package com.imooc.dao;
import com.imooc.domain.Student;
import com.imooc.util.JDBCUtil;
import java.io.IOException;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
/**
* StudentDao访问接口实现类
*/
public class StudentDaoImpl implements StudentDao{
@Override
public List<Student> query() {
List<Student> students = new ArrayList<Student>();
Connection connection = null;
PreparedStatement preparedStatement = null;
ResultSet resultSet = null;
String sql = "select id , name , age from student";
try {
connection = JDBCUtil.getConnection();
preparedStatement = connection.prepareStatement(sql);
resultSet = preparedStatement.executeQuery();
//迭代resultSet
while (resultSet.next()){
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
int age = resultSet.getInt("age");
Student student = new Student();
student.setId(id);
student.setAge(age);
student.setName(name);
students.add(student);
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally {
JDBCUtil.release(resultSet,preparedStatement,connection);
}
return null;
}
}
package com.imooc.dao;
import com.imooc.domain.Student;
import org.junit.Test;
import java.util.List;
public class StudentDaoImplTest {
@Test
public void testQuery(){
StudentDao studentDao = new StudentDaoImpl();
List<Student> students = studentDao.query();
for(Student student : students){
System.out.println("id:"+student.getId()
+",name:"+student.getName()
+",age:"+student.getAge());
}
}
}
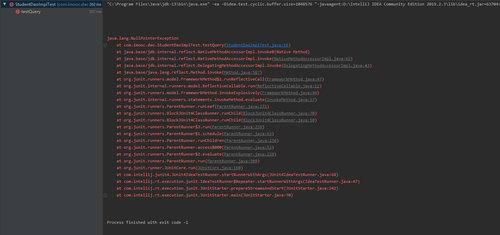