1 public class DoubleCacheQueue
2 {
3
4 public DoubleCacheQueue()
5 { }
6 public T Dequeue()
7 {
8 T t = default(T);
9
10 if (readQueue.Count > 0)
11 t= readQueue.Dequeue();
12 else
13 {
14 writeQueue = Interlocked.Exchange(ref readQueue, writeQueue);
15 }
16 return t;
17 }
18 public void Enqueue(T item)
19 {
20 writeQueue.Enqueue(item);
21 }
22
23 public int Count
24 {
25 get { return readQueue.Count; }
26 }
27 //读缓冲
28 private Queue readQueue = new Queue();
29 //写缓冲
30 private Queue writeQueue = new Queue();
31
32 }
补充下: 我应用的场景就是只有2个线程,我想实现一个lock free的队列 一个读线程不停的Dequeue 一个写线程不停的Enqueue 在只有2个线程一个单线程读和一个单线程写的情况下,能不能双线程安全?
5 回答
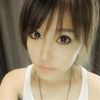
慕姐8265434
TA贡献1813条经验 获得超2个赞
MSDN说的很清楚了,Queue的Item如果是公共静态的就是线程安全的。否则自己保证。
Queue本身不保证枚举的线程安全。so 你这么做不是线程安全的。
可以考虑用ConcurrentQueue 类或者使用synchronized方法。
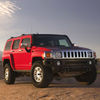
有只小跳蛙
TA贡献1824条经验 获得超8个赞
@garry:
public virtual object Dequeue()
{
if (this.Count == 0)
{
throw new InvalidOperationException(Environment.GetResourceString("InvalidOperation_EmptyQueue"));
}
object obj2 = this._array[this._head];
this._array[this._head] = null;
this._head = (this._head + 1) % this._array.Length;
this._size--;
this._version++;
return obj2;
}
public virtual void Enqueue(object obj)
{
if (this._size == this._array.Length)
{
int capacity = (int) ((this._array.Length * this._growFactor) / 100L);
if (capacity < (this._array.Length + 4))
{
capacity = this._array.Length + 4;
}
this.SetCapacity(capacity);
}
this._array[this._tail] = obj;
this._tail = (this._tail + 1) % this._array.Length;
this._size++;
this._version++;
}
帮你看了下内部代码,
1、他们使用了公用资源 this._head和this._tail 。
2、这两个方法没有临界区
3、这两个变量的计算没有使用原子操作
所以,多线程使用这两个方法会出现并发问题。
- 5 回答
- 0 关注
- 518 浏览
添加回答
举报
0/150
提交
取消