<?php
require_once 'vendor/autoload.php';
use Predis\Client;
function getKeyName($v)
{
return 'c_' . $v;
}
function getRedisClient()
{
return new Client([
'host' => '127.0.0.1',
'port' => 6379
]);
}
function writeLog($msg, $v)
{
$log = $msg . PHP_EOL;
$path = 'log/';
$filename = $path . $v . '.log';
if(!is_dir($path)){
$flag = mkdir($path,0777,true);
}
file_put_contents($filename, $log, FILE_APPEND);
}
function counter1()
{
$amountLimit = 100;
$key = getKeyName('counter1');
$redis = getRedisClient();
$incrAmount = 1;
if (!$redis->exists($key)) {
$redis->set($key, 95);
}
$currAmount = $redis->get($key);
if ($currAmount + $incrAmount > $amountLimit) {
writeLog("Bad luck", 'counter1');
return;
}
$redis->incrby($key, $incrAmount);
writeLog("Good luck", 'counter1');
}
function counter2()
{
$amountLimit = 100;
$key = getKeyName('counter2');
$redis = getRedisClient();
$incrAmount = 1;
$redis->setnx($key, 95);
if ($redis->incrby($key, $incrAmount) > $amountLimit) {
writeLog("Bad luck", 'counter2');
return;
}
writeLog("Good luck", 'counter2');
}
if (isset($_GET['v']) && $_GET['v'] == 2) {
counter2();
} else {
counter1();
}
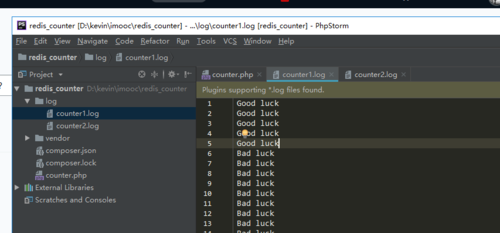