉冉说
TA贡献1877条经验 获得超1个赞
final String URL = "/volley/resource/12";// Post params to be sent to the serverHashMap<String, String> params = new HashMap<String, String>();params.put("token", "AbCdEfGh123456");JsonObjectRequest req = new JsonObjectRequest(URL, new JSONObject(params), new Response.Listener<JSONObject>() { @Override public void onResponse(JSONObject response) { try { VolleyLog.v("Response:%n %s", response.toString(4)); } catch (JSONException e) { e.printStackTrace(); } } }, new Response.ErrorListener() { @Override public void onErrorResponse(VolleyError error) { VolleyLog.e("Error: ", error.getMessage()); } });// add the request object to the queue to be executedApplicationController.getInstance().addToRequestQueue(req);
- 3 回答
- 0 关注
- 715 浏览
添加回答
举报
0/150
提交
取消