// Car.java
public class Car {
public String name;
public int rent_money;
public int capacity_person;
public int capacity_goods;
public Car(String name, int rent_money, int capacity_person, int capacity_goods) {
this.name = name;
this.rent_money = rent_money;
this.capacity_person = capacity_person;
this.capacity_goods = capacity_goods;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getRent_money() {
return rent_money;
}
public void setRent_money(int rent_money) {
this.rent_money = rent_money;
}
public int getCapacity_person() {
return capacity_person;
}
public void setCapacity_person(int capacity_person) {
this.capacity_person = capacity_person;
}
public int getCapacity_goods() {
return capacity_goods;
}
public void setCapacity_goods(int capacity_goods) {
this.capacity_goods = capacity_goods;
}
}
// SmallCar.java
public class SmallCar extends Car {
public SmallCar(String name, int rent_money, int capacity_person, int capacity_goods) {
super(name, rent_money, capacity_person, capacity_goods);
}
}
// Bus.java
public class Bus extends Car {
public Bus(String name, int rent_money, int capacity_person, int capacity_goods) {
super(name, rent_money, capacity_person, capacity_goods);
}
}
// Pick.java
public class Pick extends Car {
public Pick(String name, int rent_money, int capacity_person, int capacity_goods) {
super(name, rent_money, capacity_person, capacity_goods);
}
}
// Truck.java
public class Truck extends Car {
public Truck(String name, int rent_money, int capacity_person, int capacity_goods) {
super(name, rent_money, capacity_person, capacity_goods);
}
}
// Test.java
import java.util.Scanner;
public class Test {
public static void main(String[] args) {
System.out.println("欢迎使用哒哒租车系统:");
System.out.println("您是否需要租车:1是 0否");
int is_rent = 0; // 是否租车
is_rent = new Scanner(System.in).nextInt();
if (is_rent == 1) {
System.out.println("您可租车的类型及价目表:");
Car cars[] = {
new SmallCar("奥迪A4", 500, 4, 0),
new SmallCar("马自达6", 400, 4, 0),
new Pick("皮卡雪6", 450, 4, 2),
new Bus("金龙", 800, 20, 0),
new Truck("松花江", 400, 0, 4),
new Truck("依维柯", 1000, 0, 20),
};
System.out.println("序号 汽车名称 租金 容量");
for (int i = 0; i < cars.length; i++) {
System.out.println(
i + 1 + " " + cars[i].getName() + " " + cars[i].getRent_money() + "元/天" + " 载人:" + cars[i].getCapacity_person()
+ "人 载货:" + cars[i].getCapacity_goods() + "吨"
);
}
System.out.println("请输入您要租赁汽车的数量");
int rent_count = 0; // 租车数量
rent_count = new Scanner(System.in).nextInt();
Car rent_cars[] = new Car[rent_count];
for (int i = 0; i < rent_count; i++) {
System.out.println("请输入第" + (i + 1) + "辆租赁的汽车序号");
int number = 0; // 租车序号
number = new Scanner(System.in).nextInt();
rent_cars[i] = cars[number - 1];
}
int rent_days = 0; // 租车天数
System.out.println("请输入您要租赁的天数");
rent_days = new Scanner(System.in).nextInt();
int person_count = 0; // 总载人数
int goods_count = 0; // 总载货数
int rent_money_a_day = 0; // 租所选择的所有车每天的租金
System.out.println("您的账单:");
System.out.println("*****可载人的车有:");
for (int i = 0; i < rent_cars.length; i++) {
if (!(rent_cars[i] instanceof Truck)) {
System.out.print(rent_cars[i].getName() + " ");
}
person_count += rent_cars[i].getCapacity_person();
goods_count += rent_cars[i].getCapacity_goods();
rent_money_a_day += rent_cars[i].getRent_money();
}
System.out.println("共载人:" + person_count + "人");
System.out.println("*****可载货的车有:");
for (int i = 0; i < rent_cars.length; i++) {
if ((rent_cars[i] instanceof Truck) || (rent_cars[i] instanceof Pick)) {
System.out.print(rent_cars[i].getName() + " ");
}
}
System.out.println("共载货:" + goods_count + "吨货");
System.out.println("*****租车总价格:" + rent_money_a_day * rent_days + "元");
} else {
System.out.println("退出哒哒租车系统");
}
System.exit(0);
}
}
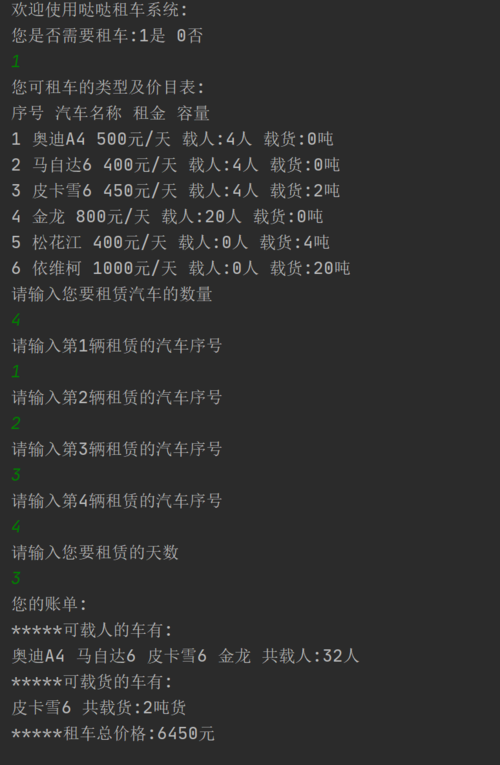