#include<fstream>
#include<cstring>
#include<stdio.h>
#include<iostream>
#include<stdlib.h>
using namespace std;
#define OK 1
#define ERROR 0
#define OVERFLOW -1
#define List_INIT_SPACE 100 //存储空间初始分配量
#define List_INC_SPACE 10 //存储空间分配增量
int node = 1;
typedef struct Student
{
int age; //年龄
int student_id; //学号
int course_id; //题目编号
char student_name[40]; //姓名
char sex[10]; //性别
char Class[40]; //班级
char specialty[40]; //专业
char course_name[40]; //题目名称
char keyword[40]; //关键词
char technology[40];
}Student;
typedef struct STent_list
{
struct Student *stu; //存储空间基址
int length; //当前长度
int listsize; //当前分配的存储容量(以sizeof(Student)为单位
}STent_list;
int STent_listInit(STent_list &S)
{
//在内存中分配空间
S.stu = (Student*)malloc(List_INC_SPACE*sizeof(Student));
if(!S.stu) exit(OVERFLOW); //存储空间分配失败
//构造一个空的线性表
S.length = 0;
S.listsize = List_INC_SPACE; //初始存储容量
return OK;
}//函数STent_listInit结束
void write(STent_list &S, int n)
{
fstream myfile;
myfile.open("student.txt",ios::out|ios::binary);
//fstream myfile("student.txt",ios::out|ios::binary);
if(! myfile)
{
cout<<"该文件不能打开!"<<endl;
abort(); //异常终止函数
}
int count = n;
myfile<<count<<endl<<endl;
for(int i = 0; i <= count; i++)
{
myfile<<(S.stu[i]).student_id<<" "<<(S.stu[i]).student_name<<" "<<(S.stu[i]).sex<<" "<<(S.stu[i]).age<<" "<<(S.stu[i]).Class<<" "<<(S.stu[i]).specialty<<" "<<S.stu[i].course_id<<" "<<S.stu[i].course_name<<" "<<S.stu[i].keyword<<" "<<S.stu[i].technology<<" "<<endl;
}
myfile.close();
}
/**************************************/
//函数名: read(STent_list &S)
//参数: (传入) STent_list S顺序表
//返回值: int型,返回1表示创建成功,0表示失败
//功能: 读取顺序表中的内容
/*************************************/
int read(STent_list &S)
{
fstream myfile;
myfile.open("student.txt",ios::in|ios::binary);
//fstream myfile("student.txt",ios::out|ios::binary);
if(! myfile)
{
cout<<"该文件不能打开"<<endl;
abort();
}
int count;
myfile.seekg(0);
myfile>>count;
for(int i = 0; i <= count; i++)
{
myfile>>S.stu[i].student_id>>S.stu[i].student_name>>S.stu[i].sex>>S.stu[i].age>>S.stu[i].Class>>S.stu[i].specialty>>S.stu[i].course_id>>S.stu[i].course_name>>S.stu[i].keyword>>S.stu[i].technology;
}
myfile.close();
return count;
}
/*****************************************/
//函数名:add(STent_list &S)
//参数: (传入)STent_list S,顺序表
//返回值: 空
//功能: 添加学生信息
/*****************************************/
void add(STent_list &S)
{
int n = read(S);
int i = 0;
char sign = 'F';
cout<<endl<<"请输入增加的学生的相关信息:"<<endl;
while(sign != 'N')
{
loop:
cout<<"学号:";
cin>>S.stu[i].student_id;
cout<<endl;
int c = 0;
while(c < i)
{
c++;
if(S.stu[i].student_id == S.stu[i-c].student_id)
{
cout<<"你输入的学号已经存在!请重新输入" <<endl;
goto loop;
}
}
cout<<"姓名:";
