直接贴代码了public class SftpUtil {
private static Session session = null;
private static Channel channel = null;
private static final Logger log = Logger.getLogger(SftpUtil.class.getName());
/**
* 获取ChannelSftp实例对象
*
* @param sftpDetails
* @param timeout
* @return ChannelSftp
* @throws JSchException
*/
private static ChannelSftp getChannel(int timeout) throws JSchException {
ResourceBundle bundle = ResourceBundle.getBundle("ZJW");
String ftpHost = bundle.getString(SftpConstants.SFTP_REQ_HOST);
String port = bundle.getString(SftpConstants.SFTP_REQ_PORT);
String ftpUsername = bundle.getString(SftpConstants.SFTP_REQ_USERNAME);
String ftpPassword = bundle.getString(SftpConstants.SFTP_REQ_PASSWORD);
int ftpPort = SftpConstants.SFTP_DEFAULT_PORT;
if (port != null && !port.equals("")) {
ftpPort = Integer.valueOf(port);
}
JSch jsch = new JSch(); // 创建JSch对象
session = jsch.getSession(ftpUsername, ftpHost, ftpPort); // 根据用户名,主机ip,端口获取一个Session对象
System.out.println(ftpUsername+"###"+ftpHost+"###"+ftpPort+"###"+ftpPassword);
log.info("Session created.");
if (ftpPassword != null) {
session.setPassword(ftpPassword); // 设置密码
}
Properties config = new Properties();
config.put("StrictHostKeyChecking", "no");
//config.put("PreferredAuthentications", "password");
session.setConfig(config); // 为Session对象设置properties
session.setTimeout(timeout); // 设置timeout时间
log.info("Session建立连接。。。");
session.connect(); // 通过Session建立链接
log.info("Session connected.");
log.info("Opening Channel.");
channel = session.openChannel("sftp"); // 打开SFTP通道
channel.connect(); // 建立SFTP通道的连接
log.info("Connected successfully to ftpHost = " + ftpHost + ",as ftpUsername = " + ftpUsername
+ ", returning: " + channel);
return (ChannelSftp) channel;
}
/**
* zip文件上传
* @param dst
* @param src
* @param timeout
* @description dst为目标文件名(e.g:xxx/sftp/xxx.zip")
* src为本地文件名(e.g:c:\\xxx\\xxx.zip)
* timeout为连接超时时间,单位ms
*/
public static void uploadZip(String dst,String src,int timeout){
try {
ChannelSftp channel = getChannel(timeout); //获取执行对象
if(channel==null){
log.info("程序错误,ChannelSftp获取为null!");
}
log.info("zip上传开始...");
channel.put(src, dst, ChannelSftp.OVERWRITE); //执行sftp上传,模式为overwrite
log.info("zip上传成功...");
} catch (Exception e) {
log.info("系统异常,文件上传失败!");
e.printStackTrace();
}finally {
try {
closeChannel();
} catch (Exception e) {
log.info("系统异常,资源关闭失败!");
e.printStackTrace();
}
}
}
}
在本机装了一个msftpsrvr64模拟sftp,使用FlashFXP连接上传都很正常用自己写的测试就不行了
@Test
public void demo03(){
String dst = "\0000.zip";
String src = "D:\\0000.zip";
SftpUtil.uploadZip(dst, src, 6000);
}
错误码:
java.lang.StringIndexOutOfBoundsException: String index out of range: 16777219
at java.lang.String.checkBounds(Unknown Source)
at java.lang.String.<init>(Unknown Source)
at com.jcraft.jsch.Util.byte2str(Util.java:417)
at com.jcraft.jsch.Util.byte2str(Util.java:428)
at com.jcraft.jsch.DHG14.next(DHG14.java:203)
at com.jcraft.jsch.Session.connect(Session.java:326)
at com.jcraft.jsch.Session.connect(Session.java:183)
at com.waterelephant.zjw.utils.SftpUtil.getChannel(SftpUtil.java:67)
at com.waterelephant.zjw.utils.SftpUtil.uploadZip(SftpUtil.java:102)
at com.waterelephant.test.PropTest.demo03(PropTest.java:23)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source)
at java.lang.reflect.Method.invoke(Unknown Source)
at org.junit.runners.model.FrameworkMethod$1.runReflectiveCall(FrameworkMethod.java:50)
at org.junit.internal.runners.model.ReflectiveCallable.run(ReflectiveCallable.java:12)
at org.junit.runners.model.FrameworkMethod.invokeExplosively(FrameworkMethod.java:47)
at org.junit.internal.runners.statements.InvokeMethod.evaluate(InvokeMethod.java:17)
at org.junit.runners.ParentRunner.runLeaf(ParentRunner.java:325)
at org.junit.runners.BlockJUnit4ClassRunner.runChild(BlockJUnit4ClassRunner.java:78)
at org.junit.runners.BlockJUnit4ClassRunner.runChild(BlockJUnit4ClassRunner.java:57)
at org.junit.runners.ParentRunner$3.run(ParentRunner.java:290)
at org.junit.runners.ParentRunner$1.schedule(ParentRunner.java:71)
at org.junit.runners.ParentRunner.runChildren(ParentRunner.java:288)
at org.junit.runners.ParentRunner.access$000(ParentRunner.java:58)
at org.junit.runners.ParentRunner$2.evaluate(ParentRunner.java:268)
at org.junit.runners.ParentRunner.run(ParentRunner.java:363)
at org.eclipse.jdt.internal.junit4.runner.JUnit4TestReference.run(JUnit4TestReference.java:86)
at org.eclipse.jdt.internal.junit.runner.TestExecution.run(TestExecution.java:38)
at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.runTests(RemoteTestRunner.java:459)
at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.runTests(RemoteTestRunner.java:678)
at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.run(RemoteTestRunner.java:382)
at org.eclipse.jdt.internal.junit.runner.RemoteTestRunner.main(RemoteTestRunner.java:192)
调试过 是在session.connect()那里出的异常 debug进去看了一下 是连上了的 但是中间出了异常 没看太懂...
1 回答
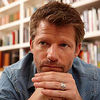
噜噜哒
TA贡献1784条经验 获得超7个赞
是msftpsrvr64这个软件的问题 换了一个freeSSHD模拟sftp服务器就好了
连接成功之后上传文件的时候又报了如下异常:
3: Permission denied
问题原因:服务器的根目录默认C盘
解决:修改服务器目录到D:XXX 即可解决问题
添加回答
举报
0/150
提交
取消