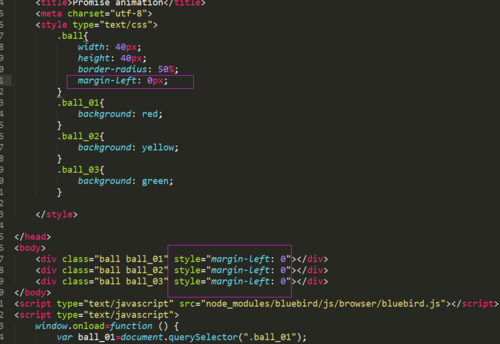
<!DOCTYPE html>
<html>
<head>
<title>Promise animation</title>
<meta charset="utf-8">
<style type="text/css">
.ball{
width: 40px;
height: 40px;
border-radius: 50%;
margin-left: 0px;
}
.ball_01{
background: red;
}
.ball_02{
background: yellow;
}
.ball_03{
background: green;
}
</style>
</head>
<body>
<div class="ball ball_01" style="margin-left: 0"></div>
<div class="ball ball_02" style="margin-left: 0"></div>
<div class="ball ball_03" style="margin-left: 0"></div>
</body>
<script type="text/javascript" src="node_modules/bluebird/js/browser/bluebird.js"></script>
<script type="text/javascript">
window.onload=function () {
var ball_01=document.querySelector(".ball_01");
var ball_02=document.querySelector(".ball_02");
var ball_03=document.querySelector(".ball_03");
function animate(ball,distance,callback) {
setTimeout(function(){
var marginLeft = parseInt(ball.style.marginLeft,10);
if(marginLeft === distance){
callback&&callback();
}else{
if(marginLeft < distance){
marginLeft++;
}else{
marginLeft--;
}
ball.style.marginLeft = marginLeft + "px";
animate(ball,distance,callback);
}
}, 13);
}
// animate(ball_01,100,function(){
// animate(ball_02,200,function(){
// animate(ball_03,300,function(){
// animate(ball_03,150,function(){
// animate(ball_02,150,function(){
// animate(ball_01,150,function(){
// //
// })
// })
// })
// })
// })
// })
var Promise = window.Promise;
function promiseAnmiate(ball,distance){
return new Promise(function(resolve,reject){
function _animate() {
setTimeout(function(){
var marginLeft = parseInt(ball.style.marginLeft,10);
if(marginLeft === distance){
resolve();
}else{
if(marginLeft<distance){
marginLeft++;
}else{
marginLeft--;
}
ball.style.marginLeft = marginLeft + "px";
_animate();
}
}, 13);
}
_animate();
});
}
promiseAnmiate(ball_01,100)
.then(function(){
return promiseAnmiate(ball_02,200)
})
.then(function(){
return promiseAnmiate(ball_03,300)
})
.then(function(){
return promiseAnmiate(ball_03,150)
})
.then(function(){
return promiseAnmiate(ball_02,150)
})
.then(function(){
return promiseAnmiate(ball_01,150)
})
}
</script>
</html>