class BankAccount:
def __init__(self, acct_number, acct_name):
self.acct_number = acct_number
self.acct_name = acct_name
self.balance = 0.0
def displayBalance(self):
print "The account balance is:", self.balance
def deposit(self, amount):
self.balance = self.balance + amount
print "You deposited", amount
print "The new balance is:", self.balance
def withdraw(self, amount):
if self.balance >= amount:
self.balance = self.balance - amount
print "You withdrew", amount
print "The new balance is:", self.balance
else:
print "You tried to withdraw", amount
print "The account balance is:", self.balance
print "Withdrawal denied. Not enough funds."
class InterestAccount(BankAccount):
def addInterest(self, rate):
interest = self.balance * rate
print "adding interest to the account,", rate * 100,"percent"
self.deposit (interest)
myAccount = BankAccount(234, "Warren Sande")
print "Account name:",myAccount.acct_name
print "Account number:", myAccount.acct_number
myAccount.displayBalance()
myAccount.deposit(34.52)
myAccount.addInterest(0.11)运行时提示:Traceback (most recent call last): File "D:\Workspaces\MyEclipse Professional 2014\NO1\helloworld\__init__.py", line 46, in <module> myAccount.addInterest(0.11)AttributeError: BankAccount instance has no attribute 'addInterest'不知为何出错,请指教。
3 回答
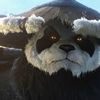
小猫过河
TA贡献26条经验 获得超15个赞
myAccount下没有'addInterest'属性,报错也是这个意思,你漏写了一句。
class BankAccount: def __init__(self, acct_number, acct_name): self.acct_number = acct_number self.acct_name = acct_name self.balance = 0.0 def displayBalance(self): print "The account balance is:", self.balance def deposit(self, amount): self.balance = self.balance + amount print "You deposited", amount print "The new balance is:", self.balance def withdraw(self, amount): if self.balance >= amount: self.balance = self.balance - amount print "You withdrew", amount print "The new balance is:", self.balance else: print "You tried to withdraw", amount print "The account balance is:", self.balance print "Withdrawal denied. Not enough funds." class InterestAccount(BankAccount): def addInterest(self, rate): interest = self.balance * rate print "adding interest to the account,", rate * 100,"percent" self.deposit (interest) myAccount = InterestAccount(234, "Warren Sande") #看这里 # myAccount = BankAccount(234, "Warren Sande") print "Account name:",myAccount.acct_name print "Account number:", myAccount.acct_number myAccount.displayBalance() myAccount.deposit(34.52) myAccount.addInterest(0.11)
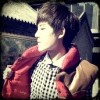
有心寻性
TA贡献5条经验 获得超0个赞
InterestAccount继承BankAccount,也就是InterestAccount有BankAccount的所有方法和属性,反过来BankAccount是没有InterestAccount的属性和方法的,你声明的是BankAccount,所以没有addInterest这个方法了.你要声明InterestAccount才行
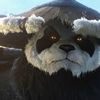
小猫过河
TA贡献26条经验 获得超15个赞
AttributeError: BankAccount instance has no attribute 'addInterest'
说没有这个法属性,看看有没有'addInterest',从你的代码里我没找到,可能是你漏写了。
添加回答
举报
0/150
提交
取消