package com.cards;
import java.util.ArrayList;
import java.util.List;
public class Card implements Comparable<Card> {
private String num;
private String type;
private String[] types = { "黑桃", "红桃", "梅花", "方片" };
private String[] cha = { "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K", "A" };
public String getNum() {
return num;
}
public String getType() {
return type;
}
public List<String> changStringToList(String[] Array) {
List<String> str = new ArrayList<String>();
for (int i = 0; i < Array.length; i++) {
str.add(Array[i]);
}
return str;
}
public Card(String type, String num) {
this.type = type;
this.num = num;
}
public void printCard() {
System.out.print(this.type + this.num);
}
@Override
public int compareTo(Card card) {
// TODO Auto-generated method stub
if (!(this.num.equals(card.num))) {
Integer i = this.changStringToList(cha).indexOf(this.num);
Integer j = this.changStringToList(cha).indexOf(card.num);
return i.compareTo(j);
} else {
Integer i = this.changStringToList(types).indexOf(this.type);
Integer j = this.changStringToList(types).indexOf(card.type);
return i.compareTo(j);
}
}
}
package com.cards;
import java.util.ArrayList;
import java.util.List;
public class Customer {
public int ID;
public String name;
public List<Card> cards = new ArrayList<Card>();
// public Cards card1;
// public Cards card2;
public Customer(int ID, String name) {
this.ID = ID;
this.name = name;
}
public void resetCards(){
cards = new ArrayList<Card>();
}
public void setCard(Card cards) {
this.cards.add(cards);
}
}
package com.cards;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Scanner;
public class PlayGames {
List<Card> cards = new ArrayList<Card>();
List<Customer> customers = new ArrayList<Customer>();
public void initiateCards() {
String[] types = { "黑桃", "红桃", "梅花", "方片" };
String[] cha = { "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K", "A" };
System.out.println("-------------创建扑克牌--------------");
for (int i = 0; i < types.length; i++) {
for (int j = 0; j < cha.length; j++) {
cards.add(new Card(types[i], cha[j]));
}
}
System.out.println("-------------扑克牌创建成功!--------------");
}
public void shuffleCards() {
Collections.shuffle(cards);
System.out.println("-------------洗牌结束!--------------");
}
public void createCards() {
initiateCards();
for (Card card : cards) {
System.out.print(card.getType() + card.getNum() + ",");
}
System.out.println();
System.out.println("总共有" + cards.size() + "张牌。");
shuffleCards();
}
public void createCustomer() {
Scanner scanner;
for (int i = 1; i <= 2; i++) {
System.out.println("请输入第" + i + "位玩家的ID和姓名:");
System.out.println("输入ID");
int id;
while (true) {
try {
scanner = new Scanner(System.in);
id = scanner.nextInt();
break;
} catch (Exception e) {
System.out.println("请输入整数类型的ID!");
continue;
}
}
System.out.println("输入姓名");
String name = scanner.next();
customers.add(new Customer(id, name));
System.out.println("--------欢迎玩家:" + name + "------------");
}
}
public void sendCards() {
System.out.println("-------------开始发牌-----------");
int sentNum = 0;
// for (int cirecle = 0; cirecle < cards.size()/customers.size();
// cirecle++) {
for (int cirecle = 0; cirecle < 4 / customers.size(); cirecle++) {
for (Customer customer : customers) {
System.out.println("玩家 " + customer.name + " 拿牌");
customer.setCard(cards.get(sentNum));
sentNum++;
}
}
System.out.println("----------发牌结束------------");
}
public void startGames() {
List<Card> cardsMax = new ArrayList<Card>();
System.out.println("----------开始游戏------------");
// 取得当前玩家手上最大的牌
for (Customer customer : customers) {
List<Card> cardMaxOfCustomer = new ArrayList<Card>();
cardMaxOfCustomer = customer.cards;
Collections.sort(cardMaxOfCustomer);
Collections.reverse(cardMaxOfCustomer);
cardsMax.add(customer.cards.get(0));
System.out.print("玩家: " + customer.name + " 最大的手牌为:");
customer.cards.get(0).printCard();
System.out.println();
}
// 取得最大的牌
Collections.sort(cardsMax);
Collections.reverse(cardsMax);
for (Customer customer : customers) {
if (customer.cards.contains(cardsMax.get(0))) {
System.out.println("-----------玩家: " + customer.name + " 获胜 !---------");
break;
}
}
// 打印玩家手上各自的牌
System.out.println("玩家各自的手牌为:");
for (Customer customer : customers) {
System.out.print(customer.name + ":");
for (Card card : customer.cards) {
card.printCard();
System.out.print(" ");
}
System.out.println("");
}
}
public static void main(String[] args) {
PlayGames c = new PlayGames();
c.createCards();
c.createCustomer();
c.sendCards();
c.startGames();
}
}
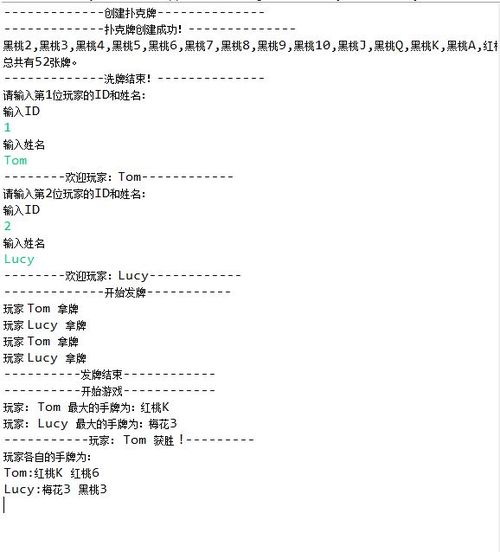