1 package per.swwfourteen.fourteen;
2
3 public class Student {
4 private String id;
5
6 private String name;
7
8 private String password;
9
10 private String age;
11
12 public void setId(String id) {
13 this.id = id;
14 }
15
16 public String getId() {
17 return this.id;
18 }
19
20 public void setName(String name) {
21 this.name = name;
22 }
23
24 public String getName() {
25 return this.name;
26 }
27
28 public void setPassword(String password) {
29 this.password = password;
30 }
31
32 public String getPassword() {
33 return this.password;
34 }
35
36 public void setAge(String age) {
37 this.age = age;
38 }
39
40 public String getAge() {
41 return this.age;
42 }
43
44 }
1 package per.swwfourteen.fourteen;
2
3 public class Teacher {
4 private String id;
5
6 private String name;
7
8 private String password;
9
10 private String age;
11
12 private Car car;
13
14 public void setId(String id) {
15 this.id = id;
16 }
17
18 public String getId() {
19 return this.id;
20 }
21
22 public void setName(String name) {
23 this.name = name;
24 }
25
26 public String getName() {
27 return this.name;
28 }
29
30 public void setPassword(String password) {
31 this.password = password;
32 }
33
34 public String getPassword() {
35 return this.password;
36 }
37
38 public void setAge(String age) {
39 this.age = age;
40 }
41
42 public String getAge() {
43 return this.age;
44 }
45
46 public void setCar(Car car) {
47 this.car = car;
48 }
49
50 public Car getCar() {
51 return this.car;
52 }
53
54 }
1 package per.swwfourteen.fourteen;
2
3 public class Car {
4 private String num;
5
6 public void setNum(String num) {
7 this.num = num;
8 }
9
10 public String getNum() {
11 return this.num;
12 }
13
14 }
1 package per.swwfourteen.fourteen;
2
3 import java.util.List;
4
5 public class Root_List {
6 private List<Student> student;
7
8 private List<Teacher> teacher;
9
10 public void setStudent(List<Student> student) {
11 this.student = student;
12 }
13
14 public List<Student> getStudent() {
15 return this.student;
16 }
17
18 public void setTeacher(List<Teacher> teacher) {
19 this.teacher = teacher;
20 }
21
22 public List<Teacher> getTeacher() {
23 return this.teacher;
24 }
25
26 }
1 package per.swwfourteen.fourteen;
2
3 import java.io.BufferedReader;
4 import java.io.File;
5 import java.io.FileReader;
6 import java.io.IOException;
7
8 import com.alibaba.fastjson.JSONObject;
9
10 public class JsonRoot {
11 public static void main(String[] args)throws Exception{
12 Root_List root=JSONObject.parseObject(ReadRoot("D:"+File.separator+"1.json"),Root_List.class);
13 for(Student s:root.getStudent()){
14 System.out.println(s.getId());
15 System.out.println(s.getName());
16 System.out.println(s.getAge());
17 System.out.println(s.getPassword());
18 }
19 for(Teacher t:root.getTeacher()){
20 System.out.println(t.getId());
21 System.out.println(t.getName());
22 System.out.println(t.getAge());
23 System.out.println(t.getPassword());
24 System.out.println(t.getCar());
25 }
26 }
27
28 public static String ReadRoot(String rer)throws IOException{
29 StringBuffer sb=new StringBuffer();
30 BufferedReader br=new BufferedReader(new FileReader(rer));
31 char[] it=new char[1024];
32 int sum=0;
33 while((sum=br.read(it))!=-1){
34 String s=String.valueOf(it, 0, sum);
35 sb.append(s);
36 }
37 br.close();
38 return sb.toString();
39 }
40 }
运行后提示13行报错,
Exception in thread "main" java.lang.NullPointerException at per.swwfourteen.fourteen.JsonRoot.main(JsonRoot.java:13)
1 {
2 "Root": {
3 "student": [
4 {
5 "id": "001",
6 "name": "student1",
7 "password": "123",
8 "age": "20"
9 },
10 {
11 "id": "002",
12 "name": "student2",
13 "password": "456",
14 "age": "21"
15 },
16 {
17 "id": "003",
18 "name": "student3",
19 "password": "123",
20 "age": "21"
21 }
22 ],
23 "teacher": [
24 {
25 "id": "001",
26 "name": "teacher1",
27 "password": "123",
28 "age": "20",
29 "car": { "num": "098" }
30 },
31 {
32 "id": "002",
33 "name": "teacher2",
34 "password": "123",
35 "age": "20",
36 "car": { "num": "098" }
37 }
38 ]
39 }
40 }
20 回答
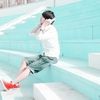
慕侠2389804
TA贡献1719条经验 获得超6个赞
@略知闻风雨: 无异常和无数据不冲突,语法没问题肯定无异常。。。。。。你把ReadRoot里面代码全删了也无异常。。。。,还是慢慢弄读json的代码
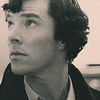
翻阅古今
TA贡献1780条经验 获得超5个赞
@博客园乄小光: 方的不行,这个是我自己练手的,不着急,我慢慢找抽空帮我感激不尽,读到Root_List root=JSONObject.parseObject(ReadRoot("D:"+File.separator+"1.json"),Root_List.class);
发现student和teacher获取到的都是null;然后for循环就不用想了,感觉应该是ReadRoot的问题,但是ReadRoot运行完无异常- -
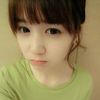
慕的地10843
TA贡献1785条经验 获得超8个赞
@略知闻风雨: 肯定啊,空对象,就是你的root中集合为空,取不到student对象,我是让你看第十二行
Root_List root=JSONObject.parseObject(ReadRoot("D:"+File.separator+"1.json"),Root_List.class);
这里面的root中有数据没,应该是没有,解析json获得数据为空
,你看下是不是
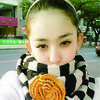
当年话下
TA贡献1890条经验 获得超9个赞
public List<Student> getStudent() { if(this.student==null)return new List<Student>(); return this.student; }
添加回答
举报
0/150
提交
取消