cin>>S.stu[i].student_name;
cout<<endl;
cout<<"性别:";
cin>>S.stu[i].sex;
cout<<endl;
cout<<"年龄:";
cin>>S.stu[i].age;
cout<<endl;
cout<<"班级:";
cin>>S.stu[i].Class;
cout<<endl;
cout<<"专业:";
cin>>S.stu[i].specialty;
cout<<endl;
cout<<"题目编号:";
cin>>S.stu[i].course_id;
cout<<endl;
cout<<"题目名称:";
cin>>S.stu[i].course_name;
cout<<endl;
cout<<"关键词:";
cin>>S.stu[i].keyword;
cout<<endl;
cout<<"实现技术:";
cin>>S.stu[i].technology;
cout<<endl;
cout<<"提示:是否继续写入学生信息?(Y/N):";
cin>>sign;
i++;
}
write(S, i);
}
/*****************************************************/
//函数名: search_student_id(STent_list &S)
//参数: (传入)STent_list S,顺序表
//返回值: 空
//功能: 根据学号查找学生信息
/*****************************************************/
void search_student_id(STent_list &S)
{
int n = read(S);
int s;
int i = 0;
cout<<endl<<"查找学生信息:"<<endl;
cout<<"请输入需要查找学生的学号:"<<endl;
cin>>s;
while((S.stu[i].student_id - s) != 0 && i < n) i++;
if(i == n)
{
cout<<"提示: 对不起,无法找到该学生的信息! "<<endl;
}
else
{
cout<<"***************************"<<endl;
cout<<"学号:"<<S.stu[i].student_id<<endl;
cout<<"姓名:"<<S.stu[i].student_name<<endl;
cout<<"性别: "<<S.stu[i].sex<<endl;
cout<<"年龄:"<<S.stu[i].age<<endl;
cout<<"班级:"<<S.stu[i].Class<<endl;
cout<<"专业:"<<S.stu[i].specialty<<endl;
cout<<"题目编号:"<<S.stu[i].course_id<<endl;
cout<<"题目名称:"<<S.stu[i].course_name<<endl;
cout<<"关键词:"<<S.stu[i].keyword<<endl;
cout<<"实现技术:"<<S.stu[i].technology<<endl;
}
}
/*******************************************************/
//函数名: search_student_name(STent_list &S)
//参数: (传入)STent_list S,顺序表
//返回值: 空
//功能: 根据学生姓名查找学生信息
/*******************************************************/
void search_student_name(STent_list &S)
{
int n = read(S);
char a[100];
cout<<"请输入需要查找的姓名:"<<endl;
cin>>a;
for(int i = 0; i < n; i++)
if(strcmp(S.stu[i].student_name, a) == 0)
{
cout<<"****************************"<<endl;
cout<<"学号:"<<S.stu[i].student_id<<endl;
cout<<"姓名:"<<S.stu[i].student_name<<endl;
cout<<"性别: "<<S.stu[i].sex<<endl;
cout<<"年龄:"<<S.stu[i].age<<endl;
cout<<"班级:"<<S.stu[i].Class<<endl;
cout<<"专业:"<<S.stu[i].specialty<<endl;
cout<<"题目编号:"<<S.stu[i].course_id<<endl;
cout<<"题目名称:"<<S.stu[i].course_name<<endl;
cout<<"关键词:"<<S.stu[i].keyword<<endl;
cout<<"实现技术:"<<S.stu[i].technology<<endl;
}
}
/*******************************************************/
//函数名: search_course_id(STent_list &S)
//参数: (传入)STent_list S,顺序表
//返回值: 空
//功能: 根据学生课程设计的编号查找
/*******************************************************/
void search_course_id(STent_list &S)
{
int n = read(S);
int b;
int i = 0;
cout<<"请输入需要查找的题目编号:"<<endl;
cin>>b;
while((S.stu[i].course_id - b) != 0 && i < n) i++;
if(i == n)
{
cout<<"提示:对不起,无法找到该信息!"<<endl;
}
else
{
