<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
html, body, div, table, tr, th, td, input {
margin: 0;
padding: 0;
}
a {
text-decoration: none;
color: #ff7474;
font-size: 14px;
display: block;
width: 60px;
margin: 0 auto;
}
button {
width: 160px;
line-height: 40px;
text-align: center;
margin: 0 auto;
display: block;
background-color: #2196F3;
color: #fff;
border: none;
border-radius: 3px;
outline: none;
}
table {
width: 600px;
margin: 50px auto;
table-layout: fixed;
border-collapse: collapse;
text-align: center;
line-height: 40px;
border: 1px solid #e2e2e2;
}
tr:nth-child(2n+1) {
background-color: #f7f7f7;
}
table tr:first-child{
background-color: #f7f7f7!important;
}
table .active{
background-color: #e0f1ff;
}
th, td {
border: none;
}
th {
color: #333;
font-size: 14px;
}
td {
color: #666;
font-size: 14px;
}
</style>
</head>
<body>
<table border="1" width="50%" id="table">
<tr>
<th>学号</th>
<th>姓名</th>
<th>操作</th>
</tr>
<tr>
<td>xh001</td>
<td>王小明</td>
<td class="del"><a href="javascript:;" onclick="del(this)">删除</a></td> <!--在删除按钮上添加点击事件 -->
</tr>
<tr>
<td>xh002</td>
<td>刘小芳</td>
<td class="del"><a href="javascript:;" onclick="del(this)">删除</a></td> <!--在删除按钮上添加点击事件 -->
</tr>
<tr>
<td>xh003</td>
<td>张三</td>
<td class="del"><a href="javascript:;" onclick="del(this)">删除</a></td> <!--在删除按钮上添加点击事件 -->
</tr>
<tr>
<td>xh004</td>
<td>李四</td>
<td class="del"><a href="javascript:;" onclick="del(this)">删除</a></td> <!--在删除按钮上添加点击事件 -->
</tr>
<tr>
<td>xh005</td>
<td>王五</td>
<td class="del"><a href="javascript:;" onclick="del(this)">删除</a></td> <!--在删除按钮上添加点击事件 -->
</tr>
<tr>
<td>xh006</td>
<td>赵柳</td>
<td class="del"><a href="javascript:;" onclick="del(this)">删除</a></td> <!--在删除按钮上添加点击事件 -->
</tr>
</table>
<button type="button" id="btn" onclick="add()">添加一行</button><!--在添加按钮上添加点击事件-->
</body>
<script>
let table = document.querySelector('#table');
function add() {
let node = document.createElement('tr');
table.childNodes[1].appendChild(node);
let num= parseInt( Math.random() * 10 );
let txt = getTxt(num);
node.innerHTML = '<td>xh00'+num+'</td><td>江'+ txt +'</td><td class="del"><a href="javascript:;" onclick="del(this)">删除</a></td>';
mouse()
}
function getTxt(num) {
let txt = '';
switch (num) {
case 0 :
txt = '灵';
break;
case 1 :
txt = '梦';
break;
case 2 :
txt = '叶';
break;
case 3 :
txt = '月';
break;
case 4 :
txt = '晚';
break;
case 5 :
txt = '晴';
break;
case 6 :
txt = '年';
break;
case 7 :
txt = '吟';
break;
case 8 :
txt = '言';
break;
case 9 :
txt = '烟';
break;
}
return txt
}
function del(that) {
table.childNodes[1].removeChild(that.parentNode.parentNode)
}
function mouse() {
let tr = table.querySelectorAll('tr');
for(let i=0;i<tr.length;i++){
tr[i].onmouseover = function () {
this.classList.add('active');
};
tr[i].onmouseout = function () {
this.classList.remove('active');
}
}
}
mouse()
</script>
</html>
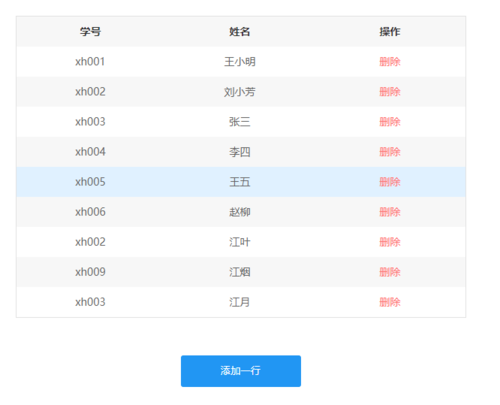