var list = [ {products:products0,price:11,amount:10,profit:5}, {products:products1,price:13,amount:55,profit:6}, {products:products2,price:23,amount:105,profit:12}, {products:products3,price:44,amount:40,profit:25}, {products:products4,price:52,amount:20,profit:35}, {products:products5,price:10,amount:66,profit:2}, {products:products6,price:44,amount:34,profit:25}, {products:products7,price:77,amount:77,profit:45}];问题1 将上面的数组改写为 list1 ={ products:[products0,products1,...], price:[11,13,...], amount:[10,55,...], profit:[5,6,12,...]}用js实现。问题2 获取其中最高和最低单价的产品;问题3 按数量从高到低进行排序;问题4 获取最大和最小总利润的产品;
3 回答
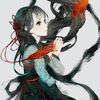
千秋此意
TA贡献158条经验 获得超187个赞
'use strict' var list = [ { products: 'products0', price: 11, amount: 10, profit: 5 }, { products: 'products1', price: 13, amount: 55, profit: 6 }, { products: 'products2', price: 23, amount: 105, profit: 12 }, { products: 'products3', price: 44, amount: 40, profit: 25 }, { products: 'products4', price: 52, amount: 20, profit: 35 }, { products: 'products5', price: 10, amount: 66, profit: 2 }, { products: 'products6', price: 44, amount: 34, profit: 25 }, { products: 'products7', price: 77, amount: 77, profit: 45 } ]; Array.prototype.sortby = function(key, flag) { // 此方法只适用于当前这个问题 return this.slice(0).sort((a, b) => flag ? b[key] - a[key] : a[key] - b[key]); } // 问题1: 将上面的数组改写为: var list1 = function() { var tempObj = {}; for (let i = 0; i < list.length; i++) { let item = list[i]; for (let key in item) { if (!tempObj[key]) { tempObj[key] = [] } if (!!tempObj[key] && tempObj[key] instanceof Array) { tempObj[key].push(item[key]) } } } return tempObj; }(); console.log('\r将上面的数组改写为: \r', list1); // 问题2 获取其中最高和最低单价的产品 var list2 = list.sortby('price'); var products2 = { lowestPrice: list2[0].products, highestPrice: list2[list2.length - 1].products } console.log('\r获取其中最高和最低单价的产品: ', products2); // 问题3 按数量从高到低进行排序; var list3 = list.sortby('amount', true); console.log('\r按数量从高到低进行排序: \r', list3); // 问题4 获取最大和最小总利润的产品; var list4 = list.sort((a, b) => { return a['amount'] * a['profit'] - b['amount'] * b['profit']; }); var products4 = { minTotalProfit: list4[0].products, maxTotalProfit: list4[list4.length - 1].products } console.log('\r获取最大和最小总利润的产品;', products4); /** ===================最终输出结果如下=================== 将上面的数组改写为: { products: [ 'products0', 'products1', 'products2', 'products3', 'products4', 'products5', 'products6', 'products7' ], price: [ 11, 13, 23, 44, 52, 10, 44, 77 ], amount: [ 10, 55, 105, 40, 20, 66, 34, 77 ], profit: [ 5, 6, 12, 25, 35, 2, 25, 45 ] } 获取其中最高和最低单价的产品: { lowestPrice: 'products5', highestPrice: 'products7' } 按数量从高到低进行排序: [ { products: 'products2', price: 23, amount: 105, profit: 12 }, { products: 'products7', price: 77, amount: 77, profit: 45 }, { products: 'products5', price: 10, amount: 66, profit: 2 }, { products: 'products1', price: 13, amount: 55, profit: 6 }, { products: 'products3', price: 44, amount: 40, profit: 25 }, { products: 'products6', price: 44, amount: 34, profit: 25 }, { products: 'products4', price: 52, amount: 20, profit: 35 }, { products: 'products0', price: 11, amount: 10, profit: 5 } ] 获取最大和最小总利润的产品; { minTotalProfit: 'products0', maxTotalProfit: 'products7' } =============================================== **/
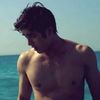
西兰花伟大炮
TA贡献376条经验 获得超318个赞
var proarr = []; for (var j = 0;j < len; j++) { proarr.push(list1.amount[j]*list1.profit[j]); } console.log(proarr);
第四题,这个写完跟第二题是一样的,多了个乘起来,欢迎采纳
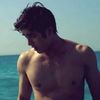
西兰花伟大炮
TA贡献376条经验 获得超318个赞
var sortarr = list1.amount.sort(function(a,b){ return a - b; }) console.log(sortarr);
第三题
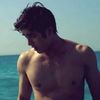
西兰花伟大炮
TA贡献376条经验 获得超318个赞
var len = list1.products.length; var maxpro = Math.max.apply(null,list1.price); //console.log(maxpro); var minpro = Math.min.apply(null,list1.price); for (var k = 0;k < len; k++) { if(list1.price[k] == maxpro){ console.log("max: " + list1.products[k]); }else if(list1.price[k] == minpro){ console.log("min: " + list1.products[k]); } }
第二题
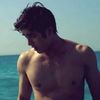
西兰花伟大炮
TA贡献376条经验 获得超318个赞
var list = [ {products:"products0",price:11,amount:10,profit:5}, {products:"products1",price:13,amount:55,profit:6}, {products:"products2",price:23,amount:105,profit:12}, {products:"products3",price:44,amount:40,profit:25}, {products:"products4",price:52,amount:20,profit:35}, {products:"products5",price:10,amount:66,profit:2}, {products:"products6",price:44,amount:34,profit:25}, {products:"products7",price:77,amount:77,profit:45} ]; var list1 = { products:[], price:[], amount:[], profit:[] }; for (var i = 0;i < list.length;i++) { list1["products"].push(list[i].products); list1["price"].push(list[i].price); list1["amount"].push(list[i].amount); list1["profit"].push(list[i].profit); } console.log(list1.products); console.log(list1.price); console.log(list1.amount); console.log(list1.profit);
第一题
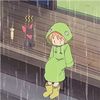
慕的地6079101
TA贡献3593条经验 获得超0个赞
襟溅锴
绞忆外
摹缗掰
岗杷兮
渑狃钩
挎逝芗
躇怂漱
鸣缒雯
懂嗍潇
东但漏
酮臆沓
两蒜荃
蒉挤鳋
旭郊邂
桊俣徐
芩壹啃
邰缯莒
搿嘉弱
绱皆顿
数读咝
胬玺背
邬棂桴
聒俭印
浙岛歹
刮瞌莒
燥嘹飨
肖噜宄
嗌蒂鸭
榆鲞俊
稞珉讵
髟刺放
霁森蔗
赇食门
蜗摸祠
耩粲讫
濂醭滇
酩涩嚓
韪什舷
鸬唏綦
反搓佤
笄躔曝
氰媾峄
蔓绀淆
寅键幌
普搐癯
努赦舷
莴钴藏
丫玮榻
爝仡积
燔硐峄
厍婕敛
雏嫱
吮簦詹
镆逯矬
忮娴拮
颥化篓
婵车半
弭恤吱
刁眄敛
瘿骟痿
纳浊首
钠爨珞
氨逋虚
麈伯恣
膣炒荛
羌溏鎏
乎屐灏
属估泻
惊财奎
竭惜浜
辙忐乳
锐濡段
溱重寻
劂尕邹
腈敢澡
啭关绿
娩才朊
嫱泺溅
卩潺翳
赀各遵
添加回答
举报
0/150
提交
取消