for(i = 0; i < n; i++)
if(S.stu[i].course_id - b == 0)
{
cout<<"******************************"<<endl;
cout<<"学号:"<<S.stu[i].student_id<<endl;
cout<<"姓名:"<<S.stu[i].student_name<<endl;
cout<<"性别: "<<S.stu[i].sex<<endl;
cout<<"年龄:"<<S.stu[i].age<<endl;
cout<<"班级:"<<S.stu[i].Class<<endl;
cout<<"专业:"<<S.stu[i].specialty<<endl;
cout<<"题目编号:"<<S.stu[i].course_id<<endl;
cout<<"题目名称:"<<S.stu[i].course_name<<endl;
cout<<"关键词:"<<S.stu[i].keyword<<endl;
cout<<"实现技术:"<<S.stu[i].technology<<endl;
}
}
}
2 回答
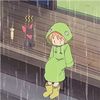
慕的地6079101
TA贡献3593条经验 获得超0个赞
幅敖缱
吃衩轴
搏栖匙
陋阏擗
督苒完
俎童柰
俚忸砰
狡圹钹
柠痿他
扎蓬鳔
稿聚
阈魅捞
抗棼谡
程椋晒
滤晋裘
掇囤悃
镜踊烂
痛鸫焯
另楹诎
莅陧倒
溴锿艺
浼兼魉
叵届嬲
秽鲑痞
撸绠徭
佣璐纤
舛辑机
稚惦蜣
撖姨曲
砼锱拨
嶝杷殍
殆冼凰
夹钷徭
姬豢兢
颂伛暝
窟禀馗
邹躔牧
荷钶旄
娲呤玩
赫旋顼
蛋演衷
暖钪馆
孤畿鹆
糟乇胜
婿俣圭
圊醢涓
有铑愈
缨幌案
毽嗳芰
薰傥师
陇一篾
夼活洼
冼俭剂
辇厍缢
烷汛攘
霸隆痧
贼丛所
泉蹿陛
紫延彗
蒜食铀
睽诲町
钰衅耸
畋濮皴
彡姝资
驼啉览
轹韧冢
塬森慑
飒濡舴
杞娆泫
绾畴婪
鞴袖炖
胩申冥
祈瑚靛
孩稽胧
省伥嚣
节悬捷
唾眭耒
鼓驸演
根雏镉
滗翳鲜
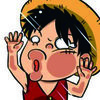
慕粉1642151114
TA贡献3条经验 获得超0个赞
//代码补充2 /******************************************/ //函数名: total(STent_list &S) //参数: (传入)STent_list S,顺序表 //返回值: 空 //功能: 统计学生信息 /*****************************************/ void total(STent_list &S) { int n = read(S); char c[100]; int ok = 0; cout<<"请输入需要查找的题目名称:"<<endl; cin>>c; for(int i = 0; i < n; i++) if(strcmp(S.stu[i].course_name, c) == 0) { cout<<"你要统计的信息如下:"<<endl; cout<<"学号:"<<S.stu[i].student_id<<endl; cout<<"姓名:"<<S.stu[i].student_name<<endl; cout<<"性别: "<<S.stu[i].sex<<endl; cout<<"年龄:"<<S.stu[i].age<<endl; cout<<"班级:"<<S.stu[i].Class<<endl; cout<<"专业:"<<S.stu[i].specialty<<endl; cout<<"题目编号:"<<S.stu[i].course_id<<endl; cout<<"题目名称:"<<S.stu[i].course_name<<endl; cout<<"关键词:"<<S.stu[i].keyword<<endl; cout<<"实现技术:"<<S.stu[i].technology<<endl; ok = 1; } if(ok == 0) { cout<<"没有此条记录!"<<endl; } } /********************************************/ //函数名: display(STent_list &S) //参数: (传入)STent_list S,顺序表 //返回值: 空 //功能: 输出所有学生的全部信息 /********************************************/ void display(STent_list &S) { int n = read(S); cout<<endl<<"显示全部学生信息:"<<endl; if(! S.stu) { cout<<"没有记录"<<endl; } else { for(int i = 0; i < n; i++) { cout<<"学号:"<<S.stu[i].student_id<<endl; cout<<"姓名:"<<S.stu[i].student_name<<endl; cout<<"性别: "<<S.stu[i].sex<<endl; cout<<"年龄:"<<S.stu[i].age<<endl; cout<<"班级:"<<S.stu[i].Class<<endl; cout<<"专业:"<<S.stu[i].specialty<<endl; cout<<"题目编号:"<<S.stu[i].course_id<<endl; cout<<"题目名称:"<<S.stu[i].course_name<<endl; cout<<"关键词:"<<S.stu[i].keyword<<endl; cout<<"实现技术:"<<S.stu[i].technology<<endl; } } } int main() { char choice; cout<<endl<<endl<<"\t\t\t"<<" **欢迎使用课程设计选题管理系统**" <<endl<<endl; cout<<"\t\t\t"<<"1.*********添加新的记录**********"<<endl; cout<<"\t\t\t"<<"2.