package com.example.admin.wuziqi;
import android.content.Context;
import android.graphics.Canvas;
import android.graphics.Paint;
import android.support.annotation.Nullable;
import android.util.AttributeSet;
import android.view.View;
public class wuziqipanel extends View {
private int panlWidth;//棋盘宽度
private float lineHeight;
private int Max_LINE = 10;
private Paint mPaint = new Paint();
public wuziqipanel(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
setBackgroundColor(0x44ff0000);
init();
}
private void init(){
mPaint.setColor(0x88000000);
mPaint.setAntiAlias(true);
mPaint.setDither(true);
mPaint.setStyle(Paint.Style.STROKE);
}
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
int widthSize = MeasureSpec.getSize(widthMeasureSpec);
int widthMode = MeasureSpec.getMode(widthMeasureSpec);
int heightSize = MeasureSpec.getSize(heightMeasureSpec);
int heightMode = MeasureSpec.getMode(heightMeasureSpec);
int width = Math.min(widthSize,heightSize);
if(widthMode == MeasureSpec.UNSPECIFIED){
width = heightSize;
}else if(heightMode == MeasureSpec.UNSPECIFIED){
width = widthSize;
}
setMeasuredDimension(width,width);
}
@Override
protected void onSizeChanged(int w, int h, int oldw, int oldh) {
super.onSizeChanged(w, h, oldw, oldh);
panlWidth = w;
lineHeight = panlWidth * 1.0f /Max_LINE;
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
drawBoard(canvas);
}
private void drawBoard(Canvas canvas){
int w =panlWidth;
float h = lineHeight;
for(int i =0 ;i<Max_LINE;i++){
int startX = (int)(h/2);
int endX = (int)(w-h/2);
int y =(int)((0.5 + i) * h);
canvas.drawLine(startX,y,endX,y,mPaint);
canvas.drawLine(y,startX,y,endX,mPaint);
}
}
}
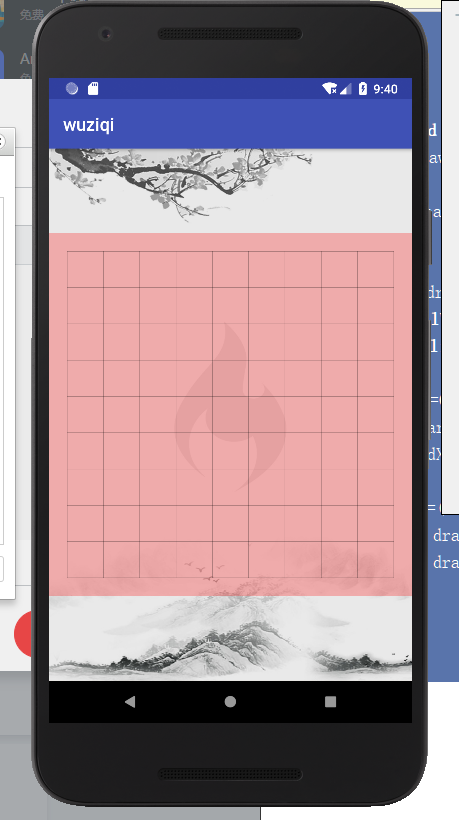