package pojo;
public class Book {
int id;//图书编号
String name;//图书名字
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Book(){
}
public Book(int id,String name){
this.id=id;
this.name=name;
}
public void show(){
System.out.println("书本编号为:"+id+"书本名称为:"+name);
}
}
package pojo;
//输入命令错误异常
public class InputErrorException extends Exception {
public InputErrorException(){
}
public InputErrorException(String message){
super(message);
}
}
package pojo;
//图书不存在异常
public class NotFindThisBookException extends Exception {
public NotFindThisBookException(){}//无参构造函数
public NotFindThisBookException(String message){
super(message);
}
}
package pojo;
import java.util.InputMismatchException;
import java.util.Scanner;
//显示与流程控制类
public class BookLent {
int num=0;
int num1=0;
int count=0;//借书数量
int sum=5;//借书限制为5本
Book[] books={new Book(1,"至尊人生"),
new Book(2,"阴间商人"),
new Book(3,"斗破苍穹"),
new Book(4,"星际穿越"),
new Book(5,"盗梦空间"),
};
public void lent()throws NotFindThisBookException,InputErrorException{
try{
System.out.println("亲爱的同学欢迎您使用借书系统! 一份耕耘,份收获,愿你在书中所得能使你变得更好!");
System.out.println("请您输入命令:1.按照名称查找图书;2.按照序号查找图书");
Scanner in=new Scanner(System.in);
int select=in.nextInt();
if(!(select==1||select==2)){
System.out.println("输入不合法系统自动退出");
throw new InputErrorException("输入错误异常");
//System.exit(0);
}else if(select==1){
System.out.println("请输入图书书名");
String select1=in.next();
for(int i=0;i<books.length;i++){
if(select1.equals(books[i].name)){
books[i].show();
count=count+1;
sum=sum-count;
System.out.println("你已借书"+count+"本还能借:"+sum+"本");
break;
}else if(!(select1.equals(books[i].name))){
num++;
if(num==5){
throw new NotFindThisBookException("图书不存在异常");
}
}
}
}else {
System.out.println("请输入图书编号");
int select2=in.nextInt();
for(int j=0;j<books.length;j++){
if((select2<0||select2>books.length)){
System.out.println("输入不合法系统自动退出");
throw new InputErrorException("没有此编号的图书异常");
}else if(select2-1==j){
books[j].show();
count=count+1;
sum=sum-count;
System.out.println("你已借书"+count+"本还能借:"+sum+"本");
}
}
}
}catch (InputErrorException e){
//System.out.println("命令输入错误!请根据提示输入数字命令!");
e.printStackTrace();
}catch(NotFindThisBookException e){
// System.out.println("不好意思我们这里没有这本书!");
e.printStackTrace();
}catch (Exception e){
}
}
}
import pojo.BookLent;
import pojo.InputErrorException;
import pojo.NotFindThisBookException;
public class Test2 {
public static void main(String[]arg) throws NotFindThisBookException, InputErrorException {
BookLent bl=new BookLent();
bl.lent();
}
}
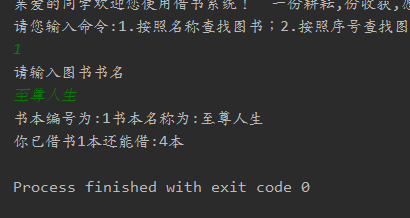
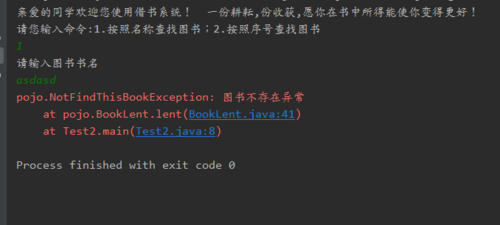