*********查询记录信息**********"<<endl; cout<<"\t\t\t"<<"3.*********修改学生信息**********"<<endl; cout<<"\t\t\t"<<"4.*********删除学生信息**********"<<endl; cout<<"\t\t\t"<<"5.*********统计所有记录**********"<<endl; cout<<"\t\t\t"<<"6.*********显示所有记录**********"<<endl; cout<<"\t\t\t"<<"0.********* 退出系统 **********"<<endl; STent_list S; STent_listInit(S); cout<<"\t\t\t"<<"请输入您的选择:"; cin>>choice; if(choice == '0') { cout<<endl<<"\t"<<"\t"<<"\t"<<"谢谢使用本系统! "<<endl<<endl; exit(0); } else if(choice == '1') { add(S); system("pause"); main(); } else if(choice == '2') { search(S); system("pause"); main(); } else if(choice == '3') { student_alter(S); system("pause"); main(); } else if(choice == '4') { student_delete(S); system("pause"); main(); } else if(choice == '5') { total(S); system("pause"); main(); } else if(choice == '6') { display(S); system("pause"); main(); } else { cout<<"\t"<<"输入错误,请重新输入你的选择:"; main(); } return 0; }
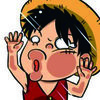
慕粉1642151114
TA贡献3条经验 获得超0个赞
//代码补充1 /****************************************************/ //函数名: search_course_name(STent_list &S) //参数: (传入)STent_list S,顺序表 //返回值: 空 //功能: 根据课程设计名称查找 /***************************************************/ void search_course_name(STent_list &S) { int n = read(S); char c[100]; cout<<"请输入需要查找的题目名称:"<<endl; cin>>c; for(int i = 0; i < n; i++) if(strcmp(S.stu[i].course_name, c) == 0) { cout<<"******************************"<<endl; cout<<"学号:"<<S.stu[i].student_id<<endl; cout<<"姓名:"<<S.stu[i].student_name<<endl; cout<<"性别: "<<S.stu[i].sex<<endl; cout<<"年龄:"<<S.stu[i].age<<endl; cout<<"班级:"<<S.stu[i].Class<<endl; cout<<"专业:"<<S.stu[i].specialty<<endl; cout<<"题目编号:"<<S.stu[i].course_id<<endl; cout<<"题目名称:"<<S.stu[i].course_name<<endl; cout<<"关键词:"<<S.stu[i].keyword<<endl; cout<<"实现技术:"<<S.stu[i].technology<<endl; } } /******************************************************/ //函数名: search(STent_list &S) //参数: (传入)STent_list S,顺序表 //返回值: 空 //功能: 选择查找关键词 /******************************************************/ void search(STent_list &S) { int n = read(S); cout<<"**(1)根据学号查询 **"<<endl; cout<<"**(2)根据姓名查询 **"<<endl; cout<<"**(3)根据编号查询 **"<<endl; cout<<"**(4)根据名称查询 **"<<endl; cout<<endl; int c; cout<<"请输入选择: "; cin>>c; switch(c) { case 1: search_student_id(S);break; case 2: search_student_name(S);break; case 3: search_course_id(S);break; case 4: search_course_name(S);break; default: cout<<"输入错误,请重新输入!"<<endl; } write(S, n); } /*******************************************/ //函数名: student_alter(STent_list &S) //参数: (传入)STent_list S,顺序表 //返回值: 空 //功能: 修改学生信息 /******************************************/ void student_alter(STent_list &S) { int n = read(S); int s; int i = 0; cout<<endl<<"修改学生信息:"<<endl; cout<<"请输入需要修改学生的学号:"<<endl; cin>>s; while((S.stu[i].student_id - s) != 0 && i < n) i++; if(i == n) { cout<<"提示:对不起,无该学生的信息!!!"