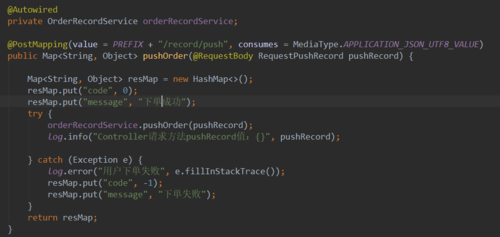
@Override
@Path("push")
@POST
@Consumes(value = MediaType.APPLICATION_JSON)
@Produces(value = MediaType.APPLICATION_JSON)
public BaseResponse pushOrder(PushOrderDto dto) {
if (dto.getItemId() == null || dto.getItemId() <= 0 || Strings.isNullOrEmpty(dto.getCustomerName())
|| dto.getTotal() == null) {
return new BaseResponse(StatusCode.InvalidParams);
}
log.info("请求过来的参数:{} ", dto);
BaseResponse response = new BaseResponse(StatusCode.Success);
try {
//TODO:实际的业务逻辑
//TODO:校验商品信息是否存在
ItemInfo info = itemInfoMapper.selectByPrimaryKey(dto.getItemId());
if (info == null) {
return new BaseResponse(StatusCode.NotAllowParams);
}
//TODO:库存服务-校验....
//TODO:客户中心服务-校验....
//TODO:订单服务-下单
OrderRecord entity = new OrderRecord();
BeanUtils.copyProperties(dto, entity);
entity.setOrderTime(new Date());
orderRecordMapper.insertSelective(entity);
response.setData(entity.getId());
} catch (Exception e) {
e.printStackTrace();
response = new BaseResponse(StatusCode.Fail.getCode(), e.getMessage());
}
return response;
}
package com.yy.dubbo.two.server.service;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.yy.dubbo.two.server.request.RequestPushRecord;
import okhttp3.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.io.Serializable;
@Service
public class OrderRecordService implements Serializable{
private static final Logger log = LoggerFactory.getLogger(OrderRecordService.class);
@Autowired
private ObjectMapper objectMapper;
private OkHttpClient okHttpClient = new OkHttpClient();
private static final String URL = "http://192.168.110.1:9013/v1/record/push";
public void pushOrder(RequestPushRecord pushRecord) throws Exception {
try {
// 构建request builder
Request.Builder builder = new Request.Builder().url(URL).header("Content-Type","application/json");
// 构造请求体
RequestBody requestBody = RequestBody.create(MediaType.parse("application/json"),
objectMapper.writeValueAsString(pushRecord));
// 构造请求
Request request = builder.post(requestBody).build();
// 发起请求
Response response = okHttpClient.newCall(request).execute();
log.info(response.body().toString());
} catch (Exception e) {
throw e;
}
}
}