你是否想过要将文本数据集中出现频率最高的词汇可视化?词云是一种非常好的方式,可以以既吸引人又富有信息量的视觉形式来展示文本数据。在本文中,我们将介绍使用React和react-d3-cloud库,来创建一个动态且可自定义的词云图的步骤。
我们要建造的东西我们将创建一个名为 WordCloudComponent
的组件,它会接收包含单词及其频率的数组作为输入并渲染一个漂亮排版的词云。具体来说,这个组件将具有以下功能:
- 根据词频动态调整字体大小
- 可自定义的字体粗细选项
- 限制显示的最大单词数量
- 自适应设计
让我们跳进去吧!
搭建项目环境安装所需的依赖:
npm install react-d3-cloud
``` (在终端中运行此命令可以安装react-d3-cloud)
点这里全屏,再点一下就退出全屏了.
## 构建词云组件:
我们先从创建主组件开始吧。创建一个名为 `WordCloudComponent.tsx` 的新文件(或 `.jsx`,如果你没有使用 TypeScript),并在此文件中添加以下代码:
import React, { forwardRef, useCallback, useMemo } from "react";
import WordCloud from "react-d3-cloud";
type Word = { text: string; value: number };
类型 Props = {
words: Word[];
};
const MAX_FONT_SIZE = 200;
const MIN_FONT_SIZE = 30;
const 最大字体粗细 = 700;
const 最小字体粗细 = 400;
const 最大单词数 = 150;
导出 const WordCloudComponent = forwardRef<HTMLDivElement, Props>(
({ words }, ref) => {
// 此处将实现组件逻辑
返回 (
<div ref={ref} style={{ width: "900px", height: "500px" }}>
{/* 此处将实现 WordCloud 组件 */}
</div>
);
}
);
WordCloudComponent.displayName = "WordCloud";
切换到全屏 退出全屏
这为我们组件设置了基本结构,我们来分解一下这里我们具体在做什么。
* 我们使用 `forwardRef` 让父组件能够访问词云的 DOM 节点。
* 我们定义了 `Word` 类型和 `Props` 类型以确保类型安全。
* 我们设置了几个常量,包括字体大小和粗细的范围,以及要显示的最大词汇数。
## 排序和筛选单词
接下来,我们添加代码逻辑,按频率排序单词并限制显示的数量。
const sortedWords = useMemo(
() => words.sort((a, b) => b.value - a.value).slice(0, MAX_WORDS),
[words]
);
定义一个名为sortedWords的常量,使用useMemo函数,对words数组中的元素按value属性降序排序,并截取前MAX_WORDS个元素。
点击这里进入全屏模式,点击这里退出全屏模式
我们利用 `useMemo` 来优化性能表现,仅当 `words` prop 发生变化时才进行重新计算。
## 字体尺寸和粗细
为了使我们的词云看起来更吸引人,我们将根据词频调整字体大小和粗细。下面在组件中添加这些功能。
const [minOccurences, maxOccurences] = useMemo(() => {
const min = Math.min(...sortedWords.map((w) => w.value));
const max = Math.max(...sortedWords.map((w) => w.value));
return [min, max];
}, [sortedWords]);
const calculateFontSize = useCallback(
(wordOccurrences: number) => {
const normalizedValue =
(wordOccurrences - minOccurences) / (maxOccurences - minOccurences);
const fontSize =
MIN_FONT_SIZE + normalizedValue * (MAX_FONT_SIZE - MIN_FONT_SIZE);
return Math.round(fontSize);
},
[maxOccurences, minOccurences]
);
const calculateFontWeight = useCallback(
(wordOccurrences: number) => {
const normalizedValue =
(wordOccurrences - minOccurences) / (maxOccurences - minOccurences);
const fontWeight =
MIN_FONT_WEIGHT +
normalizedValue * (MAX_FONT_WEIGHT - MIN_FONT_WEIGHT);
return Math.round(fontWeight);
