import java.util.ArrayList;import java.util.Comparator;import java.util.List;import java.util.Optional;public class GetMiles {
public static void main(String args[]) {
List<Student> studentList = new ArrayList<>();
Student s = new Student();
s.setFee("12000");
studentList.add(s);
Student s1 = new Student();
s1.setFee("3000");
studentList.add(s1);
Optional<Student> optionalStudent =
studentList.stream().min(Comparator.comparing(Student::getFee));
if (optionalStudent.isPresent()) {
System.out.println(optionalStudent.get().getFee());
}
}static class Student {
private String fee;
public String getFee() {
return this.fee;
}
public void setFee(String fee) {
this.fee = fee;
}
}
}在上面的例子中它应该返回3000但是返回12000如果我们将给2000和3000它将返回2000也在大多数情况下它的工作正常但不是全部。
5 回答
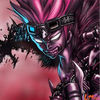
哈士奇WWW
TA贡献1799条经验 获得超6个赞
将映射解析为列表中的int,然后像下面的示例代码一样获得最小费用:
Optional<Integer> optionalVal = studentList.stream().map(l -> Integer.parseInt(l.getFee())).min(Comparator.comparingInt(k -> k)); if(optionalVal.isPresent()) {String minFee = String.valueOf(optionalVal.get()); Optional<Student> studentObj = studentList.stream().filter(p -> minFee.equals(p.getFee())).findFirst();}
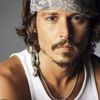
慕哥9229398
TA贡献1877条经验 获得超6个赞
那是因为你使用的是String,但正如你所指定的那样,这是一个要求。所以你必须以这种方式改变流:
OptionalInt min = studentList.stream() .map(Student::getFee) .mapToInt(Integer::parseInt) .min();
通过这种方式,您将String转换为Int,然后您将获取最小值。
如果您的值有小数,请mapToDouble
改用
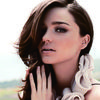
慕莱坞森
TA贡献1810条经验 获得超4个赞
你正在比较String
而不是Integer
。
您可以通过提供一个解决这个问题Comparator
是解析String
到一个Integer
(或者double
,如果你喜欢):
Optional<Student> opt = studentList .stream() .min(Comparator.comparing(stud -> Integer.parseInt(stud.getFee())));
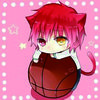
小怪兽爱吃肉
TA贡献1852条经验 获得超1个赞
您正在比较String
值,您应该比较数值以获得预期结果,如doubles
或ints
。改变你的类型fee
字段Double
,Long
或Integer
。
比较字符串逐个字母,所以比较3000
和12000
使3000
显得更大,因为第一个字母比较3
> 1
。
添加回答
举报
0/150
提交
取消