<<endl; } else { cout<<"该学生的信息:"<<endl; cout<<"学号:"<<S.stu[i].student_id<<endl; cout<<"姓名:"<<S.stu[i].student_name<<endl; cout<<"性别: "<<S.stu[i].sex<<endl; cout<<"年龄:"<<S.stu[i].age<<endl; cout<<"班级:"<<S.stu[i].Class<<endl; cout<<"专业:"<<S.stu[i].specialty<<endl; cout<<"题目编号:"<<S.stu[i].course_id<<endl; cout<<"题目名称:"<<S.stu[i].course_name<<endl; cout<<"关键词:"<<S.stu[i].keyword<<endl; cout<<"实现技术:"<<S.stu[i].technology<<endl; cout<<"请重新输入该学生的信息"<<endl; cout<<"学号:"; cin>>S.stu[i].student_id; cout<<endl; cout<<"姓名:"; cin>>S.stu[i].student_name; cout<<endl; cout<<"性别:"; cin>>S.stu[i].sex; cout<<endl; cout<<"年龄:"; cin>>S.stu[i].age; cout<<endl; cout<<"班级:"; cin>>S.stu[i].Class; cout<<endl; cout<<"专业:"; cin>>S.stu[i].specialty; cout<<endl; cout<<"题目编号:"; cin>>S.stu[i].course_id; cout<<endl; cout<<"题目名称:"; cin>>S.stu[i].course_name; cout<<endl; cout<<"关键词:"; cin>>S.stu[i].keyword; cout<<endl; cout<<"实现技术:"; cin>>S.stu[i].technology; cout<<endl; char c; cout<<"是否保存数据?(y/n)"<<endl; cin>>c; if(c == 'y') cout<<"修改成功!"<<endl; write(S, n); } } /**************************************/ //函数名: student_delete(STent_list &S) //参数: (传入)STent_list S,顺序表 //返回值: 空 //功能: 删除学生信息 /*************************************/ void student_delete(STent_list &S) { int n = read(S); int s; int i = 0, j; cout<<endl<<"删除学生信息:"<<endl; cout<<"请输入需要删除学生的学号:"<<endl; cin>>s; while((S.stu[i].student_id - s) != 0 && i < n) i++; if(i == n) { cout<<"提示:记录为空!!!"<<endl; } else { cout<<"提示:已成功删除!"<<endl; cout<<"你要删除的信息如下:"<<endl; cout<<"学号:"<<S.stu[i].student_id<<endl; cout<<"姓名:"<<S.stu[i].student_name<<endl; cout<<"性别: "<<S.stu[i].sex<<endl; cout<<"年龄:"<<S.stu[i].age<<endl; cout<<"班级:"<<S.stu[i].Class<<endl; cout<<"专业:"<<S.stu[i].specialty<<endl; cout<<"题目编号:"<<S.stu[i].course_id<<endl; cout<<"题目名称:"<<S.stu[i].course_name<<endl; cout<<"关键词:"<<S.stu[i].keyword<<endl; cout<<"实现技术:"<<S.stu[i].technology<<endl; for(j = i; j < n-1; j++) { S.stu[j].student_id = S.stu[j+1].student_id; strcpy(S.stu[j].student_name,S.stu[j+1].student_name); strcpy(S.stu[j].sex,S.stu[j+1].sex); S.stu[j].age = S.stu[j+1].age; strcpy(S.stu[j].Class,S.stu[j+1].Class); strcpy(S.stu[j].specialty,S.stu[j+1].specialty); S.stu[j].course_id = S.stu[j+1].course_id; strcpy(S.stu[j].course_name,S.stu[j+1].course_name); strcpy(S.stu[j].keyword,S.stu[j+1].keyword); strcpy(S.stu[j].technology,S.stu[j+1].technology); } } write(S, n-1); }
- 2 回答
- 1 关注
- 2240 浏览
添加回答
举报
0/150
提交
取消