1 回答
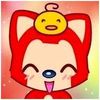
TA贡献1858条经验 获得超8个赞
允许JVM假定其他线程pizzaArrived
在循环期间不更改变量。换句话说,它可以pizzaArrived == false
在循环外提升测试,优化:
while (pizzaArrived == false) {}
进入这个:
if (pizzaArrived == false) while (true) {}
这是一个无限循环。
要确保一个线程所做的更改对其他线程可见,您必须始终在线程之间添加一些同步。最简单的方法是创建共享变量volatile
:
volatile boolean pizzaArrived = false;
创建变量可以volatile
保证不同的线程可以看到彼此对其的更改的影响。这可以防止JVM缓存pizzaArrived
循环外的测试值或将测试挂起。相反,它必须每次都读取实变量的值。
(更正式地说,在访问变量之间volatile
创建了一个先发生的关系。这意味着在传递披萨之前线程所做的所有其他工作对于接收披萨的线程也是可见的,即使那些其他更改不是volatile
变量。)
同步方法主要用于实现互斥(防止两件事同时发生),但它们也具有所有相同的副作用volatile
。在读取和写入变量时使用它们是另一种使更改对其他线程可见的方法:
class MyHouse { boolean pizzaArrived = false; void eatPizza() { while (getPizzaArrived() == false) {} System.out.println("That was delicious!"); } synchronized boolean getPizzaArrived() { return pizzaArrived; } synchronized void deliverPizza() { pizzaArrived = true; }}
打印声明的效果
System.out
是一个PrintStream
对象。方法是PrintStream
这样同步的:
public void println(String x) { synchronized (this) { print(x); newLine(); }}
同步防止pizzaArrived
在循环期间缓存。严格地说,两个线程必须在同一个对象上同步,以保证对变量的更改是可见的。(例如,println
在设置之后pizzaArrived
调用并在读取之前再次调用它将pizzaArrived
是正确的。)如果只有一个线程在特定对象上同步,则允许JVM忽略它。在实践中,JVM不够智能,无法证明其他线程println
在设置后不会调用pizzaArrived
,因此它假定它们可能。因此,如果调用它,它不能在循环期间缓存变量System.out.println
。这就是为什么这样的循环在有打印语句时起作用的原因,尽管它不是正确的解决方法。
使用System.out
不是导致这种效果的唯一方法,但它是人们最常发现的,当他们试图调试为什么他们的循环不起作用时!
更大的问题
while (pizzaArrived == false) {}
是一个忙碌的等待循环。那很糟!当它等待时,它会占用CPU,从而减慢其他应用程序的速度,并增加系统的功耗,温度和风扇速度。理想情况下,我们希望循环线程在等待时休眠,因此它不会占用CPU。
以下是一些方法:
使用wait / notify
一个低级解决方案是使用以下的wait / notify方法Object
:
class MyHouse { boolean pizzaArrived = false; void eatPizza() { synchronized (this) { while (!pizzaArrived) { try { this.wait(); } catch (InterruptedException e) {} } } System.out.println("That was delicious!"); } void deliverPizza() { synchronized (this) { pizzaArrived = true; this.notifyAll(); } }}
在这个版本的代码中,循环线程调用wait()
,这使线程处于休眠状态。睡觉时不会使用任何CPU周期。在第二个线程设置变量之后,它调用notifyAll()
唤醒正在等待该对象的任何/所有线程。这就像披萨家伙敲响了门铃一样,所以你可以坐下来休息,等待,而不是笨拙地站在门口。
在对象上调用wait / notify时,必须保持该对象的同步锁,这就是上面的代码所做的。你可以使用你喜欢的任何对象,只要两个线程使用相同的对象:这里我使用this
(实例MyHouse
)。通常,两个线程无法同时进入同一对象的同步块(这是同步目的的一部分),但它在此处起作用,因为线程在wait()
方法内部时临时释放同步锁。
BlockingQueue的
A BlockingQueue
用于实现生产者 - 消费者队列。“消费者”从队列前面取物品,“生产者”在后面推动物品。一个例子:
class MyHouse { final BlockingQueue<Object> queue = new LinkedBlockingQueue<>(); void eatFood() throws InterruptedException { // take next item from the queue (sleeps while waiting) Object food = queue.take(); // and do something with it System.out.println("Eating: " + food); } void deliverPizza() throws InterruptedException { // in producer threads, we push items on to the queue. // if there is space in the queue we can return immediately; // the consumer thread(s) will get to it later queue.put("A delicious pizza"); }}
注意:可以抛出s 的put
和take
