using System;using System.Collections.Generic;using System.Linq;using System.Text;using System.Threading.Tasks;
namespace PetShop
{
struct fish
{
int weight;
int size;
int type;
}
interface ICatchMice {
void CatchMice();
}
interface IClimbTree {
void climbTree();
}
abstract public class Pet {
public Pet(string name)
{
_name = name;
}
protected string _name;
public void PrintName()
{
Console.WriteLine("Pet's name is " + _name);
}
//public uint age; //public string food; abstract public void Speak();
virtual public void Move()
{
}
}
public class Dog:Pet {
static int Num;
static Dog()
{
Num = 0;
}
public Dog(string name):base(name)
{
++Num;
}
new public void PrintName()
{
Console.WriteLine("宠物的名字是 " + _name);
}
sealed override public void Speak()
{
Console.WriteLine(_name + " is speaking" + " wow");
}
override public void Move()
{
}
static public void ShowNum()
{
Console.WriteLine("Dog's number = " + Num);
}
}
public class Labrador : Dog {
public Labrador(string name):base(name)
{
}
}
public class Cat:Pet,ICatchMice,IClimbTree {
public Cat(string name):base(name)
{
}
override public void Speak()
{
Console.WriteLine(_name + " is speaking" + " meow");
}
public void CatchMice()
{
Console.WriteLine(" Catch mice");
}
public void ClimbTree()
{
Console.WriteLine(" Climb tree");
}
}
class Program {
static void Main(string[] args)
{
Pet[] pets = new Pet[] {new Dog("Jack"),new Cat("Tom")};
//Pet dog = new Dog(); //dog.PrintName(); //dog.Speak(); ////Pet cat = new Cat(); //cat.PrintName(); //cat.Speak(); for (int i = 0; i < pets.Length; ++i)
{
pets[i].Speak();
}
Cat c = new Cat("Tom2");
IClimbTree climb = (IClimbTree)c;
c.ClimbTree();
climb.ClimbTree();
ICatchMice catchM = (ICatchMice)c;
c.CatchMice();
catchM.CatchMice();
Dog.ShowNum();
}
}
}
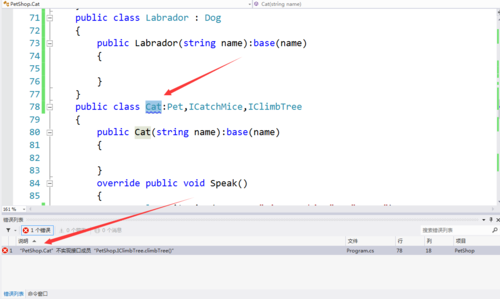