},
[maxOccurences, minOccurences]
);
您可以全屏模式,退出全屏。
这些函数在我们定义的最小值和最大值之间根据线性尺度计算字体大小和粗细。
## 生成词云
现在,我们来使用 react-d3-cloud 库渲染词云。用如下所示的内容替换返回语句里的占位符:
<WordCloud
width={1800}
height={1000}
font={"Poppins"}
fontWeight={(word) => calculateFontWeight(word.value)}
data={sortedWords}
rotate={0}
padding={1}
fontSize={(word) => calculateFontSize(word.value)}
random={() => 0.5}
// 这个代码片段用于生成一个词云,其中字体、旋转角度、边距等参数都进行了设置。
/>
进入全屏 退出全屏
这样就配置好了WordCloud组件,采用了我们计算出的字体大小及粗细。
## 使用 WordCloudComponent 组件
要在你的 React 应用中使用这个组件,你可以这样用。
import { WordCloudComponent } from './WordCloudComponent';
function App() {
const words = [
{ text: 'React', value: 100 },
{ text: 'JavaScript', value: 80 },
{ text: 'TypeScript', value: 70 },
// ... 更多词
];
return (
<div className="App">
<h1>我的词云图</h1>
<WordCloudComponent words={words} />
</div>
);
}
全屏,退出全屏
## 数据分词处理准备
既然我们已经有了词云组件,让我们来谈谈如何准备数据吧。通常,你会从原始内容开始,并需要将其分词以获取每个词的频率。这里有一个简单的分词方法使用'natural'包。
import { WordTokenizer } from 'natural';
import stopwords from 'natural/lib/natural/util/stopwords';
const tokenizer = new WordTokenizer();
const stopWords = new Set(stopwords);
// 获取文本中的单词token,排除停用词和单字符
export const getTokens = (text: string): string[] => {
const lowerText = text.toLowerCase().replace(/[^\w\s]/g, "");
return tokenizer
.tokenize(lowerText)
.filter((token) => token.length > 1 && !stopWords.has(token));
};
全屏查看,取消全屏
这一功能做到了几个重要的作用:
1. 步骤1:将文本转换成小写
2. 步骤2:移除标点
3. 步骤3:将文本分割成单独的词
4. 步骤4:移除停用词(如“the”,“a”,“an”这类含义不重要的常用词)
5. 步骤5:过滤掉单个字符的词
您可以使用此功能来处理您的文本数据,在计算词频前。
const text = "这里输入您的长文本...";
const tokens = getTokens(text);
const wordCounts = tokens.reduce((acc, token) => {
acc[token] = (acc[token] || 0) + 1;
return acc;
}, {});
const words = Object.entries(wordCounts).map(([text, value]) => ({ text, value }));
// 词频统计
// 映射为
全屏模式 退出全屏
## 结尾
[\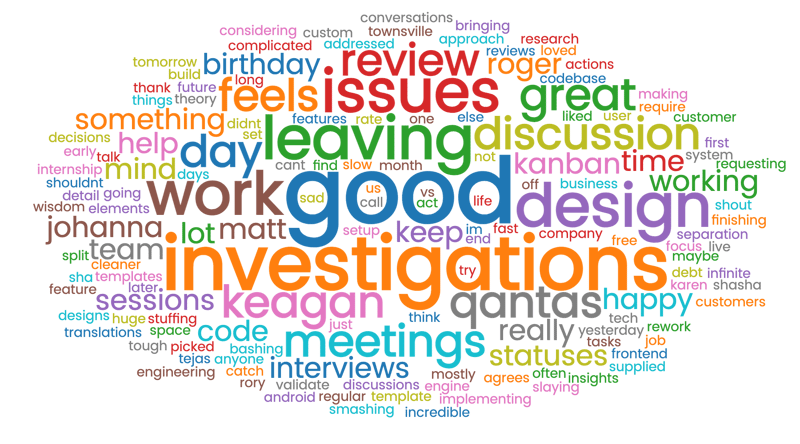](https://imgapi.imooc.com/6705e9ff09a7b4fe08000444.jpg)
使用 `react-d3-cloud` 创建词云是一种强大的可视化文本数据的方法。我们构建的 `WordCloudComponent` 灵活且可以轻松集成到更大的 React 应用程序中。通过根据词频自定义字体大小和粗细,我们创建了数据的视觉吸引人的表现。
记得,关键步骤如下:
1. 对你的文本数据进行分词和处理
2. 统计每个词出现的次数
3. 把词的数据传给词云组件
4. 看看美丽的词云渲染出来是什么样的!
顺便说一句,我也来推荐一下 🔌。如果你在敏捷开发团队工作,并且使用像计划扑克或回顾会议这样的在线工具,可以试试我免费提供的[Kollabe](https://kollabe.com/retrospectives)工具哦!
点击查看更多内容
为 TA 点赞
评论
共同学习,写下你的评论
评论加载中...
作者其他优质文章
正在加载中
感谢您的支持,我会继续努力的~
扫码打赏,你说多少就多少
赞赏金额会直接到老师账户
支付方式
打开微信扫一扫,即可进行扫码打赏哦