方法,它们是必须处理的已检查异常。在上面的代码中,为简单起见,重新抛出了异常。您可能更喜欢捕获方法中的异常并重试put或take调用以确保它成功。除了那一点丑陋之外,很容易使用。BlockingQueue
InterruptedException
BlockingQueue
这里不需要其他同步,因为BlockingQueue
确保在将项目放入队列之前所做的所有线程对于将这些项目取出的线程是可见的。
执行人
Executor
s就像现成的BlockingQueue
执行任务一样。例:
// A "SingleThreadExecutor" has one work thread and an unlimited queueExecutorService executor = Executors.newSingleThreadExecutor(); Runnable eatPizza = () -> { System.out.println("Eating a delicious pizza"); };Runnable cleanUp = () -> { System.out.println("Cleaning up the house"); }; // we submit tasks which will be executed on the work threadexecutor.execute(eatPizza);executor.execute(cleanUp); // we continue immediately without needing to wait for the tasks to finish
有关详情请参阅该文档Executor
,ExecutorService
和Executors
。
事件处理
在等待用户在UI中单击某些内容时循环是错误的。而是使用UI工具包的事件处理功能。例如,在Swing中:
JLabel label = new JLabel();JButton button = new JButton("Click me");button.addActionListener((ActionEvent e) -> { // This event listener is run when the button is clicked. // We don't need to loop while waiting. label.setText("Button was clicked");});
因为事件处理程序在事件派发线程上运行,所以在事件处理程序中执行长时间的工作会阻止与UI的其他交互,直到工作完成。可以在新线程上启动慢速操作,或使用上述技术之一(wait / notify,a BlockingQueue
或Executor
)将调度分派给等待的线程。您还可以使用SwingWorker
专为此设计的a,并自动提供后台工作线程:
JLabel label = new JLabel();JButton button = new JButton("Calculate answer");// Add a click listener for the buttonbutton. addActionListener((ActionEvent e) -> { // Defines MyWorker as a SwingWorker whose result type is String: class MyWorker extends SwingWorker<String,Void> { @Override public String doInBackground() throws Exception { // This method is called on a background thread. // You can do long work here without blocking the UI. // This is just an example: Thread.sleep(5000); return "Answer is 42"; } @Override protected void done() { // This method is called on the Swing thread once the work is done String result; try { result = get(); } catch (Exception e) { throw new RuntimeException(e); } label.setText(result); // will display "Answer is 42" } } // Start the worker new MyWorker().execute();});
计时器
要执行定期操作,您可以使用a java.util.Timer
。它比编写自己的定时循环更容易使用,更容易启动和停止。该演示每秒打印一次当前时间:
Timer timer = new Timer();TimerTask task = new TimerTask() { @Override public void run() { System.out.println(System.currentTimeMillis()); }};timer.scheduleAtFixedRate(task, 0, 1000);
每个java.util.Timer
都有自己的后台线程,用于执行其调度的TimerTask
s。当然,线程在任务之间休眠,因此它不会占用CPU。
在Swing代码中,还有一个javax.swing.Timer
类似的,但它在Swing线程上执行侦听器,因此您可以安全地与Swing组件交互,而无需手动切换线程:
JFrame frame = new JFrame();frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);Timer timer = new Timer(1000, (ActionEvent e) -> { frame.setTitle(String.valueOf(System.currentTimeMillis()));});timer.setRepeats(true);timer.start();frame.setVisible(true);
其他方法
如果您正在编写多线程代码,那么值得探索这些包中的类以查看可用的内容:
另请参阅Java教程的Concurrency部分。多线程很复杂,但有很多帮助可用!
添加回答
举报