写一个Java应用程序,使用RandomAccessFile流统计Hello.txt中的单词,要求如下:
(1)计算全文中共出现了多少个单词(重复的单词只计算一次);
(2)统计出有多少个单词只出现了一次;
(3)统计并显示出每个单词出现的频率,并将这些单词按出现频率高低顺序显示在一个TextArea中
import java.io.*;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import javax.swing.JFrame;
import javax.swing.JTextArea;
public class T4 {
@SuppressWarnings("unchecked")
public static void main(String[] args) throws IOException {
String fileName = "OPEN FILE.txt";
RandomAccessFile file = new RandomAccessFile(new File(fileName), "r");
String content = null;
Map<String, Integer> map = new HashMap<String, Integer>();
while((content = file.readLine()) != null){
String[] ary = content.replaceAll("'|\\?|,|\\.|", "").split("\\s+");
for(String str : ary){
if(map.containsKey(str.trim())){
map.put(str, new Integer(map.get(str).intValue() + 1));
}else{
map.put(str.trim(), new Integer(1));
}
}
} //这里是在存储键值对吗?
List list = new ArrayList();
for (Iterator iter = map.entrySet().iterator(); iter.hasNext();) {
Map.Entry<String, Integer> element = (Map.Entry<String, Integer>) iter.next();
list.add(new WordFreq(element.getKey(), element.getValue()));
} //这里是在遍历哈希列表对吗?
Collections.sort(list, new Comparator<WordFreq>(){
public int compare(WordFreq o1, WordFreq o2) {
return o1.getCount() < o2.getCount()? 1: -1;
}
}); //collections.sort这段代码是什么意思呢?
int uniqueCount = 0;
final StringBuilder sb = new StringBuilder();
final String NEW_LINE = "\r\n";
for(Object obj: list){
WordFreq item = (WordFreq) obj;
uniqueCount += (item.getCount() == 1? 1: 0);
sb.append(item.toString());
sb.append(NEW_LINE);
} //上面这段代码是在做什么呢?
JFrame f = new JFrame();
JTextArea result = new JTextArea();
result.append("Total " + list.size() + " words found in " + fileName);
result.append(NEW_LINE);
result.append("Total " + uniqueCount + " words appear only once");
result.append(NEW_LINE);
result.append(NEW_LINE);
result.append(sb.toString());
f.add(result);
f.setVisible(true);
f.pack();
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
class WordFreq{
private String word;
private int count;
public WordFreq(String word, int count){
this.word = word;
this.count = count;
}
public int getCount() {
return count;
}
public String toString(){
return word + "\t" + count;
}
} //定义的这个类的作用是什么呢?
1 回答
已采纳
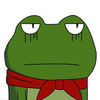
sntatas
TA贡献53条经验 获得超11个赞
这里是在存储键值对吗? 对的
这里是在遍历哈希列表对吗? 对的
collections.sort这段代码是什么意思?
这里是对list中的元素进行排序,根据集合中的元素的count实例变量的值的进行排序从大到小排序,这是一种策略模式
上面这段代码在做什么?
获取list中所有元素的字符串形式到sb对象中
定义这个类是做什么用的?
集合中的对象就是这个类
看样子你对Java集合没有搞清楚,建议你去学学Java集合,还有字符串对象String、StringBuilder、StringBuffer区别
添加回答
举报
0/150
提交
取消